
Set the same name for waves
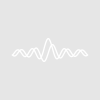
raponzoni
I've written a procedure to load all TXT files of a folder. As my text files have 2 columns IGOR automatically names them as wave0, wave1, wave2...
The first column of all files is the same. I have spectroscopy data, and the X axis is the same for all. So to be easy when plotting a graphic (I have 400 files that will be plotted in the same graphic then averaged) I'd like to rename all even waves (wave2, wave4, wave6..) as wave0 or any possible name. Is it possible?
Bellow is what I've written so far:
#pragma rtGlobals=3 // Use modern global access method and strict wave access. function loadwaves(filename, pathname) string filename string pathname if (strlen(pathname)==0) newpath/o temporaryPath if (v_flag!=0) return -1 endif pathname= "temporarypath" endif variable index = 0 do filename= indexedfile ($pathname, index, ".txt") if (strlen (filename)== 0) break endif loadwave/a/g/o/d/p=$pathname filename if (v_flag==0) break endif index+=1 while(1) end
Thanks in advance :)
I would also suggest either using the Rename operation to name your data waves something meaningful related to each file (perhaps the filename??) or using Note to add a note to each wave to describe it. Looking at the file later to see wave2, wave4, wave6.... will be confusing and difficult to discern what each wave represents.
May 24, 2013 at 08:07 am - Permalink
See the section "Specifying Characteristics of Individual Columns" in the documentation for LoadWave.
There is an example of this at http://www.igorexchange.com/node/1538
For a more complex case you can programmatically generate the /B parameter string.
As proland points out, each wave in a given data folder must have a unique name.
May 24, 2013 at 08:39 am - Permalink
Your suggestion and help about killing the x-wave was brilliant, it really worked and finally I can load the hundreds of spectra.
Although I'm still having some trouble with the rename function, more precisely with the RemoveEnding function. An error occurs and it says "To invoke a built-in function you must print or assign the result".
Above is your suggestion, so I've just added a Print command before the removeending function and I could compile:
But, when executing the rename another error appears "name already exists as a string function" . This one I couldn't solve, the waves were loaded but not renamed.
Thanks for the help!
Cheers
May 28, 2013 at 03:30 am - Permalink
rename $(stringfromlist(1, s_wavenames, ";")), cleanupname(removeending(s_filename, ".txt"), 0)
There needs to be a comma between the old name and new name in the rename operation. Also, cleanupname has a required parameter to determine whether liberal names can be used. The above line does not permit liberal wave names. This is the purpose of the final ", 0". You might look up rename, cleanupname and revomeending in command help for more details. Finally, if there is a chance that you might have duplicate files names (either from different directories or after cleaning them up), take a look at uniquename.
May 28, 2013 at 05:34 am - Permalink
My procedure, with all alterations now looks like this:
Thanks
May 28, 2013 at 06:11 am - Permalink
rename $(stringfromlist(1, s_wavenames, ";"), cleanupname(removeending(s_filename, ".txt"), 1) )
EDIT: This solution is incorrect... see below.
By the way, why don't you load the x wave from one file outside the loop and then just load the second (data) wave from each file inside the loop? I don't know the syntax for this, but you should be able to specify specific column(s) to load w/o loading all columns. It really may not be all that more efficient in this case, but it would eliminate the needless killing of innocent waves. This comment comes from a reading of your initial post. In the code that you show just above, it doesn't appear that you are saving any x waves. Is this what you want?
May 28, 2013 at 07:52 am - Permalink
Unfortunately the rename isn't working. The same error of expecting a comma right after the 1 is still happening.
With all the changes the procedures now is:
I need to rename the waves as my file names once I have more than 400 files which correspond to spectra that need to be plotted in the same graph, for being averaged after!
If this procedure works it will save much time!
So thank you again :)
May 28, 2013 at 07:03 am - Permalink
There were errors in my advice offered several comments above. This one should be correct.
rename $(stringfromlist(1, s_wavenames, ";")), cleanupname(removeending(s_filename, ".txt"), 1)
May 28, 2013 at 07:40 am - Permalink
rename $stringfromlist(1, s_wavenames, ";"), $cleanupname(removeending(s_filename, ".txt"), 1)
May 28, 2013 at 07:50 am - Permalink
Perhaps we were misunderstanding each other.. sorry.
Attached is a screenshot of the error.
Cheers
May 28, 2013 at 08:29 am - Permalink
I didn't see your last message! Just trying it again!!
Thanks a lot!
May 28, 2013 at 08:34 am - Permalink
As I've skipped the first column of my data, in this rename parameter IGOR expects to have a name of wave, variable or string as the attached file shows.
If you still wanna take look go ahead!
Here the entire procedure:
Sorry for all these questionings.
May 28, 2013 at 08:54 am - Permalink
rename $(stringfromlist(1, s_wavenames, ";")), cleanupname(removeending(s_filename, ".txt"), 1)
and got the error as you describe.
But it compiles without error when written like this
rename $(stringfromlist(1, s_wavenames, ";")), $cleanupname(removeending(s_filename, ".txt"), 1)
May 28, 2013 at 08:55 am - Permalink
You mean the error about invoking a built-in function right?
With your help this was fixed, although a new one was created :P
In my previous message I attached a screenshot of the error when running the function in the command line..
cheers
May 28, 2013 at 09:02 am - Permalink
I recommend writing it as:
In this form it can be debugged with Print statements or using the Debugger.
The CleanupName call is not necessary. We know that name contains the name of a wave loaded by LoadWave. Therefore name must contain a valid name and it does not need to be cleaned up. It would need to be cleaned up if you wanted to force a standard rather than a liberal name. You would do this by changing the CleanupName parameter from 1 to 0.
May 28, 2013 at 09:32 am - Permalink
May 28, 2013 at 09:39 am - Permalink
Are your sure that your files have the expected columns?
Howard's suggestions related to breaking up the rename command so that print statements can be used to diagnose the problem are very good. Is the debugger enabled and, if so, do you know how to use it?
May 28, 2013 at 10:46 am - Permalink
Finally my procedure is working properly. I've used the /B flag as hrodstein suggested but replacing the first and second column info, once I needed to skip the first and rename the second.
Here is the final procedure, totally working if someone needs to load all files of a folder and skip/rename columns.
Thanks again to everyone who helped!!
Cheers
May 29, 2013 at 01:42 am - Permalink