
"Block averaging" function?
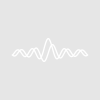
terracotta
I have actually written a simple function myself to do this, but it is rather slow and I was wondering if a function existed within Igor that would allow me to do this quickly.
I am attempting to "compress" a wave by taking the first 5 points of it, taking the average value of these points, and making this average one point in a new wave. I would then go to the next 5 points, take the average, and so on; the resulting wave is 5 times smaller than the initial. I could then make it 5 times smaller still by calling the function again using the output of the first call.
As I mentioned, it is very simple to program conceptually, but I was hoping someone might be able to point out a function that could do this and would be less computationally intensive (since I hear that many built-in Igor functions are quite optimized). I've looked through the Igor documentation and wasn't able to find anything, but it's entirely possible I missed something.
Thanks in advance.
ImageTransform compress myWave
A.G.
WaveMetrics, Inc.
February 17, 2010 at 11:21 am - Permalink
February 17, 2010 at 12:42 pm - Permalink
Then choose Analysis->Decimate.
To see what the is going on under the hood, choose Windows->Procedure Windows->Decimation.ipf. Basically, it creates a destination wave and then executes a wave assignment statement that calls the mean function.
February 17, 2010 at 01:20 pm - Permalink
Thanks again.
February 18, 2010 at 08:55 am - Permalink
June 27, 2018 at 02:55 am - Permalink