
"Can't use $ in this way in a function"
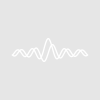
neogin
I'm making a function to normalize my graph data, its goal is to take each trace of an active graph and divide by its max value in order to normalize it to 1.
So here it is :
<br /> <br /> Function/S NormaliserGraph()<br /> string NomDesWaves<br /> variable NbrDeWave,MaxWave,pas<br /> string NomWave,NowWaveNorm<br /> <br /> NomDesWaves=TraceNameList("", ";", 1 )<br /> NbrDeWave=ItemsInList(NomDesWaves)<br /> print NomDesWaves<br /> print NbrDeWave<br /> <br /> for(pas=0;pas<NbrDeWave;pas=pas+1) <br /> <br /> NomWave=StringFromList(pas,NomDesWaves,";") <br /> NomWave=StringFromList(1,NomWave,"'") <br /> print NomWave<br /> MaxWave=WaveMax($NomWave)<br /> print MaxWave<br /> <br /> $NomWave=$NomWave/MaxWave<br /> <br /> endfor <br /> <br /> <br /> return("1")<br /> End<br />
Of couse I got the "Can't use $ in this way in a function" error for the line :
<br /> $NomWave=$NomWave/MaxWave<br />
My question is how can I do this division as I don't knonw in advance the name of my wave ?
thank you for your input,
cheers
Gautier
January 5, 2012 at 05:51 am - Permalink
if there is people interested here's the final code. I added a part to save the wave before normalization and an if part to check if the wave has already been normalized to prevent the overwrite of the saved wave.
January 5, 2012 at 07:14 am - Permalink
Hi guys!
When you use the <igor> and </igor> tags instead of the <ccode> and </ccode> tags it looks like actual Igor code (plus using Adjust Indentation from the Edit menu helps a little, too):
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
January 5, 2012 at 11:49 am - Permalink
I have an instance of this error that I don't understand.
The error is on "Speed1 = $Speed1Str". I guess this should involve some problem with runtime lookup of globals without declaring them correctly, but LFWaveString is local and it seems like substring Speed1Str should also be local, so there should be no problem. Changing String declarations to SVAR as I tried above doesn't help.
April 13, 2023 at 07:44 am - Permalink
Speed1 and Speed2 are numeric variables, not references to anything global, so the $ operator isn't what you need here. Instead, you need to do something to Speed1Str and Speed2Str to get a number from them. The easy way is str2num():
There are other variations on this to handle some corner cases, but str2num() probably covers 99% of cases.
Let us know if that's not what you intended.
April 13, 2023 at 09:26 am - Permalink
Thank you, that immediately fixed it. I've just reviewed the string substitution help file and I think I understand better now.
April 13, 2023 at 09:30 am - Permalink