
I'm newbie, please help me! (calculation problem in macro)
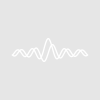
akihisa.y
I have been using Igor to make good looking graph for years,
recently I decided to try to using procedure for automation even if newbie I am at
computer programming.
Then I tried to make easy prime number searching program for self learning.
But the result show some unexpected numbers, like 27, 35, but almost correct.
I can't understand why the program skip the step like (27/3), (27/9), (35/5)...
Please give me an advice.
Best regards.
procedure
-------------------------------------------------------------------------------------------------------------------------
#pragma rtGlobals=1 // Use modern global access method.
macro test()
pauseupdate
silent 1
killwaves /a
make /N = 100 stack1
variable v1
variable v2
variable count1
variable timeron
variable timeroff
timeron = startmstimer
v1 = 2
v2 = 2
count1 = 0
do
if (v1 == 100)
print "end"
break
else
if ( mod(v1, v2) == 0)
if (v1/v2 == 1)
stack1[count1] = v1
count1 = count1 + 1
v1 = v1 + 1
v2 = 2
else
v1 = v1 + 1
endif
else
v2 = v2 + 1
endif
endif
while(1)
timeroff=stopmstimer(timeron)
print "Calculation time is...."
print timeroff / 1000
print "ms"
print stack1
endmacro
To learn how to use the Igor debugger, execute this:
Also, with rare exceptions you should write functions rather than macros. Execute this:
If you have not already done it, read chapters IV-1 through IV-3 of the Igor PDF manual. This will give you an introduction to Igor programming.
August 28, 2011 at 07:01 pm - Permalink
The problem is actually with your algorithm. If one number is not a prime, then testing for the next number does not start over at v2=2.
I figured that out using Igor's symbolic debugger, stepping through your code one line at a time, and watching how the values of the variables change during execution. To enable the debugger, right-click in any procedure window and select Enable Debugger. To learn how to use the debugger, execute this command line in Igor:
DisplayHelpTopic "The Debugger"
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
August 29, 2011 at 09:41 am - Permalink
http://www.astahost.com/info.php/Prime-Number-Generator_t11203.html
http://www.daniweb.com/software-development/python/code/216558
http://www.codingforums.com/showthread.php?t=167710
You might consider posting your code on the Code Snippets tab once you have it operating, as other folks might likely find it useful.
Perhaps someone resourceful could even use one of these algorithms to create an XOP with a function primes(ww) that fills wave ww with primes.
Also note the statement in one of these URLs that 1 is apparently now no longer considered the first prime number.
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
August 29, 2011 at 10:00 am - Permalink
I find out the mistake by using debugger!
So much thanks!
------------------------------------------------------------
#pragma rtGlobals=1 // Use modern global access method.
macro test()
pauseupdate
silent 1
killwaves /a
make /N = 100 stack1
variable v1
variable v2
variable count1
variable timeron
variable timeroff
timeron = startmstimer
v1 = 2
v2 = 2
count1 = 0
do
if (v1 == 100)
print "end"
break
else
if ( mod(v1, v2) == 0)
if (v1/v2 == 1)
stack1[count1] = v1
count1 = count1 + 1
v1 = v1 + 1
v2 = 2
else
v1 = v1 + 1
v2 = 2 // <-----------HERE!!!
endif
else
v2 = v2 + 1
endif
endif
while(1)
timeroff=stopmstimer(timeron)
print "Calculation time is...."
print timeroff / 1000
print "ms"
print stack1
endmacro
August 29, 2011 at 11:43 am - Permalink