
Splitting waves based on value in one wave
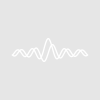
Soepvork
I have a set of waves which I want to split based on the value for that point in one particular wave. Basically, I have multiple waves which contain my data, and one wave which contains an id-number to distinguish between different data set. Based on that id-number, I'd like to cut the large multiple waves (containing data for all sets) into smaller waves containing a single data set.
Let's say I have two data waves, wave_x and wave_y, and an wave with the id-number, wave_id. Based on the value of a point in wave_id, I'd like to separate the waves. In particular, the values of my wave_id are of three types: it's a 2, it's dividable by 6, or it's dividable by 6 once you add 2 to the value (so either 2, 6n, or 4+6n)
Now, I want to split wave_x and wave_y into three seperate sets (6 waves): one wave_x and wave_y for id=2, one of each for id=6n, and one each for id=4+6n.
Does anyone know if there is an easy way to do that?
Many thanks,
Stanley
The following function should help you out. Advanced Igor users will observe that it is inefficient due to the repeated calls to
Redimension
, but that is unlikely to be an issue unless you have very large waves.September 16, 2011 at 11:32 am - Permalink
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
September 16, 2011 at 12:08 pm - Permalink