
plotting multiple waves
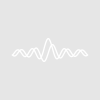
agrockowiak
Hello,
I am trying to plot some data. The spectra I'd like to plot are obtained on a grid, and I'd like to plot the resulting spectra line by line. The data collected has a standardized name Gridname-SpectrumNumber.xy. The spectrum number is generated by the instrument collecting the data and is a 5 digit number starting at 00001. Here is the code I wrote, however it plots only the first 9 spectra, and does not find the waves for the spectra number 10 and higher. Any suggestion on what I am doing wrong ?
#pragma TextEncoding = "UTF-8" #pragma rtGlobals=3 // Use modern global access method and strict wave access. Function PlotLine() String CurrentGrid = "d003_p01_g1" Setdatafolder root:$Currentgrid variable gridX =2 variable gridY = 13 variable i //number of lines variable j //number of columns variable filenumbermax = gridX*gridY for (i=1; i<=gridX; i+=1) string Name = CurrentGrid+"_testline"+num2istr(i) print Name Display DoWindow/C/T $Name, "Name" for (j=1; j<=gridY; j+=1) variable filenumber = ((i-1)*gridY)+j print filenumber if(1<=filenumber<=9) wave Yaxis = $CurrentGrid+"-0000"+num2istr(filenumber)+".xy_I" wave Xaxis = $CurrentGrid+"-0000"+num2istr(filenumber)+".xy_2th" AppendToGraph Yaxis vs Xaxis ModifyGraph offset($CurrentGrid+"-0000"+num2istr(filenumber)+".xy_I")={0,10*j} elseif (10<=filenumber<=99) wave Yaxis = $CurrentGrid+"-000"+num2istr(filenumber)+".xy_I" wave Xaxis = $CurrentGrid+"-000"+num2istr(filenumber)+".xy_2th" wavestats Yaxis AppendToGraph Yaxis vs Xaxis ModifyGraph offset($CurrentGrid+"-000"+num2istr(filenumber)+".xy_I")={0,10*j} endif endfor endfor end
The way you write your conditions is wrong, i.e., you write:
but rather mean:
Also, note that you can get rid of the conditional statement if you generate your file string using sprintf instead, e.g., like this:
Output is like this:
May 31, 2023 at 07:46 am - Permalink
Thank you for your help ! That solved my first problem ! My other problem would be changing the title of each graph window, right now it just says name, and not the value of the string name defined for each line. Any suggestion ?
May 31, 2023 at 07:55 am - Permalink
I think you could to use the Display command itself to set the Window name and window title.
Display/N=$(Name) as $Name
and drop the DoWIndow/C/T line. Sounds to me easiest to do here. Did not have chance to test this, so I am not sure if these really should be $Name or if there should be only Name. Just try it and see.
May 31, 2023 at 08:26 am - Permalink
Be careful here what you want: A graph has both an internal 'name' and a title which is displayed in the top bar. I think you just want to modify the latter, so use
Display as Name
The internal name is for the purpose of addressing the graph in code. This might be important if you want to change the graph programmatically later on. I strongly advise against using the dataset name as input for this. Also, just appending a number to a fixed string can get you in trouble (=> i.e., when the graph with this name already exists).
Here is what I do:
Obviously, choose something more descriptive than "MyGraphBaseName".
May 31, 2023 at 09:25 am - Permalink