
Parse through textwave to look for specific character
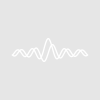
keblb1dk
Hello, I'm trying parse through a textwave that has data points containing things like "(4+)", "3+", "(17/2)-", "1(+)", "3/2-" , "(1,2)", etc. What I want to do is check if a data point contains a "+" or a "-" and if it does, put a "+" or a "-" respectively, into a new wave but in the same index as the wave being searched. I.e. if index (data point) 6 contains a "+", put a "+" in index 6 of the new wave.
I'm running into a problem where any data point that contains a "," , "/" , and "()" it doesn't work. Can someone help explain why StrSearch isnt working for this?
Function test() wave/T test_PI variable len = numpnts(test_PI) variable i make/O/T/N=(len) PI_tested For(i=0;i<len;i+=1) If(StrSearch(test_PI[i],"+",0)==1) PI_tested[i] = "+" EndIf If(StrSearch(test_PI[i],"-",0)==1) PI_tested[i] = "-" EndIf EndFor End
StrSearch returns the byte offset of the character you are trying to match e.g. "(4+)" would give 2. If you don't care about the position of the character then maybe you need to use StringMatch, or GrepString, or just change ==1 to >-1 i.e. (StrSearch(test_PI[i],"-",0) >-1)
June 10, 2021 at 07:29 am - Permalink
In reply to StrSearch returns the byte… by cpr
Changing If(StrSearch(test_PI[i], "+", 0)==1) to If(StrSearch(test_PI[i], "+", 0)>-1) worked perfectly, thank you!
June 10, 2021 at 07:36 am - Permalink
Something such as this may be helpful.
(edit -- now sets values for both negative and positive signs)
June 10, 2021 at 12:46 pm - Permalink