
Graphing Problems
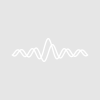
Cheryl
Mon, 02/15/2010 - 07:31 pm
#pragma rtGlobals=1 // Use modern global access method.
Function MyBergFunc(w, x) : FitFunc
Wave w
Variable x
//CurveFitDialog/
//CurveFitDialog/ Independent Variables 1
//CurveFitDialog/ x
//CurveFitDialog/ Coefficients 4
//CurveFitDialog/ w[0] = Xp
//CurveFitDialog/ w[1] = b
//CurveFitDialog/ w[2] = wp
//CurveFitDialog/ w[3] = Q_val
Variable Xp = w[0]
Variable b = w[1]
Variable wp = w[2]
Variable Q_val = w[3]
if ( x>10^-6)
return Xp/ (x*(1-b+(b/1+b)*(b*((wp*(Q_val^2))/x)+(x/(wp*(Q_val^2)))^b)))
elseif ( x<-10^-6)
return abs(Xp/ (x*(1-b+(b/1+b)*(b*((wp*(Q_val^2))/x)+(x/(wp*(Q_val^2)))^b))))
else
return 0
endif
end
I am having problems for when x is negative, it plots no values at all using the function grapher. Can anyone see why this is?
Also, I would like to know how to write a code to graph the function in a new window. Can anyone help me with how to do this?
Thank you very much for any help offered, I am new at using Igor and I am a little confused.
Could you perhaps provide further information about the data range you are fitting and the likely values for w[0]-w[3] that are expected.
As a note, you can avoid the if/elseif/else portion by recoding the function as such ...
Variable b = w[1]
Variable wp = w[2]
Variable Q_val = w[3]
variable nupper = 10^-6, nlower = -10^-6
variable rtrn = 0
rtrn = (Xp/ (x*(1-b+(b/1+b)*(b*((wp*(Q_val^2))/x)+(x/(wp*(Q_val^2)))^b))))*((x < nlower)||(x > nupper))
return (rtrn)
The portion at the end is a logical test that will notch the return (rtrn) as zero between the lower and upper bounds. The upside of this change is, it is faster to execute during fitting. The downside of this change is, you will no longer be able to edit the fit function in the curve fit dialog (you will have to edit it directly in the macro procedure window).
I have attached a BergFuncTest.pxp file to illustrate.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
February 16, 2010 at 08:37 am - Permalink
The abs I was putting in becuase the function was not plotting for the negative x values. The code I have now is:
Function MyBergFunc(w, x) : FitFunc
Wave w
Variable x
//CurveFitDialog/
//CurveFitDialog/ Independent Variables 1
//CurveFitDialog/ x
//CurveFitDialog/ Coefficients 4
//CurveFitDialog/ w[0] = Xp
//CurveFitDialog/ w[1] = b
//CurveFitDialog/ w[2] = wp
//CurveFitDialog/ w[3] = Q_val
Variable Xp = w[0]
Variable b = w[1]
Variable wp = w[2]
Variable Q_val = w[3]
if ( x>10^-6)
return Xp/ (x*(1-b+(b/(1+b))*(b*((wp*(Q_val^2))/x)+(x/(wp*(Q_val^2)))^b)))
elseif ( x<-10^-6)
Variable x2=-x
return (Xp/ (x2*(1-b+(b/(1+b))*(b*((wp*(Q_val^2))/x2)+(x2/(wp*(Q_val^2)))^b))))
else
return 0
endif
end
So that is why I was using the if/elseif/else command, so it would plot for negative x (negative x is just a mirror image of positive x) and for small values of x so I could have it plot zero instead of doing the calculation.
I will look at how you have done it, I really like the logical test. In the end I would like to get the y values for this function for when x =-100 to 100 with a step size of 0.02, fourier transform these into the time domain and then fit a stretched exponential. I think that I should be able to do that in Igor.
Thank you again for your help.
February 16, 2010 at 02:51 pm - Permalink
February 16, 2010 at 03:32 pm - Permalink