
Find npnts in text wave
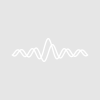
Thomas.Dane
Fri, 01/27/2012 - 03:51 am
Function UpdateDataWaveList(matOutput)
Wave matOutput
String exptDF = GetDataFolder(1)
Wave/T DataWaveList = $(exptDF+":All_data:DataWaveList")
If ( waveDims(DataWaveList) == 0 )
InsertPoints 0,1, DataWaveList
DataWaveList[0]=NameOfWave(matOutput)
else
WaveStats/Q DataWaveList
InsertPoints (V_endRow),1, DataWaveList
DataWaveList[V_npnts]=NameOfWave(matOutput)
endif
End
Wave matOutput
String exptDF = GetDataFolder(1)
Wave/T DataWaveList = $(exptDF+":All_data:DataWaveList")
If ( waveDims(DataWaveList) == 0 )
InsertPoints 0,1, DataWaveList
DataWaveList[0]=NameOfWave(matOutput)
else
WaveStats/Q DataWaveList
InsertPoints (V_endRow),1, DataWaveList
DataWaveList[V_npnts]=NameOfWave(matOutput)
endif
End
But wavestats doesn't work on textwaves. Perhaps a feature for IP7? Obviously numerical values like V_max won't work but it could still return V_npnts etc.
Many thanks,
Tom
Wave matOutput
String exptDF = GetDataFolder(1)
Wave/T DataWaveList = $(exptDF+":All_data:DataWaveList")
If ( waveDims(DataWaveList) == 0 )
InsertPoints 0,1, DataWaveList
DataWaveList[0]=NameOfWave(matOutput)
else
InsertPoints (numpnts(DataWaveList)),1, DataWaveList
DataWaveList[numpnts(DataWaveList)]=NameOfWave(matOutput)
endif
End
January 27, 2012 at 04:20 am - Permalink
You say that DataWaveList is predefined, but what are the dimension, points etc. at time of execution? Can I assume that the wave has one dimension and one empty row entry at start?
First, I'm not sure if your if-else statement is useful at all since I don't see any statement modifying the wave dimension (could be somewhere else though). This way, the function fills the name into the first row entry if the wave is one-dimensional and into the last row entry if the wave is multidimensional. But then you should be careful with the use of numpnts.
If you only want a one-dimensional list of names, I'd suggest something like (this is not tested though. Counting could be off):
Wave matOutput
String exptDF = GetDataFolder(1)
Wave/T DataWaveList = $(exptDF+":All_data:DataWaveList")
Variable NewSize = DimSize(DataWaveList, 0) // get the number of row points (same as numpnts for 1D)
NewSize = strlen(DataWaveList[NewSize-1]) == 0? NewSize-1 : NewSize // decide if last element has something stored
// no need to extend wave if not (especially for a fresh wave)
Redimension/N=(NewSize+1) DataWaveList // extend the wave
DataWaveList[NewSize] = NameOfWave(matOutput) // write the name
End
January 27, 2012 at 06:49 am - Permalink
A few other notes:
1. Instead of storing the names in a text wave, you might find using a list contained in a string to be easier. It depends on what you are doing with the text wave later on. If you want to use a string list, look at the AddListItem, StringFromList, and ItemsInList functions.
2. Instead of using NameOfWave, I suggest you use
GetWavesDataFolder(matOutput, 2)
. This will give you the full path to the wave, including its name. You may not be using data folders much now, but if you decide to do so in the future you won't have to change this code to support the waves being in different data folders.3. Depending on what you are doing with the text wave containing wave names, you might be better off using a wave that contains wave references to the loaded waves. Execute
DisplayHelpTopic "Wave Reference Waves"
on the command line for more info.January 27, 2012 at 07:09 am - Permalink
Using a string list would be a lot simpler but I need a text wave as it will be used for a list box in a data browsing panel.
The package uses a hierachical data folder system to store all waves but I don't think I will need the full path as I define the path the text wave on creation of the list box.
I was not aware of the syntax:
NewSize = strlen(DataWaveList[NewSize-1]) == 0? NewSize-1 : NewSize
but I like this method and will try and implement that.
I'll look into the wave reference waves route too.
January 27, 2012 at 07:40 am - Permalink
I may be wrong, but from this I get the strong feeling that you are mixing up dimensions and size of the wave. A wave can have up to four dimensions and many items in each dimension. Please be careful what you do with WaveDims(...) != DimSize(...).
Also, you haven't written it in your post, but please consider that calling make/T without the N flag results not in zero rows but 128(!) empty entries as a default value.
January 27, 2012 at 09:50 am - Permalink