
unknown/inappropriate name or symbol
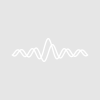
BLawrie
Fri, 06/22/2012 - 10:37 pm
wavesize=numpnts(conjugate)/2
Make/N=(wavesize) conj2, probe2
for(i=0;i<=wavesize;i=i+1)
conj2[x2pnt(conj2,i)]=(conjugate[x2pnt(conjugate,2*i)]+conjugate[x2pnt(conjugate,2*i+1)])/2
probe2[x2pnt(probe2,i)]=(probe[x2pnt(probe,2*i)]+probe[x2pnt(probe,2*i+1)])/2
endfor
However, Igor complains that all occurrences of conjugate and probe within the for loop represent an "unknown/inappropriate name or symbol." Any suggestions would be greatly appreciated.
Edit wave0
Make /O /N=(numpnts(wave0)/2) wave1 = mean(wave0, 2*p, 2*p+1)
AppendToTable wave1
This is a "wave assignment statement" and is much faster than a loop. To read about it, execute:
Now to your original question. If you were trying to do this in a user-defined function, the problem is that you need to tell Igor what conjugate and probe are (waves?, numeric variables?, string variables?, operations?) using "wave references". Here is an example:
Wave conjugate, probe // Create wave references
Variable wavesize=numpnts(conjugate)/2
Make/N=(wavesize) conj2, probe2
Variable i
for(i=0;i<=wavesize;i=i+1)
conj2[x2pnt(conj2,i)]=(conjugate[x2pnt(conjugate,2*i)]+conjugate[x2pnt(conjugate,2*i+1)])/2
probe2[x2pnt(probe2,i)]=(probe[x2pnt(probe,2*i)]+probe[x2pnt(probe,2*i+1)])/2
endfor
End
For help on wave references:
The Test1 function is of limited use because it is hard-coded to use waves named conjugate and probe and can not be used for any other waves. This can be fixed by making the input waves parameters to the function so that any pair of waves can be passed in.
Wave conjugate, probe // Wave references passed as parameters
String conjugate2Name = NameOfWave(conjugate) + "2"
String probe2Name = NameOfWave(probe) + "2"
Variable wavesize=numpnts(conjugate)/2
Make/N=(wavesize) $conjugate2Name, $probe2Name
Wave conj2 = $conjugate2Name // Create wave reference
Wave probe2 = $probe2Name // Create wave reference
Variable i
for(i=0;i<=wavesize;i=i+1)
conj2[x2pnt(conj2,i)]=(conjugate[x2pnt(conjugate,2*i)]+conjugate[x2pnt(conjugate,2*i+1)])/2
probe2[x2pnt(probe2,i)]=(probe[x2pnt(probe,2*i)]+probe[x2pnt(probe,2*i+1)])/2
endfor
End
For an explanation of the $ symbol:
Now we improve this by using wave assignment statements instead of a loop:
Wave conjugate, probe // Wave references passed as parameters
String conjugate2Name = NameOfWave(conjugate) + "2"
String probe2Name = NameOfWave(probe) + "2"
Variable wavesize=numpnts(conjugate)/2
Make/N=(wavesize) $conjugate2Name, $probe2Name
Wave conj2 = $conjugate2Name // Create wave reference
Wave probe2 = $probe2Name // Create wave reference
conj2 = mean(conjugate, 2*p, 2*p+1)
probe2 = mean(probe, 2*p, 2*p+1)
End
June 23, 2012 at 04:20 am - Permalink
I would question your assumption that the most appropriate way of compressing the data is to average pairs of points. This might be appropriate if the 2-point intervals were themselves separated. However if the original data is evenly sampled, you might consider a simple alternative wherein each point is replaced by a weighted sum of itself and two adjacent neighbors. This is exactly what the Smooth operation, with 'num' equal 1 does: the weighting coefficients are 1/4, 1/2, 1/4. Then, in the smoothed wave take every other point to get the smaller wave with half the number of points. For example
Smooth 1, conj2big
Make/O/N=(numpnts(conjugate)/2) conj2
setscale/P x, leftx(conjugate), 2*deltax(conjugate),"" conj2
conj2 = conj2big[2*p]
You could also create 'conj2big' as a /FREE wave in your procedure, or kill it when done.
June 26, 2012 at 11:37 am - Permalink
To perform the comparison I used a wave of white Gaussian noise. This has more or less uniform frequency content over its entire spectral range. Two smaller waves, with half the points, were calculated from the large wave using the two methods described previously, and scaled to the same range as the initial wave (but with 2x the sample interval). Then the (complex) FFTs were compared to the original wave's FFT over the low-frequency half of its domain. (The higher frequency content is not relevant to the down-sampled waves.) For each down-sampled wave the comparison metric was the sum over frequencies of the absolute squares of the complex difference between the down-sampled and original FFTs. The tests were repeated over a large number of trials, and the results were averaged.
The final, averaged spectral deviation for the 3-pt method was 0.68 that of the pair-averaged method. This eased one of my concerns that 3-pt averaging might potentially cause excess filtering, with resultant 'x' domain distortion. This seems not to be the case. I do not claim this method will always be the best to down-sample any waveform, but suggest it is worth considering.
June 28, 2012 at 07:15 am - Permalink
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
June 28, 2012 at 08:58 am - Permalink
pair : 1.00 ; 3-pt smooth : 0.685 ; Resample : 0.653
The winner is.......RESAMPLE !! (but not by much)
In the original 'x' domain, the 3-pt and Resample waves were quite close to each other, and distinct from the pair-sampled waves.
Thanks for the suggestion, John.
June 28, 2012 at 10:58 am - Permalink