
Allow waves for multiple parameters in built-in functions
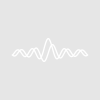
gsb
Built-in function parameters are often specified like this:
Slider limits = {low,high,inc}
I'd often like to be able to use syntax like this:
Slider limits=myLimitsWave //where myLimitsWave would presumably be a 3-point 1-dimensional wave
For an example use case: Sometimes I make a function that is going to iterate over multiple calls of a built-in function. I'll use Slider as an example below. (It could also be ModifyGraph rgb, muloffset, or any function with a multiple-value named parameter.) In such cases I resort to loops like the following:
function buildSliders_currently() newpanel/k=1 int i,numSliders = 3 make/o/free/n=(3,numSliders) minMaxIncr minMaxIncr[][0] = {-100,100,10} minMaxIncr[][1] = {-1000,0,100} minMaxIncr[][2] = {-5,10,.1} for (i=0;i<numSliders;i++) //passing the 3 parameters like this has always seemed cumbersome slider $("slider_"+num2str(i)) limits={minMaxIncr[0][i],minMaxIncr[1][i],minMaxIncr[2][i]} endfor end
But every so often I try (and then fail to compile) code like this. I'd use this syntax regularly if it worked because I find it easier to read and write
function buildSliders_wishedSyntax() newpanel/k=1 int i,numSliders = 3 make/o/free/n=(3,numSliders)/free minMaxIncr minMaxIncr[][0] = {-100,100,10} minMaxIncr[][1] = {-1000,0,100} minMaxIncr[][2] = {-5,10,.1} for (i=0;i<numSliders;i++) //option A duplicate/o/r=[][i] minMaxIncr,limitsWv;redimension/n=(-1) limitsWv slider $("slider_"+num2str(i)) limits=limitsWv //doesn't compile //option B slider $("slider_"+num2str(i)) limits=minMaxIncr[][i] //doesn't compile endfor end
I like this idea. In the meantime, why not use a general purpose function for both cases:
This change might also lend itself to this:
as a way to avoid the for-loop.
April 6, 2020 at 11:02 am - Permalink