
Matrices are two dimensional named data objects (Igor supports up to four dimensions.) You can perform basic arithmetic operations on matrices using standard data assignment statements. For example:
Mat1= Mat2*Mat3 // element by element multiplication
You can perform matrix multiplication and matrix dot product with a natural syntax using MatrixOP. For example:
MatrixOp Mat1= Mat2 x Mat3 // matrix multiply
The following example computes element by element multiplication of matrix A with matrix B from which we subtract the inverse of matrix C times the diagonal matrix created from the array D:
MatrixOP res=A*B-Inv(D) x Diagonal(D)
MatrixOP supports array based operations such as Fourier Transforms, Chirp-Z transforms, convolutions and correlations. For example, you can compute circular or acausal convolutions using cascaded transforms and array multiplications as in the following lines:
MatrixOP/O circConvolution=IFFT(FFT(fx,0)*FFT(rect,0),0) MatrixOP/O acausalConvolution=IFFT(FFT(fx,0)*FFT(rect,0),4)
Obviously you can get the same results using the more compact syntax:
MatrixOP/O circConvolution2=Convolve(fx,rect,0) MatrixOP/O acausalConvolution2=Convolve(fx,rect,4)
The following is a list of functions supported under MatrixOP:
Numbers and Arithmetic | e, inf, Pi, nan, maxAB, mod. |
Trigonometric | acos, asin, atan, atan2, cos, hypot, phase, sin, sqrt, tan. |
Exponential | acosh, asinh, atanh, cosh, exp, ln, log, powC, powR, sinh, tanh. |
Complex | cmplx, conj, imag, magSqr, p2Rect, phase, powC, r2Polar, real. |
Rounding and Truncation | abs, ceil, clip, floor, mag, round. |
Conversion | cmplx, fp32, fp64, int8, int16, int32, uint8, uint16, uint32. |
Data Properties | numCols, numPoints, numRows, numType, waveChunks, waveLayers, wavePoints. |
Data Characterization | averageCols, crossCovar, chol, det, frobenius, integrate, intMatrix, maxCols, maxVal, mean, minVal, productCol, productCols, productDiagonal, productRows, sgn, sum, sumBeams, sumCols, sumRows, sumSqr, trace, varCols. |
Data Creation and Extraction | beam, catCols, catRows, col, colRepeat, rowRepeat, chunk, const, getDiag, identity, insertMat, inv, layer, rec, subRange, subWaveC, subWaveR, tridiag, waveIndexSet, waveMap, zeroMat. |
Data Transformation | diagonal, diagRC, normalize, normalizeCols, normalizeRows, redimension, replace, replaceNaNs, rotateChunks, rotateCols, rotateLayers, rotateRows, scale, scaleCols, setCol, setNaNs, setOffDiag, setRow, shiftVector, subtractMean, transposeVol. |
Time Domain | asyncCorrelation, convolve, correlate, limitProduct, syncCorrelation. |
Frequency Domain | chirpZ, chirpZf, fft, ifft. |
Matrix | backwardSub, chol, det, diagonal, diagRC, forwardSub, frobenius, getDiag, identity, inv, setOffDiag, tensorProduct, trace. |
Special Functions | erf, erfc, inverseErf, inverseErfc. |
Logical | equal, greater, within. |
Bitwise | bitAnd, bitOr, bitShift, bitXOR, bitNot. |
Linear Algebra Operations
Igor Pro® includes a group of operations and functions for linear algebra applications. For convenience their names start with the word "Matrix". Additional matrix math features are grouped under image operations.
Igor uses the industry-tested LAPACK library for many linear algebra operations. Following is a list of the more common MatrixXXX operations:
MatrixDet | returns the determinant of a matrix. |
MatrixEigenV | an eigenvalue/eigenvector solver for general and for symmetric matrices. |
MatrixGaussJ | a Gauss-Jordan matrix inverter for solution of linear equations. Gauss-Jordan may be one of the elementary methods that they teach us in linear algegra but it is not the one that we would recommend for numerical stability. Linear equations should be solved using MatrixLinearSolve, MatrixLLS or MatrixLUD. |
MatrixGLM | solves the general Gauss-Markov Linear Model problem. |
MatrixInverse | computes the inverse or the pseudo-inverse of a square matrix. |
MatrixLinearSolve | MatrixLinearSolve solves the linear system matrixA*X=matrixB where matrixA is an N-by-N matrix and matrixB is an N-by-M matrix of the same data type. |
MatrixLinearSolveTD | MatrixLinearSolve solves the linear system matrixA*X=matrixB where matrixA is a tri-diagonal matrix and matrixB is an N-by-M matrix of the same data type. |
MatrixLLS | MatrixLLS solves overdetermined or underdetermined linear systems involving MxN matrixA, using either QR/LQ or singular value decompositions. Supported types are real or complex single precision and double precision numbers. |
MatrixLUD | perform LU decomposition on a square matrix resulting in a pair of lower (L) and upper (U) triangular matrices. |
MatrixMultiply | performs matrix multiplication for up to 10 matrices. You can also use MatrixOP for more convenient notation. |
MatrixSchur | computes the Schur decomposition of a square matrix. |
MatrixSVD | performs singular value decomposition using LAPACK routines. |
MatrixTranspose | swaps the rows and columns of a matrix in place. |
MatrixDet | computes the determinant of a real square matrix. |
MatrixRank | computes the rank of a matrix subject to a user specified condition number. |
MatrixTrace | computes the trace of a square matrix. |
Other Matrix Operations:
MatrixConvolve: convolves a small 2D kernel with a larger destination matrix usually for image processing applications.
MatrixFilter: contains a variety of options for filtering matrix data usually for image processing. Some of the built-in filters include averaging, edge finding, Gaussian blur, gradients, median, sharpen and more.
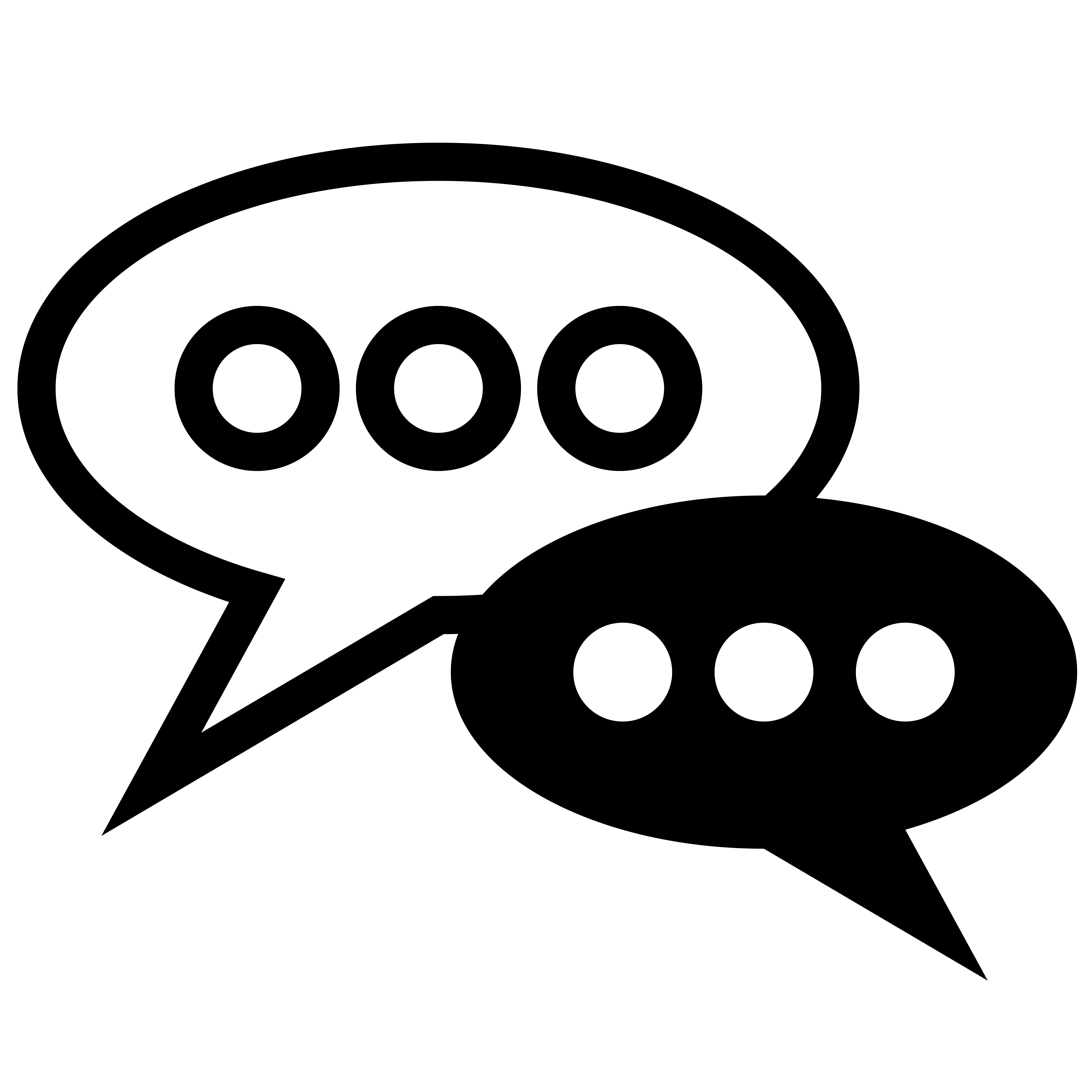
Forum
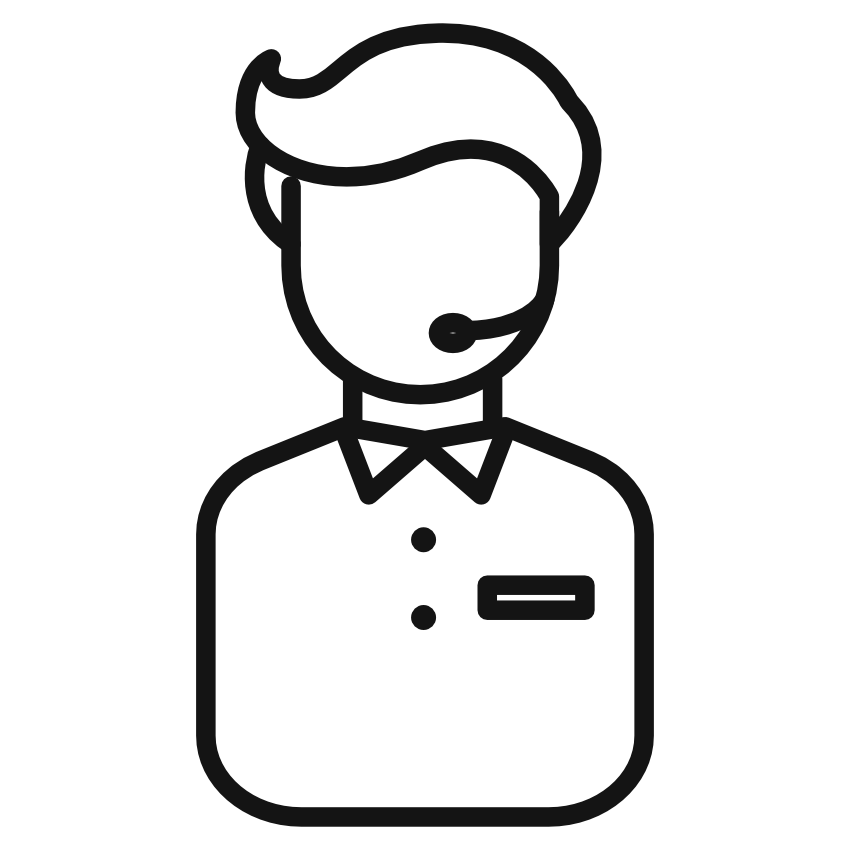
Support
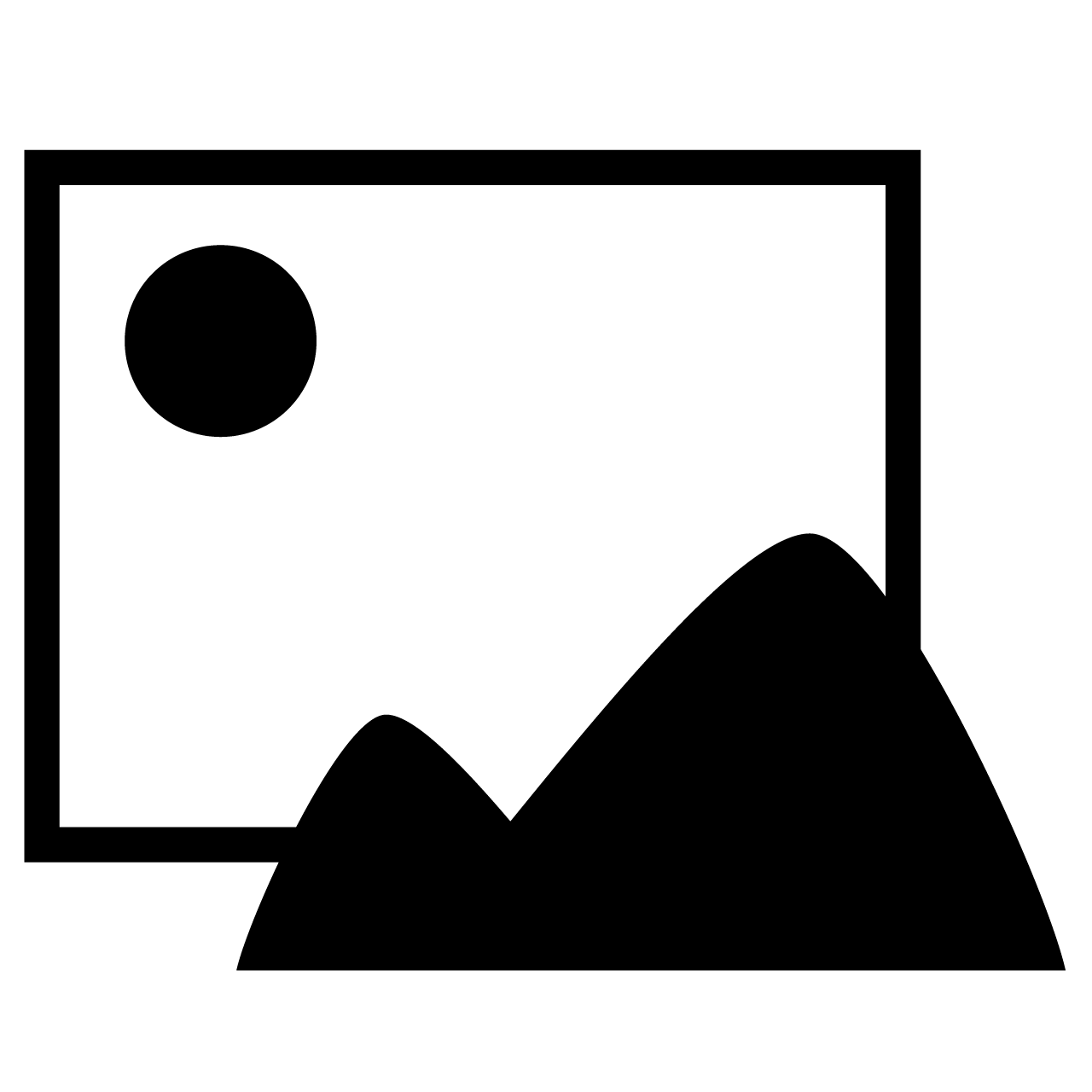
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More