
duplicate using the s_wavenames
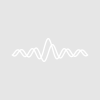
Simon87
Mon, 12/05/2011 - 05:54 am
I'm writting a function in which I'd like to automatically duplicate the wave from a textfile. The problem is that the wave name is not always the same (it depends on some parameters used for the data acquisition) and it would be preferable for me to keep the original wavename. But I also need a unique name so that I don't have to change the wavename each time in the body function. So, I would like to duplicate the original wave called "dataX" (X varying from a textfile to another) to a wave called wave0. But the name "dataX" is not always the same, and I don't want to change it manually each time. So I think I have to use the s_wavename in the function, to automatically search the wavename and duplicate the wave dataX to wave0, but I don't know how to do it.
ps : I download the data manually, not using the loadwave function.
Thanks,
Simon
For example:
// Your actual question:
Duplicate sourceWave, wave0
// What you probably want:
Variable counter = 0
String destWaveNameString = "wave" + num2istr(counter)
Duplicate sourceWave, $(destWaveNameString)
For more information, execute the following on Igor's command line:
December 5, 2011 at 07:21 am - Permalink
If s_wavename only contains the name of one wave then you can refer to it in the duplicate satement as
Duplicate $s_wavename, wave0
A quick glance at the help file for the duplicate operation indicates that you will then need to create a reference to wave0 as in
Wave wave0
Then you can use wave0 in your code.
If s_wavename contains a list of wave names then you will have to use StringFromList to pull out individual wave names.
December 5, 2011 at 07:23 am - Permalink
Simon
December 5, 2011 at 08:41 am - Permalink
Please post your code. The $ operator works in functions as well.
December 5, 2011 at 08:47 am - Permalink
string w0
w0=stringfromlist(0, s_wavenames)
duplicate $w0, super
edit super
print w0
End
-> It works
Function dodo()
string w0, s_wavenames
w0=stringfromlist(0, s_wavenames)
duplicate $w0, super
edit super
print w0
End
-> It doesn't work (error : attempt to use a null string variable). I can't understand why.
December 5, 2011 at 09:17 am - Permalink
Is that really the entire code, or an extract? Because you didn't set S_wavenames to anything in either the macro or function.
In the macro you didn't define S_wavenames, so the macro will find the global string S_wavenames.
The function won't do that unless you use:
string w0
SVAR swn= root:s_wavenames
w0=stringfromlist(0, swn)
duplicate $w0, super
edit super
print w0
End
You can see a description of the differences between writing macros and writing functions by entering this in Igor's command line:
--Jim Prouty
Software Engineer, WaveMetrics, Inc.
December 5, 2011 at 11:09 am - Permalink
LoadWave . . . // Creates and declares S_waveNames
String nameOfLoadedWave = StringFromList(0, S_waveNames) // Get name of loaded wave
Wave loadedWave = $nameOfLoadedWave // Create a wave reference
Duplicate/O loadedWave, copyOfLoadedWave
End
As Jim said, if you run LoadWave from the command line, it will create a global string variable named S_waveNames. In this case you can reference it using SVAR. However, functions that depend on globals are hard to debug and maintain so this approach should be avoided.
December 5, 2011 at 02:24 pm - Permalink