
Delete all NaN (empty points) from a numeric wave or a text wave
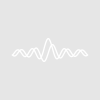
PeterR
// Handy Function to delete NaNs from TextWaves: Function DeleteNaNTextwave(W) Wave /T W Duplicate /T /O W WwoNaNs Variable Points = numpnts(WwoNaNs) InsertPoints Points,1, WwoNaNs // Insert trailing NaN to make loop-break-condition work Variable i=0, NOP Do NOP = numpnts(WwoNaNs) if((strlen(WwoNaNs [i]) == 0)||(cmpstr("",WwoNaNs [i]) == 0)||(cmpstr("NaN",WwoNaNs [i])==0)) DeletePoints i, 1, WwoNaNs i = 0 // Start over again else i = i+1 endif while(strlen(WwoNaNs[NOP])==0) // see above End // Handy Function to delete NaNs from NumericWaves: Function DeleteNaNNumWave(W) Wave W String OldName = NameofWave(W) String NewName = OldName+"_woNaNs" ConvertNumWvToTxtWv(W) DeleteNaNTextwave(TxtConvert) ConvertTxtWvToNumWv(WwoNaNs) Duplicate /O NumConvert $NewName End // Handy Function to convert numeric waves into text waves Function ConvertNumWvToTxtWv(W) Wave W Variable np = numpnts(W) Make /T /O /N=(np) TxtConvert Variable x for(x=0;x<np;x+=1) TxtConvert[x] = num2str(W[x]) endfor End // Handy Function to convert text waves into numeric waves Function ConvertTxtWvToNumWv(W) Wave /T W Variable np = numpnts(W) Make /O /N=(np) NumConvert Variable x for(x=0;x<np;x+=1) NumConvert[x] = str2num(W[x]) endfor End
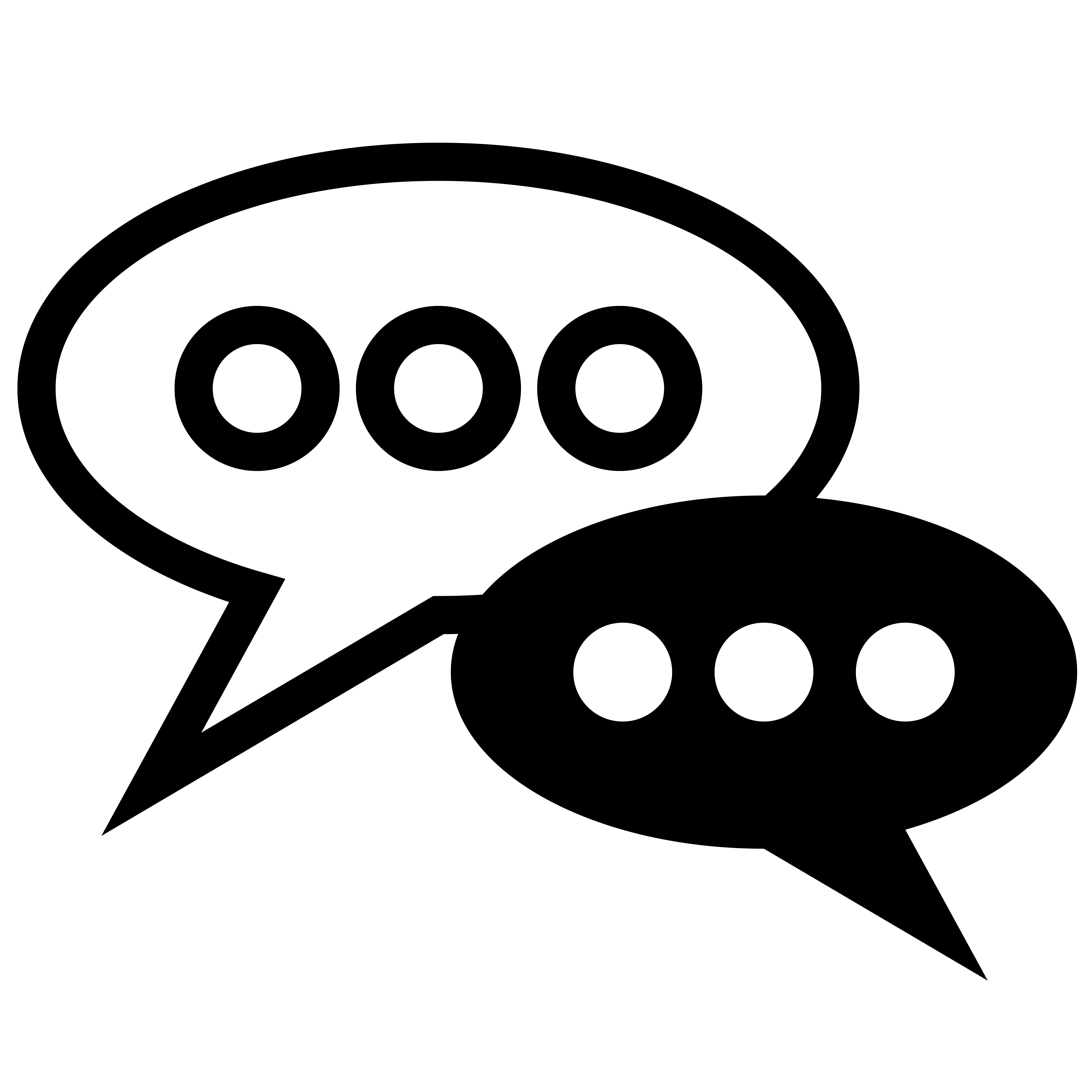
Forum
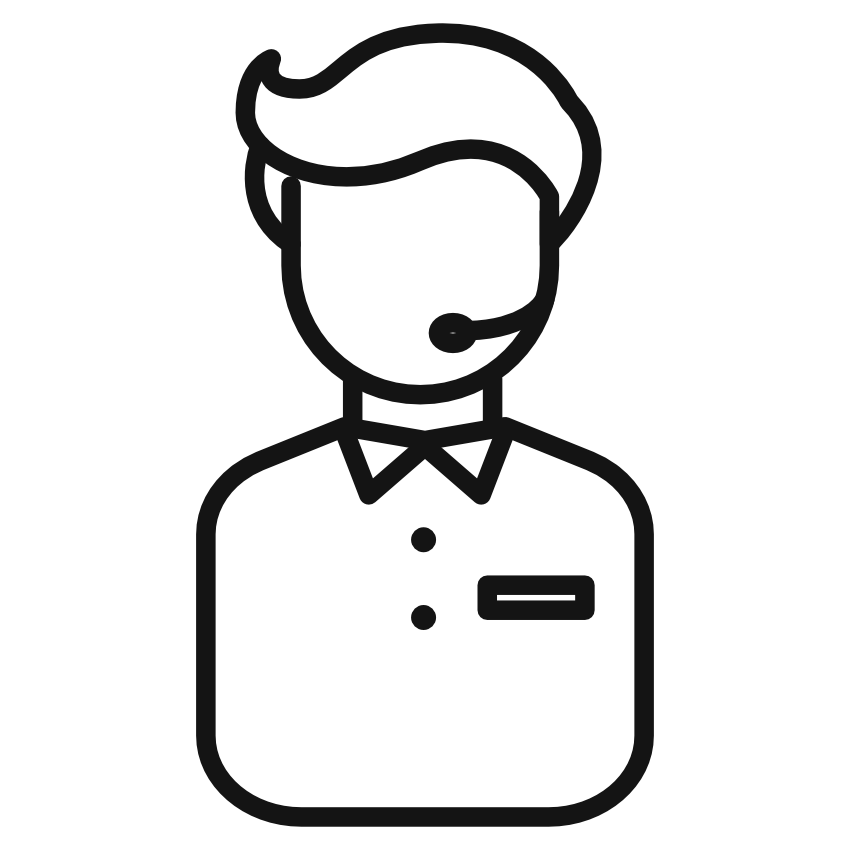
Support
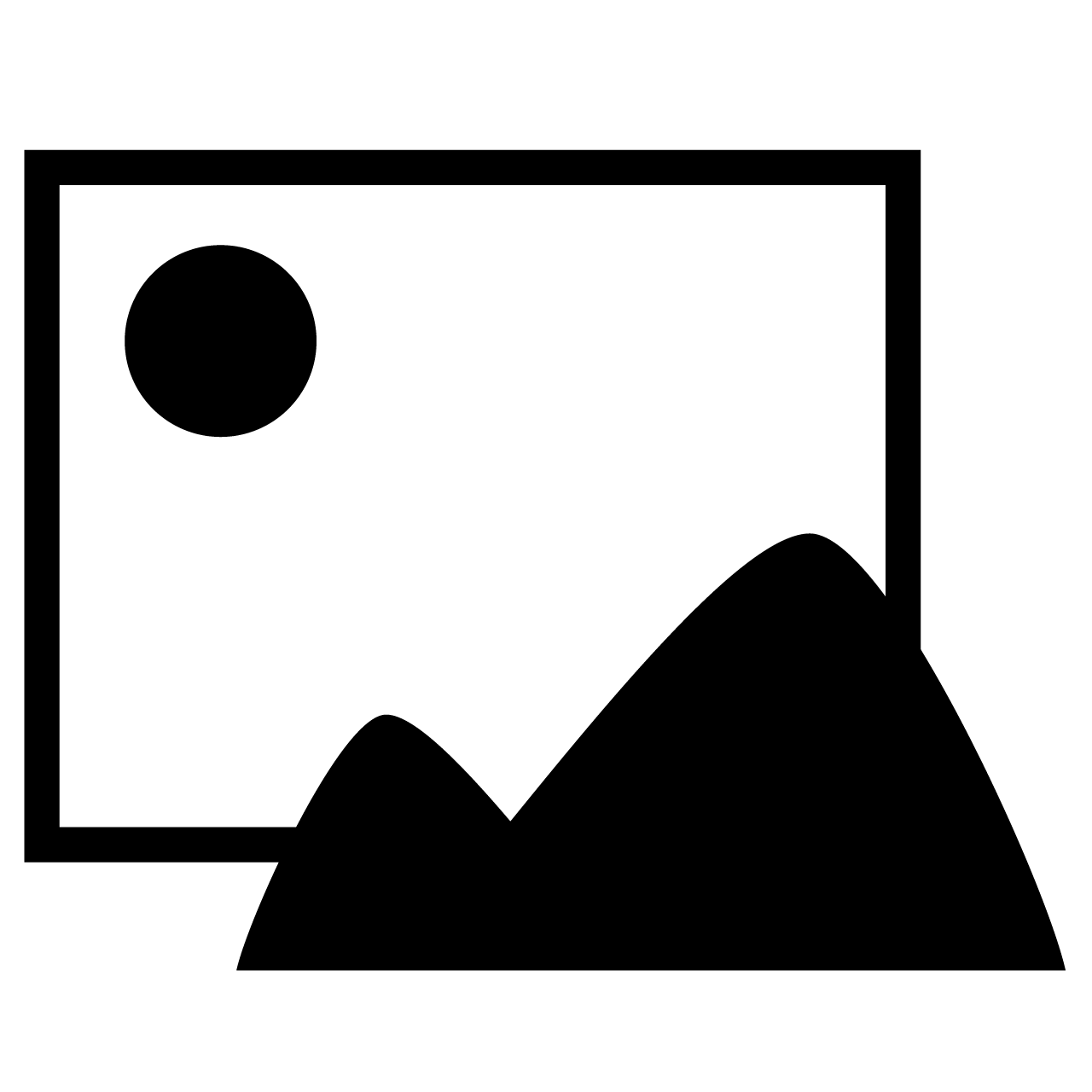
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
WaveTransform zapNaNs
for a builtin way.August 28, 2014 at 04:13 am - Permalink
You don't need to "start over again" after every point that you've deleted. You could simply call continue:
Note that this code gives a run time error if
#pragma rtGlobals=3
is in effect.I would recommend writing the function a slightly different way. This iterates in reverse using a For loop and it doesn't call InsertPoints at the beginning. The fewer inserting and deleting of points you do to a text wave, the better off you are, because redimensioning a text wave like that can be slow if the wave is large.
By the way, I don't understand why you have both
(strlen(WwoNaNs [i]) == 0)
and(cmpstr("",WwoNaNs [i]) == 0)
in your test. I can't think of a situation in which the second would be true but the first would be false.August 28, 2014 at 06:19 am - Permalink
Extract /o W, WwoNaNs, numtype(wtest)==0
Is WaveTransform more efficient?
September 13, 2014 at 05:54 pm - Permalink