
Value ± Error rounding
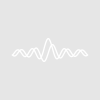
KurtB
I have as an input a value and its error (uncertainty). I want to create a string that is the Value ± Error but with appropriate rounding on both numbers. By appropriate rounding, I mean 2 significant figures in the error value.
I have written the following function that does mostly does this, but has some exceptions to which I am struggling to find a simple resolution.
Function/S FormatValueError(vVal,vErr) variable vVal variable vErr // Return string containing value ± error rounded to 2 sf on error // or "" if there was a problem string sValErr = "" string sVal,sErr variable vPower do if( numType(vVal) == 2) //NaN break // return empty string endif if( (numType(vErr) == 2) || (vErr == 0) ) // NaN or zero sprintf sValErr, "%g", vVal // just return vVal break endif // Find power 10 of Error variable vErrPower = floor( log( abs(vErr) ) ) vErrPower -= 1 // go to 2 sf // Find power 10 of Value variable vValPower = floor( log( abs(vVal) ) ) if( vValPower < vErrPower ) // catch value less than error vPower = vValPower - 1 //round to 2 sf in Value else vPower = vErrPower // round to 2 sf in Error endif // Now do the rounding vVal=sign(vVal) * (10 ^ vPower) * round( abs(vVal) * (10 ^ (-vPower) ) ) sprintf sVal,"%g",vVal vErr=sign(vErr) * (10 ^ vPower) * round( abs(vErr) * (10 ^ (-vPower) ) ) sErr=num2str(vErr) sprintf sErr,"%g",vErr // assemble the result sValErr = sVal if( strLen(sErr) > 0) sValErr += " ± " + sErr endif break while(1) return sValErr End
For example:
print FormatValueError(123.456,7.123456)
gives 123.5 ± 7.1 (correct)
print FormatValueError(1.008,0.111)
gives 1.01 ± 0.11 (correct)
print FormatValueError(1.0008,0.111)
gives 1 ± 0.11 (no - I would like to get 1.00 ± 0.11)
print FormatValueError(123.456E-4,1.345678E-5)
gives 0.012346 ± 1.3e-05 (no - I would like 0.012346 ± 0.000013 or 1234.6e-5 ± 1.3e-5)
Is there a cunning way of achieving the last two results without doing a heavy handed look for each type of number format and deal with each foreseeable situation bit by bit?
Thank you,
Kurt
Try
printf
andsprintf
.February 21, 2013 at 07:56 am - Permalink