
popup menu help
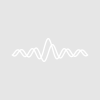
pheonixelemental
Wed, 06/23/2010 - 11:22 am
data to get from wave m_interpolatedimage (I currently have this in a table but am open to any ideas)
Section1 Section 2 Section 3 Section 4 Section 5
xmin -50 10 -50 -50 10
xmax -10 50 50 -10 50
ymin 71 71 8 -8 -8
ymax 90 90 70 7 7
code to get this
duplicate /r=(xmin,xmax)(ymin,ymax) m_interpolatedimage tempextract1
wavestats tempextract1
Then I need the following data to display or go into a wave
V_avg, V_min, V_max, V_sdev
any help would be great! THANKS!!
ImageStats/GS={xmin, xmax, ymin, ymax} m_interpolatedimage
There is no need to copy a subset of the image to a temporary wave. Note that the results are NOT printed in the history window as is the case for WaveStats. Rather, they are returned as global variables (if you execute ImageStats from the command line) or as local variables (if called from a function) named V_avg, V_min, etc.
If you want to "automate" this, I guess there are two ways to go about it. I have written some quick sample code below.
1) If you want to operate on many images and if the section coordinates are always the same, preparing a table with those coordinates (as you did) seems the best solution. You can then write a function that operates on those coordinates and puts the ImageStats results in a wave.
I have called my sample function "ImageStatsFromWave". In my code, the ImageStats results are appended as new rows to your "SectionX" waves. If you prepare a two-dimensional waves (say, "AllSections") by executing
Make/O/N=(4,5) AllSections; Edit AllSections
on the command line, and by copy+pasting the contents of your table-of-1D-waves into the new table, you'll have to call the function only once:
ImageStatsFromWave(AllSections, m_interpolatedimage)
Otherwise, you'll have to call it for each of your 1D waves "Section1", "Section2", etc.
2) If you want to be more "interactive", you might want to mark the region of interest on your image with the mouse and then select a context menu item (by clicking in the Marquee rectangle) that lets you directly print the ImageStats results to the history window. This can be done using Igor's "GetMarquee" function. The code below consists of two parts, the function
ImageStatsFromMarquee()
and the menu definitionMenu "GraphMarquee"
(see Igor's help files for more on that).// the stats results are appended as new rows
function ImageStatsFromWave(sections, imageWave)
wave sections, imageWave
Redimension/N=(8,-1) sections
variable k, kmax = DimSize(sections,1)
if( kmax == 0 ) // 1D wave
kmax = 1
endif
for( k=0; k < kmax; k+=1 )
ImageStats/GS={sections[0][k], sections[1][k], sections[2][k], sections[3][k]} imageWave
sections[4][k] = V_avg
sections[5][k] = V_min
sections[6][k] = V_max
sections[7][k] = V_sdev
endfor
end
// version 2: section is taken from a graph marquee
// the stats results are printed into the history window
function ImageStatsFromMarquee()
wave imageWave = $(StringFromList(0, ImageNameList("", ";"))) // get topmost image
if( !WaveExists(imageWave) )
print "ImageStatsFromMarquee works only on images!"
return -1
endif
string axes = ReplaceString(";",RemoveEnding(AxisList("")),",") // get axes' names as comma-separated list
Execute/Q/Z "GetMarquee/K "+axes // have to execute since axis names (not strings) are expected
if( V_flag )
print "ImageStatsFromMarquee error: no marquee?" // should never happen
return -2
endif
NVAR V_left, V_top, V_right, V_bottom
ImageStats/GS={V_left, V_right, V_top, V_bottom} imageWave
if( V_flag )
print "ImageStatsFromMarquee error: illegal coordinates?" // may happen if marquee larger than image
return -3
endif
string format = "%s(%g,%g)(%g,%g): avg: %g; min: %g; max: %g; sdev: %g\r"
printf format, NameOfWave(imageWave), V_left, V_right, V_top, V_bottom, V_avg, V_min, V_max, V_sdev
end
Menu "GraphMarquee"
"Print Local Image Statistics", /Q, ImageStatsFromMarquee()
End
If you copy the text above into your Procedure window and compile that, you can experiment with the sample code...
HTH,
Wolfgang
July 17, 2010 at 12:11 pm - Permalink