
How do I return multiple values in a function?
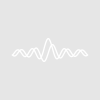
benjamin.groff
I am writing a function and would like to return several numerical values. I know that the return statement can only return one value, but when I make a fit of a line, this defines a bunch of variables (slope, y-int, r^2, stdev, etc.) -- does anybody know how the CurveFit() function creates all these variables if I'm only able to return one value in my user-defined functions?
If you are using Igor 8, you *can* return multiple values from a function. See the help: DisplayHelpTopic "Multiple Return Syntax"
In fact, CurveFit is not a function, it is an operation. User-defined procedures cannot create a user-defined operation.
The CurveFit operation creates a number of waves and variables that contain all that output info. You might also want to use a wave to return the info from your fits. You could use dimension labels to make it clear what info is in each slot of the wave. See this: DisplayHelpTopic "Dimension Labels"
February 6, 2020 at 03:55 pm - Permalink
Regarding the question in the title, here are some ideas:
You could use pass-by references, which modify the input variables you give the function. See Here:
DisplayHelpTopic "Pass-By-Reference"
However, this should be used with care, as it is not obvious how the variables have changed from the calling function, which may easily introduce errors.
You could also give a wave, which holds all your parameters, to your function as input. Any modifications to the wave will come out after the sub-function has ended. The problem here is to keep track of all the positions of this wave and which parameter is which.
You could encode multiple parameters in a string(-list) and just return the string at the end. You have to parse out all parameters later in the calling function, though. Also, depending on how you handle this, the conversion may destroy the precision of your values.
February 7, 2020 at 02:20 am - Permalink
Depending also on how far you want to carry the values, you can also use structures. For example, when I need to get multiple values from a panel input, I create a structure to store the values and a function to read the panel, fill the structure, and pass back the multiple values to the worker function.
February 7, 2020 at 08:11 am - Permalink
The multiple return syntax is great, because I'm calling this function in another function. Thank you!
February 7, 2020 at 09:47 am - Permalink
Hi, why does this not work on Macros or directly in the command line?
For example when I have:
And then type in the command line:
It says "syntax error".
February 24, 2023 at 03:33 am - Permalink
You need to define the variables as globals to run your function from the command line.
February 24, 2023 at 12:18 pm - Permalink
On the command line, all variables are global.
The multiple-return syntax is compiled to pass-by-reference function input parameters. Pass-by-reference is another thing that only works in compiled code, not in interpreted code.
February 24, 2023 at 01:35 pm - Permalink