
Use wave1 to set color scale of image of wave2
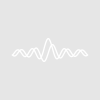
I have a 2D wave (DiffWave) which is the normalized difference of two 2D waves (wave1 and wave2). I would like to create a 2D colortable, where the color of DiffWave is determined by the value of DiffWave, while the saturation of the color is determined by the value of (wave1+wave2). I have created a 3D wave which holds the 2D colortable (colors are stored along the y axis, saturation is stored along the x axis, RGB is along the z axis).
The way I plan on doing this is to split DiffWave up into a bunch of different waves, binning by color. I would like to use cuts from the 2D colortable to set the saturation of each binned wave based on the values in (wave1 + wave2).
If this is not possible, is there a way to set the color of an image plot pixel-by-pixel?
In general, setting the color of an image plot is effectively creating an RGB(A) image (3 or 4 layer wave). You then explicitly compute the color components of each pixel using any rules you wish.
Another approach is to set colors is using:
ModifyImage cindex=matrixWave
You will have to construct the wave matrixWave to contain the range of colors that you want to use.
It seems to me that you have enough parameters to display that you may want to consider using Gizmo to create a surface plot. This can allow you to display the original wave1 and wave2 and with some tweaking you can illustrate their differences pretty well. If you would like to explore this approach feel free to send me a copy of the experiment to support@wavemetrics.com and I will show you some options.
A.G.
August 25, 2020 at 10:16 am - Permalink
In reply to In general, setting the… by Igor
I think this is what I want, but I'm not really clear on how to implement this, could you be more explicit?
For this approach, how would you construct matrixWave? Doesn't matrixWave need to be a 2D wave with either 3 or 4 columns? Or would I need a separate matrixWave for each color bin layer?
Even though surface plots are considered unconventional in my field, I am curious how this would look. I will DM you.
August 25, 2020 at 11:50 am - Permalink
Here is an example of creating an RGB image directly:
August 26, 2020 at 04:32 pm - Permalink