
Plot Multiple Traces on Graph from Procedure Not Working
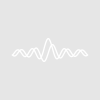
Dear all,
Igor beginner here.
I've written a procedure containing a function which takes time of flight data waves "Spectrum_01, Spectrum_02 . . ." as an argument. I perform calculations on those waves to convert to kinetic energy in the procedure and eventually want to plot each of the resultant calculated waves deriving from Spectrum_01, Spectrum_02 on the same graph.
I can plot the kinetic energy wave deriving from Spectrum_01 from the procedure, however when I call the function using Spectrum_02 as the argument, the graph updates to show only the data deriving from Spectrum_02, it does not append to the graph already showing the Spectrum_01.
Probably very simple, just not getting it at the moment.
#pragma TextEncoding = "UTF-8" #pragma rtGlobals=3 // Use modern global access method and strict wave access #pragma DefaultTab={3,20,4} // Set default tab width in Igor Pro 9 and later //constants //-------------------------------------------------------------------------------------------------------------------------------------------------- static constant PlanckConstant = 6.62607015E-34 //units of Joule seconds static constant speedofLight = 299792458 //units of metres per second static constant electronMass = 9.1093837E-31 //mass of electron in kg static constant electronCharge = 1.60217663E-19 //electron charge in C //-------------------------------------------------------------------------------------------------------------------------------------------------- //Conversion of Time of Flight to Electron Kinetic Energy function TOF2eKE(wave amplitude, variable DetectorPosition) //Variable declarations (^function parameters declared in-line ^) variable ScaleValue = 1e-09 //use this value for SetScale and for increment value in peak-finding algorithm later variable TOFlength = ((100-DetectorPosition) + 617.22)/1000 //TOF Length in metres //Set x-scaling and compute x values, storing them in TOF wave //----------------------------------------------------------------------------------------------------------------------------------------------------------------------- SetScale/P x 0, ScaleValue,"seconds", amplitude //set x-scaling such that computed x-values are in the correct increments of the digitization time bin Duplicate /O amplitude, TOF //Duplicate amplitude wave and rename this new wave TOF; ensures dimensions are always the same TOF = x //extract calculated x-values and reasign TOF wave with x-values //----------------------------------------------------------------------------------------------------------------------------------------------------------------------- //Crude Calibration Parameters (do not need to be so accurate for first iteration //----------------------------------------------------------------------------------------------------------------------------------------------------------------------- variable TemporalOffset = 0 //temporal offset in seconds, from calibration routine variable CalibrationLength = TOFLength //actual electron trajectory distance from calibration routine. Should be close to physical flight tube length. In metres. variable EnergyOffset = 0 //Energy offset from calibration routine, in J //Changed to eV //--------------------------------------------------------------------------------------------- //Calculation of the Jacobian Scaling Factor in converting from time to energy //---------------------------------------------------------------------------------------------- variable TOFlengthsquared = TOFlength^2 Duplicate /O amplitude, Jacobian //Duplicate amplitude wave to ensure the Jacobian has correct dimensions Jacobian = (electronMass*TOFlengthsquared)/((TOF-TemporalOffset)^3) //--------------------------------------------------------------------------------------------- //Calculation of eKE without Jacobian Scaling Factor //---------------------------------------------------------------------------------------------- Duplicate /O TOF, eKE eKE = (0.5*electronMass*TOFlengthsquared)/(TOF-TemporalOffset)^2 //--------------------------------------------------------------------------------------------- //Calculation of eKE scaled with (corrected by) Jacobian Scaling Factor //---------------------------------------------------------------------------------------------- string AmplitudeTraceName = NameOfWave(amplitude) +"Jacobian Scaled Amplitude" Duplicate /O eKE, eKE_Offsetcorrected //Duplicate eKE wave for new eKE wave of same dimensions which will be corrected by energy offset, in Joules Duplicate /O eKE, eKE_Offsetcorrected_eV //Duplicate eKE wave for new eKE wave of same dimensions which will be corrected by energy offset, in eV Duplicate /O amplitude, JacobianScaledAmplitude //Duplicate TOF amplitude wave for new amplitude wave of same dimensions whhich will be corrected by the Jacobian JacobianScaledAmplitude = (Jacobian)*amplitude //--------------------------------------------------------------------------------------------- //Subtraction of any energy offset from electron kinetic energies, giving the energy offset eKE in units of eV //---------------------------------------------------------------------------------------------- eKE_Offsetcorrected_eV = ((eKE_Offsetcorrected-EnergyOffset)/electronCharge) //--------------------------------------------------------------------------------------------- //Plot //---------------------------------------------------------------------------------------------- DoWindow /F /N /T electronKineticEnergy, "Electron Kinetic Energy(eV)" Display /N=electronKineticEnergy AppendToGraph JacobianScaledAmplitude /TN= AmplitudeTraceName vs eKE_Offsetcorrected_eV end
I think I figured this out.
I reconfigured the function above to return the two waves of interest:
February 20, 2023 at 01:41 am - Permalink
You have taken a good step in separating the processing function from the plot function. I might go further in the way below.
February 20, 2023 at 07:52 am - Permalink