
The last XOP you need - Introduction to the CallFunction XOP
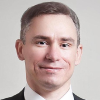
Directly calling external library functions
External libraries, so-called DLLs, can offer functionality not included
in Igor Pro, such as hardware device access. Igor Pro offers access to
DLLs with Igor Pro extensions (XOPs). However, there are hardware
devices where no XOP exists. Custom XOPs can be written, but this can
get quite involved; writing an own Igor Pro extension can significantly
increase development time. It can also be an obstacle for inexperienced
programmers.
With the introduction of our CallFunction-XOP, this problem is history.
The Call Function-XOP allows you to call functions from external shared
libraries directly from Igor code. Without the need to write any C/C++
code. Almost all third-party software, including drivers for devices,
provide their functionality through shared libraries (`.dll`) and bring
software development kits (SDK) that describe these functions in header
(`.h`) files. This information and the CallFunction-XOP are sufficient
to call these functions from Igor Pro. This approach is similar to other
meta-implementations like LabVIEW and allows the user to use these
external libraries without additional C programming knowledge.
For a straightforward example, let's assume we have the hardware vendor
library `device.dll` for a laser that offers the following function in
one of its header files:
/// @param power in milliwatts /// @return 0 on success, or a positive error code int SetLaserPower(double power);
Using this external function in Igor Pro is a four-step process. First, we have
to load the library, then call the function with our desired power value, and
retrieve the result. Finally, we unload the library.
The code with the CallFunction-XOP looks like this:
string libHandle variable jsonIDIn, jsonIDOut, power libHandle = FCALL_Load("device.dll") jsonIDIn = FCALL_SetupParameterIn("INT32;DOUBLE") power = 20.5 JSON_SetVariable(jsonIDIn, "/Parameter/0/value", power) jsonIDOut = FCALL_Call(libHandle, "SetLaserPower", jsonIDIn) printf "result: %d\r", JSON_GetVariable(jsonIDOut, "/result/value") FCALL_Free(libHandle)
First, we load the library from a given path on the file system and get a
reference to the loaded library. The next step is to set up the parameter of
the external function. From the definition in the header file, the function
returns an integer and takes a double as a parameter. With the utility function
`FCALL_SetupParameterIn` from the XOP, the function return type and the input
parameter types can be set up with a semicolon-separated list. The next two
lines set the value of the parameter. With this information, a call to the
external library can be executed, and we can retrieve the return value of the
function. At the end of our small program, we unload the library again.
The CallFunction XOP uses Javascript Object Notation (JSON) format as interface
language for making calls and retrieving information from the external libraries.
JSON strings can be created and parsed manually. It is, however, much more
convenient and less error-prone to handle them with the JSON-XOP.
In the example above, the functions prefixed with `JSON_` belong to this XOP.
This simple example shows how to use the CallFunction-XOP in general. The
parameters of external library functions can be any of the (C/C++) standard
data types. For array parameters, the XOP supports waves as well as inline
arrays. A JSON string -represented by its `jsonID` - is created during the
setup of the input parameters. Any further call to the same function can,
therefore, reuse this ID since the definition of the external function does not
change.
We demonstrated with this brief example that the CallFunction-XOP accelerates
the development of routines that access external devices using Igor Pro code
solely. The function calls are formulated in human-readable JSON formatted
strings and can be handled with the JSON-XOP.
The download package includes several real-world examples like calling
Microsoft Windows system functions, or controlling an Andor monochromator.
The screenshot shows a simple music player in Igor Pro that plays songs from a
playlist in the background.
In the music player example, all essential functions such as play/stop/pause/seek
are mapped to the functions of an external library. The FFT visualization exchanges
data in the form of an Igor Pro wave directly with the library.
The CallFunction-XOP currently supports Igor Pro 8 on Windows 7/10 (64 bit). In
case you are interested in a MacOSX version of this XOP, please drop us a line
at support@byte-physics.de.
The full documentation is available online, where a trial version of
the XOP can be downloaded as well.
Pricing information is available here.
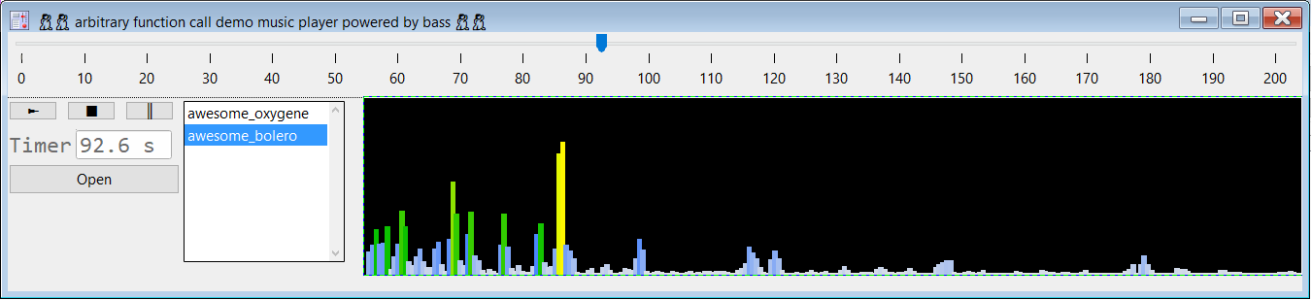