
Need help programing batch processing
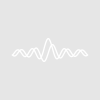
sleepingawake86
So I am doing some image analysis for my research and I need to load and analyze hundreds of photos quickly. I know how to load them with the File loader under the image analysis package, I just can't figure out how to set up a program to go through all of the images quickly and with minimal user effort.
What I am doing is looking at Gaussian burns on a film, and taking a line profile and fitting the Gaussian, here is the code so far:
Function ImageFit(Image2,x_min,x_max,y_min,y_max)
Wave Image2
Variable x_min
Variable x_max
Variable y_min
Variable y_max
ImageTransform rgb2gray Image2
Make/n=2 xTrace={x_min,x_max} ,yTrace={y_min,y_max}
ImageLineProfile /P=-2 /s srcWave=M_RGB2Gray, xWave=xTrace, yWave=yTrace, width=20
Display W_ImageLineProfile
CurveFit W_LineProfile /NTHR=0/TBOX=0 gauss W_ImageLineProfile /W=W_LineProfileStdv /I=1 /D
KillWaves xTrace, yTrace
End
So there are two main things I would like to add....
1: the ability to make it run through a bunch of images without me having to put in each wave name.
2: Output the fit parameters for every fit into a new wave automatically.
Anyone have any ideas?
Try out this function:
You can create a suitable batchstr with a clever call to the 'wavelist' function, e. g.
batchstr=wavelist("image*",";","DIMS:2")
(see the details of the corresponding help file). Be aware that fitCoefficients is overwritten each time doBatchFit is called.In this example the fit coefficients are not put into a single wave, but into a 2D wave where each column corresponds to one image file. This is marked by a dimension label.
If you have a lot of image waves and your fits run very stable, then you may want to remove the line 'Display W_ImageLineProfile' from your function, add a /Q flag to th CurveFit call and remove the /D flag.
The function has not been thoroughly tested, but can serve as a starting point that can be adjusted to your needs.
Andreas
June 29, 2010 at 03:39 am - Permalink
June 29, 2010 at 02:25 pm - Permalink
I would like to have both the fit parameters and the image profiles. Any thoughts on how I can display each line profile in a new graph? I tried this statement in the for-endfor:
Profiles[][ii]=W_ImageLineProfile
setdimlabel 1,ii,$nameofWave(image2),Profiles
it just ended up giving me the same profile for every image as I think each iteration overwrote the previous data. Any help?
and thanks so far!
June 29, 2010 at 02:45 pm - Permalink
You have to rename (or duplicate) the wave W_ImageLineProfile that is created by the ImageLineProfile operation, because as you have assumed, it is overwritten by each call to ImageLineProfile.
June 30, 2010 at 12:35 am - Permalink
If you are copying W_ImageLineProfile to the Profiles wave then you don't need to duplicate it. However, you must copy the contents of W_ImageLineProfile to Profiles after each call to ImageLineProfile.
Since I have not seen the entire procedure, I'm not sure what the flow is. If you continue to have problems you might want to post the entire procedure as an attachment.
June 30, 2010 at 07:16 am - Permalink