
Echoing command to wave note
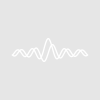
ouoeda
Hi all,
I made a function that takes several optional parameters and creates a wave according to those parameters.
To keep track of the parameters that were used to create the wave, I would like to copy the whole command to the note of the wave.
The most simple way that I can imagine of achieving this is to copy the command from the history window, but I cannot find a way to access text in the history from inside a function.
I would appreciate if someone can tell me a method of echoing the command to the note of a wave.
Thank you,
Hiroyuki
There was a thread somewhere about echoing commands into a notebook and the history, which could give you some ideas. But here is how I would do it the easy and quick way (assuming your commands are reasonably simple):
1) Don't execute the commands directly, but line them up in a string, i.e.,
2) Then run Execute over each command in the list to create your wave
3) Finally, save execStr into the wave notes: Note/K mywave, execStr
You may want to add some formatting before writing the note. But this approach makes sure that the wave notes and the actually executed commands are (have to be) the same. You could also try to keep track of the commands yourself in a string, but there will be mistakes for sure.
As an alternative, you may want to just write the used parameters in a list (ideally in a format which can be easily parsed by StringByKey() and NumberByKey() for smooth readout), which is more user-friendly but involves setting um a dedicated string list in your function. I usually go this extra mile because I want to see the parameters directly instead of skimming through a list of commands.
June 14, 2022 at 02:27 am - Permalink
Chozo gives good advice. However, history window text can be copied using the functions "CaptureHistoryStart" and "CaptureHistory". Here's a simple example:
June 14, 2022 at 06:07 am - Permalink
If the parameters that your function takes become basic properties of the wave then I recommend that you not try to store them separately in the wave note because you can just read them out of the wave itself. For example, if your function takes parameters for dimension sizing (number of rows, cols, etc), wave scaling, or dimension labels, then you don't need to store those parameters separately since you can use functions such as DimSize, DimDelta, WaveUnits, GetDimLabel, etc. to read the information out of the wave.
However if your parameter describes some sort of analysis, for example how many filter passes to use on data that is stored in the wave, you may want to store that information in the wave's note.
Since you already have a function that the user must execute to create your wave, you shouldn't need to copy the command from the history window. Your function knows the values of its parameters so it should be able to recreate the command that was called (more or less, at least).
It's not possible for a function to read from the history window. The closest thing we have is:
DisplayHelpTopic "CaptureHistory"
It's possible that you could use this feature to get what you want, but I think it would ultimately be more straightforward for you to skip trying to capture the exact command that was executed and instead just store the values of the parameters of your function that generates the wave in the first place. Here is a very simple example:
In this case you can't look at the wave's data and directly extract the values of the a, b, and c parameters, so perhaps it makes sense to store them in the wave note. However if you had used one of the parameters to determine the number of rows in the wave, you wouldn't need to store it since you could simply call DimSize(ddd, 0) to determine the number of rows.
If this doesn't answer your question I think it would be useful if you could give some more detail on what you are trying to do.
June 14, 2022 at 06:20 am - Permalink