
Finding point number for a certain wave value
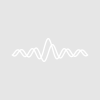
smavadia
Sun, 11/15/2009 - 09:25 am
I'm quite new to igor so sorry if this is an elementary question. I have a wave with a minimum and I want to find the difference between that point and the value of the wave a certain number of points away. My code looks something like:
function findtrapdepth ()
variable trapdepth, minxpoint, waveminimum
waveminimum = wavemin(totalpotential)
print waveminimum
minxpoint = x2pnt(totalpotential,waveminimum)
print minxpoint
End
I would have thought it had something to do with the x2pnt function but that just returns "0" for whatever value of my variable waveminimum.
After that if I want to find the value of a wave at a certain point do I just write:
totalpotential[pointnumber]
Any help appreciated,
Sandeep
A few questions first.
1) Is totalpotential the wave that has the minimum?
2) Is totalpotential a scaled wave rather than an unscaled wave?
3) Are you trying to find the value of totalpotential at point N away from its minimum rather than at a distance X (by its scaling) away?
If the answers to all of these are YES, then try this ...
wave ww
variable N
variable wmin, minpnt, vatN
string theValues
WaveStats/Q/M=1
wmin = V_min
minpnt = x2pnt(ww,V_minloc)
vatN = ww[minpnt+N]
sprintf theValues, "Minimum %g at point %d and value %g at point %d", wmin, minpnt, vatN, (minpnt+N)
return theValues
end
At the command line, type ValueAtPointNFromMin(totalpotential,3) to get a printout of what you are asking.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
November 15, 2009 at 05:40 pm - Permalink
thanks for your help, I still have a few more questions but first answering yours:
1) totalpotential is the wave with the minimum
2) I am using an unscaled wave, it would be nice to use a scaled wave in the future but it's not a problem at the moment.
3) N points away is fine I know what the conversion is.
Having a look at your code I guess the important thing is the V-minloc output of the wavestats function. As for x2pnt it seems to give out the same value the its second argument, no matter what the input wave is e.g.
x2pnt (anywave, 12) gives the value 12.
I had a go at this problem using a loop:
for (i=0; i<197; i+=1)
if (totalpotential[i] == wavemin(totalpotential))
minxpoint = i
endif
endfor
But this is quite a limited approach, it would be nice to do what I thought x2pnt was meant to do which was find the point number closest the x value that you input.
So the question is does x2pnt do anything?
Thanks
Sandeep
November 17, 2009 at 02:48 am - Permalink
That is because you are using an unscaled wave. For unscaled waves, the point number and the x-value at that point are the same. Therefore, x2pnt(anywave, ANYVALUE) will always return ANYVALUE as its response.
Based on your new code, all you need to do is the following ...
wave wy
variable wmin, minloc
string theValues
WaveStats/Q/M=1 wy
wmin = V_min
// this only works for UNSCALED waves
minloc = V_minloc
sprintf theValues, "Minimum %g at point or x value %d", wmin, minloc
return theValues
end
To get a better understand of x2pnt for scaled versus unscaled waves, try the functions below. First run MakeThem(), then try various inputs to Testx2pnt().
make/O/N=100 wscaled, wunscaled
SetScale/P x -50,0.5, wscaled
display/N=Testx2Pnt wscaled, wunscaled
ModifyGraph rgb(wscaled)=(1,3,39321),rgb(wunscaled)=(52428,1,1)
return 0
end
Function Testx2pnt(Xv,Np)
variable Xv,Np
string theValues
wave wscaled
wave wunscaled
wunscaled = x^Np
wscaled = x^Np
sprintf theValues, "Unscaled: (%g, %g) at point %g", Xv, wunscaled[x2pnt(wunscaled,Xv)], x2pnt(wunscaled,Xv)
sprintf theValues, "%s; Scaled: (%g, %g) at point %g", theValues, Xv, wscaled[x2pnt(wunscaled,Xv)], x2pnt(wscaled,Xv)
print theValues
return 0
end
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
November 17, 2009 at 08:05 am - Permalink
This all makes alot more sense now, thanks loads.
Sandeep
November 18, 2009 at 11:46 am - Permalink