
Location and font of textbox when using FuncFit
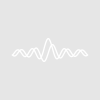
Physicist92
Hi all,
I use FuncFit to do fitting with user-defined function for a set of data. I'd like to place textbox (textbox contains found fitting parameters and their errors) in each graph in specific location. I did the following:
FuncFit/Q/TBOX=768 myFunc W_coef w /D TextBox/A=LT
But the command TextBox/A=LT doesn't change the location of textbox created using FuncFit. Is there any way to place textbox in specific location in graphs in code (not manual drag and drop)? And I'd like to change font size of text in textbox, is it possible?
I found how to change location of textbox:
TextBox/C/N=CF_wavename/X=0.00/Y=0.00
But I don't understand how to change font size without writing text of textbox. I found how to change font size with writing text:
TextBox/C/N=CF_wavename "\\Z08Coefficient values ± one standard deviation\r\ta\t=4.9649 ± 0.301\r\tb\t=10.744 ± 0.738"
It is possible if I change font size manually for some data, but I'd like to do it in code for large data set, and given that I don't know what text will be in textbox for each case. Please advise how to solve this problem.
April 23, 2019 at 10:52 am - Permalink
Use the AnnotationInfo() function. The text of the annotation is contained in the TEXT keyword, but since the text itself could contain separator characters, it can be hard to unpack. We provide the Annotation Procs.ipf that has utility functions for dealing with the output of AnnotationInfo:
#include <AnnotationInfo Procs>
Especially, see the AnnotationText() function provided by that procedure file.
April 23, 2019 at 11:59 am - Permalink
In reply to Use the AnnotationInfo()… by johnweeks
Thanks a lot!
April 23, 2019 at 01:02 pm - Permalink
I wrote test function to generate data:
Then I created fitting function:
Finally, I wrote function for fitting:
But the last line
TextBox/C/N=name_tbox/X=0.00/Y=0.00
doesn't do anything. I don't understand why it doesn't change location of textbox in graphs. Could you please tell me, how should I change code to place textbox in specific location?
April 24, 2019 at 01:05 pm - Permalink
You may need to change the anchor point of the textbox. If you want it in the upper-left corner of your graph, use /A=LT.
April 24, 2019 at 01:11 pm - Permalink
In reply to You may need to change the… by johnweeks
I set /A=LT, but the result is the same. Please find attached the experiment file with graphs.
April 24, 2019 at 01:20 pm - Permalink
You need to add a $ operator to your Textbox command:
TextBox/A=LT/C/N=$name_tbox
The clue for me was the tiny, blank textbox in the upper left corner of your graph. Those tiny textboxes all have the name "name_tbox". For more on the $ operator: DisplayHelpTopic "Converting a String into a Reference Using $" That description is mostly about waves, but this works pretty much anywhere our documentation says something is a "name" rather than a string containing a name.
April 24, 2019 at 01:35 pm - Permalink
In reply to You need to add a $ operator… by johnweeks
Thank you very much! Now it works!
Previously I tried to use /N=$name_tbox/X=0.00/Y=0.00, but without /A=LT. I thought that if I use /X=0.00/Y=0.00, then I don't need to use /A=LT.
April 24, 2019 at 01:44 pm - Permalink
The /X and /Y positions are relative to the anchor point specified via /A.
April 24, 2019 at 03:32 pm - Permalink
In reply to The /X and /Y positions are… by johnweeks
I read now Igor Pro help regarding textbox /X option more attentively: "xOffset is the distance from anchor to textbox". Previously I didn't notice this.
April 24, 2019 at 03:54 pm - Permalink