
"No symbolic path of that name"
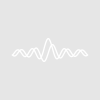
Xuttuh
Dear all, this function gives me an error "no symbolic path of that name":
Function Import_single_CSV_file(pathname,identifier,file_number) String pathname String identifier Variable file_number String import_file_name pathname=ParseFilePath(5,pathname,"*",1,0) NewPath/O stuff,pathname //String stuff=ParseFilePath(5,pathname,"*",1,0) //print stuff // Import import_file_name = identifier+"_"+num2str(file_number)+".csv" LoadWave /J /A=temp_ /O /Q /K=0 /P=stuff /L={0,1,0,0,0} import_file_name Print ">> The file "+import_file_name+" was successfully loaded!" return 0 End
The code returns the above error message, when the code is compiled and executed under IGOR 8 (trial).
After quitting the error message and deleting everything from the "data browser", the code works then just fine on second try and does not return the error message.
I have commented out some of the iterations I tried to make it work. Could anyone point me to the mistake I made or what is going on?
I am working under Windows 10, but the code needs to run on Mac, too.
Thank you and kind regards!
I don't see anything obvious wrong in your code. You can use PathInfo/S to print the disc path a symbolic path points to. Something like
should help in debugging.
August 12, 2021 at 03:22 am - Permalink
First, I don't get "no symbolic path of that name" from your code calling it like this:
Import_single_CSV_file("C:Test Folder", "Identifier", 0)
I do get "The operation could not be completed because the specified volume cannot be found".
But there are more fundamental issues.
First, we the terminology "pathName" in Igor to mean "name of an Igor symbolic path". You are using it, I think, for a full path to a folder. I recommend using fullPath as the name.
Second, if you have a full path, you don't need to use a symbolic path. The point of a symbolic path is to point to a folder in place of a full path. It also reduces issues with path separators. So, I would write your function like this:
So far so good. But I recommend using symbolic paths rather than full paths. This allows you to switch folders by merely changing the path associated with a symbolic path. So I would write the function like this:
Note /P=$pathName. The $ operator converts the contents of a string into a name. This is necessary because the /P flag expects the name of a symbolic path. Execute this for details:
DisplayHelpTopic "String Substitution Using $"
Something else to be aware of is the fact that backslash is an escape character in Igor (as in C and C++) so it needs to be treated specially. Execute this for details:
DisplayHelpTopic "Path Separators"
For more examples using LoadWave programmatically, execute this:
DisplayHelpTopic "Loading Waves Using Igor Procedures"
August 12, 2021 at 03:56 am - Permalink
One more thing...
This command from the original function:
pathname=ParseFilePath(5,pathname,"*",1,0)
is intended to convert a full path to a Macintosh HFS (using colons) path on Macintosh and a Windows (using backslashes) path on Windows. However, it is not necessary because Igor accepts Macintosh or Windows paths on both platforms and does the necessary conversions internally. ParseFilePath(5,..) is needed mostly when you are going to pass a path to another program, such as when calling ExecuteScriptText.
As explained in the help topic "Path Separators", backslash is an escape character in Igor so, to use a backslash in a literal string, you need two backslashes. For example:
String fullPathToFolder = "C:\\Users\\Howard\\Data"
You can avoid the backslash issue by using Macintosh HFS (colon-based) paths regardless of the platform you are running on:
String fullPathToFolder = "C:Users:Howard:Data" // Works on Macintosh and Windows
August 12, 2021 at 05:05 am - Permalink