
Add date to time wave
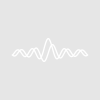
zephyr070
I have collected temperature data with a time stamp that only gives HH:MM:SS, so running the experiment overnight causes the data to loop at 11:59:59. I would like to append dates (4/16/2019 and 4/17/2019) to the appropriate range of points in the wave so that I end up with: month/date/year HH:MM:SS. Any help is much appreciated. Thank you!
-Kevin
Igor stores date/time values as the number of seconds since 1904-01-01.
You can get this number for a given date using the Date2Secs function.
If, for example, your wave consisted of time-of-day (in seconds since midnight) for 2019-04-16, you could add the date part to the time part like this:
Before you do that, make sure that the wave is double precision and has data units "dat". Execute this for details:
DisplayHelpTopic "Date/Time Waves"
April 17, 2019 at 01:20 pm - Permalink
hrodstein's solution will make every repeated day range have the same date. Here is a function that will take your cycled time-of-day data and add the appropriate date to each day:
April 17, 2019 at 01:30 pm - Permalink
Here's a slightly more efficient version:
April 17, 2019 at 01:34 pm - Permalink
Thank you both, that solved my problem!
April 17, 2019 at 02:12 pm - Permalink