
Parsing Strings and naming waves
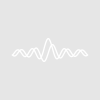
n.black
When I load waves in Igor I get the filename as a string. My filename is too long to have as a wavename but I'd like to abbreviate the filename to do so.
I was hoping someone could point me in towards the relevant literature to get an idea how to do this so I can read around and make better code.
For example I'll name my file S1_variable1_variable2_variable3_variableN.txt
If I wanted to have my igor wave name as "S1_variableN" How would I Achieve this?
Under a similar line of thought. When I mass duplicate waves I will make for example a line of code:
Wave Wave1 String NewName = NameOfWave(Wave1) + "_duplicate" Duplicate Wave1, NewName
After I now have wave1 and wave1_duplicate.
Is there a way to take wave1_duplicate name and turn it into wave1_transposed. Here I'd like to replace the "_duplicate" with "_transpose" for example.
I guess this should be under string manipulation or something? I'm not sure where to look in the manual to help get me started. I'm completely self taught and I feel there must be a chapter somewhere in the manual explaining this stuff.
Sorry this is kind of vague but thanks for any help you can give.
Best,
N
ReplaceString
for these kinds of tasks.In the first example
ReplaceString("variable1_variable2_variable3_",fileName,"")
If you don't know what variable1_variable2 etc. are you can read them using SplitString or put the string together using the possibilities if they are a combination of known variables.
Second example,
ReplaceString("_duplicate",NewName,"_transpose")
ReplaceString works on the same string you don't have to make a new string, e.g.
NewName = ReplaceString("_duplicate",NewName,"_transpose")
FWIW I would shorten _duplicate to _d to save on characters especially if you are near the limit.
February 10, 2017 at 04:42 am - Permalink
This looks exactly what I need.
Thanks so much,
N
February 10, 2017 at 05:42 am - Permalink
Sounds like you might want to look into String Indexing. But this is only useful if your filenames are consistent and predictable. Following your example
This results in sNewName having the value "S1_variableN"
Other useful functions in this realm are:
See if the
Rename
operation meets your needs.It has the form
Rename oldName, newName
But it can't overwrite an object with the same name.
February 10, 2017 at 08:29 am - Permalink
1. Using sscanf is sometimes easier than using SplitString, especially if you're not a regular expressions guru.
2. You may find the following functions useful in cases like this: CleanupName, CheckName, UniqueName
February 10, 2017 at 07:40 am - Permalink
In reply to by jtigor
Strange, but instead of the actual new name I'm getting the newName as the name of my renamed wave! What am I doing wrong? newName is a string type.
Edit: ok I found the solution:
Rename oldName, $newName
February 5, 2020 at 12:25 am - Permalink