
Creating N number of waves
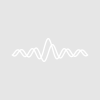
isk8
Fri, 05/11/2012 - 02:39 pm
I have waves that have a multiple of 1024 points (1024 x N points).
I'm trying to decompose these waves into N number of waves, each with 1024 points, whose names are wave_N.
I know how to decompose waves having 1024 points each, but I'm not sure how to make N number of waves depending on the number of points I have in the starting wave (1024 x N points).
Does anyone know how you would make N number of waves in this case?
Thank you.
// Example:
// Make /O /N=10 testInputWave = p
// Edit testInputWave
// DecomposeNumeric1DWave(testInputWave, 3, "output")
// AppendToTable output0, output1, output2, output3
Function DecomposeNumeric1DWave(w, pointsPerOutputWave, baseName)
Wave w // A 1D wave
Variable pointsPerOutputWave // e.g., 1024
String baseName // e.g., "wave" to get wave0, wave1, . . .
Variable numInputPoints = numpnts(w)
if (numInputPoints == 0)
return 0
endif
Variable numOutputWaves = ceil(numInputPoints / pointsPerOutputWave)
Variable startPoint=0, endPoint
Variable i
for(i=0; i<numOutputWaves; i+=1)
String outputName
sprintf outputName, "%s%d", baseName, i // wave0, wave1
endPoint = startPoint + pointsPerOutputWave - 1
endPoint = min(endPoint, numInputPoints-1)
Duplicate /O /R=[startPoint, endPoint] w, $outputName
startPoint = endPoint + 1
endfor
return 0
End
May 11, 2012 at 03:45 pm - Permalink