
Get coordinated of user drawn rectangle in image
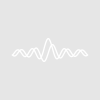
mouthbag
I have a seemingly simple problem, however I could not find a solution in the Igor documentation.
I have an image that is displayed vie NewImage. The user has to draw a rectangular ROI in the image. This can be easily done by utilizing the standard ROI-Panel. What I need is a way to get the coordinates of the user drawn rectangle. Either in pixels or some other arbitrary scaling.
Is there a way to get the rectangle coordinates inside the image?
Best regards,
Todor
ImageGenerateROIMask
. I believe it creates a 2D wave with pixels in the ROI set to one value and pixels outside set to another. When I have used it, I recall at first failing to read the second paragraph in its help file, which mentions that the ROI has to be drawn in the drawing layer 'progFront'. A way to set an image window to that drawing layer is to run:setdrawlayer progFront
If you're only interested in rectangular ROIs, it looks like
getmarquee
andsetmarquee
are a second option, as mentioned here http://www.igorexchange.com/node/8215March 9, 2018 at 05:45 am - Permalink
Take a look at
GetMarquee
in the help browser and click on the "Open Marquee Demo" link at the bottom. This provides a nice intro to how the marquee may simplify your application.March 9, 2018 at 06:26 am - Permalink
I had to solve this problem not long ago. Look at this post for the code I used using marquee.
http://www.igorexchange.com/node/8215
Andy
March 9, 2018 at 06:43 am - Permalink
March 9, 2018 at 06:46 am - Permalink
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAH
March 9, 2018 at 04:38 pm - Permalink
During the last few days my objective changed. I want the user to be able to select multiple rectangular ROIs and extract the position of their top left corner as well as height and width.
Here is the code which works for me:
There might be some syntax errors, scince I truncated the code a litte. There are a few extra steps and precautions needed in my application.
Best regards,
mouthbag
March 13, 2018 at 08:40 am - Permalink