
Regular Expression Test Panel
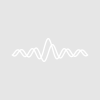
ikonen
Tue, 12/11/2012 - 10:17 pm
The conversion is primitive and only looks for doubled slashes ("\\") in the Igor version and single slashes in the interpreted version (I looked for a built in operation functions that could do this conversion, like when one enters text for an annotation and Igor builds a command with proper escape coding, but I couldn't find anything), but that should suffice for normal use. Apparently you can use the actual tab character in a regular expression to look for a tab character in a string, but I think the standard method is to use \t (which has to be entered as \\t in an Igor string literal in order to become \t in a PCRE in order to look for a tab character. As far as I know, it's only the need to double up slashes in Igor code string literals that causes the difference. Confusing? No doubt. That's why I wrote this snippet. It's also well explained in the Regular Expressions section of Programming.ihf
Note also that SetVariable controls don't convert escape strings: Entering \t in the PCRE field really does result in the slash and t characters being sent to the regular expression engine, not a tab character.
#pragma rtGlobals=1 // Use modern global access method.
Window Regexp_Panel() : Panel
PauseUpdate; Silent 1 // building window...
NewPanel /W=(50,66,634,411) as "Regular Expression Tester"
SetVariable setvar_teststring,pos={1,2},size={447,16},proc=SetVarProc_RegexpTester,title="Test String"
SetVariable setvar_teststring,value= _STR:"Thursday, May 7, 2009"
SetVariable setvar_PCREpattern,pos={3,28},size={444,16},proc=SetVarProc_RegexpTester,title="PCRE pattern"
SetVariable setvar_PCREpattern,value= _STR:"([[:alpha:]]+), ([[:alpha:]]+) ([[:digit:]]+), ([[:digit:]]+)"
SetVariable setvar_sub0,pos={17,81},size={209,16},title="sub0"
SetVariable setvar_sub0,value= _STR:"Thursday",noedit= 1
SetVariable setvar_sub1,pos={19,103},size={208,16},title="sub1"
SetVariable setvar_sub1,value= _STR:"May",noedit= 1
SetVariable setvar_sub2,pos={20,125},size={198,16},title="sub2"
SetVariable setvar_sub2,value= _STR:"7",noedit= 1
SetVariable setvar_sub3,pos={21,150},size={191,16},title="sub3"
SetVariable setvar_sub3,value= _STR:"2009",noedit= 1
SetVariable setvar_sub4,pos={20,172},size={192,16},title="sub4"
SetVariable setvar_sub4,value= _STR:"",noedit= 1
SetVariable setvar_sub5,pos={22,196},size={190,16},title="sub5"
SetVariable setvar_sub5,value= _STR:"",noedit= 1
SetVariable setvar_S_value,pos={11,226},size={406,16},title="S_Value"
SetVariable setvar_S_value,value= _STR:"Thursday, May 7, 2009",noedit= 1
SetVariable setvar_V_Flag,pos={17,255},size={88,16},title="V_Flag"
SetVariable setvar_V_Flag,value= _NUM:4,noedit= 1
CheckBox check_error,pos={11,284},size={134,14},title="Regular Expression Error"
CheckBox check_error,value= 0,side= 1
SetVariable setvar_IgorCodePattern,pos={5,51},size={441,16},proc=SetVarProc_RegexpTester,title="Igor /E pattern"
SetVariable setvar_IgorCodePattern,value= _STR:"([[:alpha:]]+), ([[:alpha:]]+) ([[:digit:]]+), ([[:digit:]]+)"
EndMacro
Function UpdateREPanel(panelname)
string panelname
ControlInfo /W=$panelname setvar_teststring
string teststring = S_Value
ControlInfo /W=$panelname setvar_PCREpattern
string regexp = S_Value
string sub0, sub1, sub2, sub3, sub4, sub5
try
SplitString /E=regexp teststring, sub0, sub1, sub2, sub3, sub4, sub5 ; AbortOnRTE
CheckBox check_error win=$panelName, value = 0
catch
CheckBox check_error win=$panelName, value = 1
endtry
SetVariable setvar_sub0 win=$panelName, value = _STR:(sub0)
SetVariable setvar_sub1 win=$panelName, value = _STR:(sub1)
SetVariable setvar_sub2 win=$panelName, value = _STR:(sub2)
SetVariable setvar_sub3 win=$panelName, value = _STR:(sub3)
SetVariable setvar_sub4 win=$panelName, value = _STR:(sub4)
SetVariable setvar_sub5 win=$panelName, value = _STR:(sub5)
SetVariable setvar_S_Value win=$panelName, value = _STR:(S_Value)
SetVariable setvar_V_Flag win=$panelName, value = _NUM:(V_Flag)
End
Function SetVarProc_RegexpTester(sva) : SetVariableControl
STRUCT WMSetVariableAction &sva
switch( sva.eventCode )
case 1: // mouse up
case 2: // Enter key
case 3: // Live update
strswitch(sva.ctrlName)
case "setvar_PCREpattern":
SetVariable setvar_IgorCodePattern win=$sva.win, value = _STR:(ReverseProcessIgorString(sva.sval))
break
case "setvar_IgorCodePattern":
SetVariable setvar_PCREpattern win=$sva.win, value = _STR:(ProcessIgorString(sva.sval))
break
case "setvar_teststring":
// print sva.sval
break
EndSwitch
UpdateREPanel(sva.win)
break
case -1: // control being killed
break
endswitch
return 0
End
Function /S ReverseProcessIgorString(stringin)
string stringin
string stringout = ReplaceString("\\",stringin,"\\\\")
return stringout
End
Function /S ProcessIgorString(stringin)
string stringin
string stringout = ReplaceString("\\\\",stringin,"\\")
return stringout
End
Window Regexp_Panel() : Panel
PauseUpdate; Silent 1 // building window...
NewPanel /W=(50,66,634,411) as "Regular Expression Tester"
SetVariable setvar_teststring,pos={1,2},size={447,16},proc=SetVarProc_RegexpTester,title="Test String"
SetVariable setvar_teststring,value= _STR:"Thursday, May 7, 2009"
SetVariable setvar_PCREpattern,pos={3,28},size={444,16},proc=SetVarProc_RegexpTester,title="PCRE pattern"
SetVariable setvar_PCREpattern,value= _STR:"([[:alpha:]]+), ([[:alpha:]]+) ([[:digit:]]+), ([[:digit:]]+)"
SetVariable setvar_sub0,pos={17,81},size={209,16},title="sub0"
SetVariable setvar_sub0,value= _STR:"Thursday",noedit= 1
SetVariable setvar_sub1,pos={19,103},size={208,16},title="sub1"
SetVariable setvar_sub1,value= _STR:"May",noedit= 1
SetVariable setvar_sub2,pos={20,125},size={198,16},title="sub2"
SetVariable setvar_sub2,value= _STR:"7",noedit= 1
SetVariable setvar_sub3,pos={21,150},size={191,16},title="sub3"
SetVariable setvar_sub3,value= _STR:"2009",noedit= 1
SetVariable setvar_sub4,pos={20,172},size={192,16},title="sub4"
SetVariable setvar_sub4,value= _STR:"",noedit= 1
SetVariable setvar_sub5,pos={22,196},size={190,16},title="sub5"
SetVariable setvar_sub5,value= _STR:"",noedit= 1
SetVariable setvar_S_value,pos={11,226},size={406,16},title="S_Value"
SetVariable setvar_S_value,value= _STR:"Thursday, May 7, 2009",noedit= 1
SetVariable setvar_V_Flag,pos={17,255},size={88,16},title="V_Flag"
SetVariable setvar_V_Flag,value= _NUM:4,noedit= 1
CheckBox check_error,pos={11,284},size={134,14},title="Regular Expression Error"
CheckBox check_error,value= 0,side= 1
SetVariable setvar_IgorCodePattern,pos={5,51},size={441,16},proc=SetVarProc_RegexpTester,title="Igor /E pattern"
SetVariable setvar_IgorCodePattern,value= _STR:"([[:alpha:]]+), ([[:alpha:]]+) ([[:digit:]]+), ([[:digit:]]+)"
EndMacro
Function UpdateREPanel(panelname)
string panelname
ControlInfo /W=$panelname setvar_teststring
string teststring = S_Value
ControlInfo /W=$panelname setvar_PCREpattern
string regexp = S_Value
string sub0, sub1, sub2, sub3, sub4, sub5
try
SplitString /E=regexp teststring, sub0, sub1, sub2, sub3, sub4, sub5 ; AbortOnRTE
CheckBox check_error win=$panelName, value = 0
catch
CheckBox check_error win=$panelName, value = 1
endtry
SetVariable setvar_sub0 win=$panelName, value = _STR:(sub0)
SetVariable setvar_sub1 win=$panelName, value = _STR:(sub1)
SetVariable setvar_sub2 win=$panelName, value = _STR:(sub2)
SetVariable setvar_sub3 win=$panelName, value = _STR:(sub3)
SetVariable setvar_sub4 win=$panelName, value = _STR:(sub4)
SetVariable setvar_sub5 win=$panelName, value = _STR:(sub5)
SetVariable setvar_S_Value win=$panelName, value = _STR:(S_Value)
SetVariable setvar_V_Flag win=$panelName, value = _NUM:(V_Flag)
End
Function SetVarProc_RegexpTester(sva) : SetVariableControl
STRUCT WMSetVariableAction &sva
switch( sva.eventCode )
case 1: // mouse up
case 2: // Enter key
case 3: // Live update
strswitch(sva.ctrlName)
case "setvar_PCREpattern":
SetVariable setvar_IgorCodePattern win=$sva.win, value = _STR:(ReverseProcessIgorString(sva.sval))
break
case "setvar_IgorCodePattern":
SetVariable setvar_PCREpattern win=$sva.win, value = _STR:(ProcessIgorString(sva.sval))
break
case "setvar_teststring":
// print sva.sval
break
EndSwitch
UpdateREPanel(sva.win)
break
case -1: // control being killed
break
endswitch
return 0
End
Function /S ReverseProcessIgorString(stringin)
string stringin
string stringout = ReplaceString("\\",stringin,"\\\\")
return stringout
End
Function /S ProcessIgorString(stringin)
string stringin
string stringout = ReplaceString("\\\\",stringin,"\\")
return stringout
End
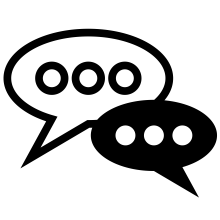
Forum

Support

Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More