
call Device Application or use VDT2?
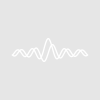
kevin.dockery
I am making my first efforts at using Igor to carry out I/O on a Com port via RS232, using ascii commands. The instrument is an electrometer (Keithley 6514, VISA communication protocol). In brief, there is a supplied communicator which can at least control the instrument for simple commands (read?, meas?, *rst). However, the supplied Communicator does not have the ability to loop, and in any case the data will ultimately end up in Igor. So I was wondering (1) would anyone know a way to simply call the Communicator application from an Igor Function to get instrument output? and/or (2) perhaps someone would
have some code to help as an introduction to VDT2 (of which I am a complete novice).
Best Regards,
Kevin
A better approach would be to use VDT2 to send the commands to the device yourself. Start by reading the documentation for the device to understand what commands it requires. Make note of whether it requires CR, LF or CRLF to terminate a command.
Then start reading the "VDT2 Help" file (in "Igor Pro Folder\More Extensions\Data Acquisition").
All you need to do is to set the port up:
and then send a command:
May 20, 2010 at 09:45 pm - Permalink
First, you can try this:
1) set up the 6514 for RS 232 communication (see manual chapter 12 'Remote Operation')
2) load the VDT2 XOP in Igor
3) go to Misc -> VDT2 -> VDT2 settings and configure the com port to match you requirements
4) open the VDT2 Window from the same menu and communicate with the instrument by actually typing in the commands (make sure the 'terminal port' is set to the right com port)
With this you can just try out, how to get the results you want and set up the device. After this you can build your procedure with the commands. For example:
Expand this as you wish... (see chapter 15 'SCPI Signal Oriented Measurement Commands' for all commands). If you are not using the default settings for the com port, I suggest you add a line at the top to configure this every time (see post from hrodstein).
To read the data then use something like this:
RS232 has the problem that a connection loss or some other errors in the device are not detected. Use timeouts and some error handling for this to prevent 'hanging' of you procedure (use ctrl-break to abort the command manually if this occurs).
June 2, 2010 at 08:43 am - Permalink