
subtract element (n-1) from element n
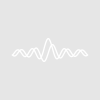
tdgordon
I have a date/time wave and I want to create a new wave that has the difference between adjacent time points. Is there a clever way to do this without an explicit loop? Related to this, as always when I've been away from Igor for awhile, I've forgotten how to call a set of waves that have names that change each time through the loop. See below.
do
n=0
string temp_string="degF_t"+num2str(i)
string time_string="igor_time_t"+num2str(i)
string time_string_temp="igor_time_t"+num2str(i)+"temp"
Extract/O degF,$temp_string,thermistor==i
Extract/O igor_time,$time_string,thermistor==i
SetScale d 0, 0, "dat", $time_string
duplicate $time_string $time_string_temp
for(n=1;n<dimsize($time_string_temp,0);n+=1) // Initialize variables;continue test
$time_string_temp[0]==0
$time_string_temp[n]=$time_string[n]-$time_string[n-1] // Condition;update loop variables
endfor // Execute body code until continue test is FALSE
$time_string_temp[n]= //??? want to set something so that on the final loop we do not have an index out of range error...
i+=1
while(i-1<thermistor_max)
This is one approach to create a difference wave using implicit indexing:
The first point in the new wave has no meaning here.
I also suggest that a better approach to avoid using "$" throughout is to use wave declarations immediately after the waves are created:
Finally, you might consider replacing the main do-while loop with a for-endfor loop:
December 23, 2019 at 11:42 am - Permalink
Thank you!
December 23, 2019 at 12:37 pm - Permalink
Just a small thing- on the left side of a wave assignment, you can simply leave out the last point number. That's a shortcut for "up to and including the last point":
January 2, 2020 at 01:37 pm - Permalink