
automating sorting
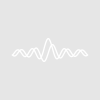
Amo
Greetings forum,
I have a list R00 and want to sort its values. R00 contains positions that are spaced on average 10.
For example: values between 8 to 11 are stored in wave R1, values between 11 to 20 are stored in wave R2 , values between 20 to 30 are stored in wave R3 and so on,
Right now I manually sort for each interval as following:
#pragma rtGlobals=1 // Use modern global access method. function Bin_v2() Wave R00 Duplicate R00, copy MatrixOP/O index = col(copy,0) index = index[p] > 8 && index[p] < 11 ? p : NaN Wavetransform zapNaNs index Make/O/N=(numpnts(index)) R1 = copy[index[p]] MatrixOP/O index2 = col(copy,0) index2 = index2[p] > 11 && index2[p] < 20 ? p : NaN Wavetransform zapNaNs index2 Make/O/N=(numpnts(index2)) R2 = copy[index2[p]] MatrixOP/O index3 = col(copy,0) index3 = index3[p] > 20 && index3[p] < 30 ? p : NaN Wavetransform zapNaNs index3 Make/O/N=(numpnts(index3)) R3 = copy[index3[p]] MatrixOP/O index4 = col(copy,0) index4 = index4[p] >30 && index4[p] <40 ? p : NaN Wavetransform zapNaNs index4 Make/O/N=(numpnts(index4)) R4 = copy[index4[p]] MatrixOP/O index5 = col(copy,0) index5 = index5[p] >40 && index5[p] <50 ? p : NaN Wavetransform zapNaNs index5 Make/O/N=(numpnts(index5)) R5 = copy[index5[p]] MatrixOP/O index6 = col(copy,0) index6 = index6[p] >50 && index6[p] <60 ? p : NaN Wavetransform zapNaNs index6 Make/O/N=(numpnts(index6)) R6 = copy[index6[p]] end
can you suggest a possible loop that could do this?
Thank you
Without looking at your code in detail, this looks like a situation where running the built-in Extract procedure could do the trick, possibly followed by calling Sort on each of the resultant waves R1,R2,..
If the original wave is multi-dimensional, you might want to use Extract's /indx flag and then write your own code to deal with the columns
September 30, 2019 at 11:25 am - Permalink
In reply to Without looking at your code… by gsb
Thanks for reply,
Lets assume it's one dimensional (not multi). I changed my code and all it does just sorts into intervals. Now I don't wanna manually do it for each R, is there a way to do a loop?
September 30, 2019 at 11:38 am - Permalink
Try something like:
In the event that R00 is multi-dimensional you can modify the last make command, e.g.,
Make/O/N=(numpnts(index1),numCols) $name=R00[index1[p]][q]
I hope this helps,
AG
September 30, 2019 at 01:16 pm - Permalink
In reply to Try something like: … by Igor
Is replace , greater and indexRows are waves? because right now i get syntax error (indexRows) , if i compile it as it is.
If they are wave and i make them separately , I get error: Nans are not allowed in this operation line 11
September 30, 2019 at 02:10 pm - Permalink
The code is for IP 8.x where Replace() greater() etc are MatrixOP functions.
September 30, 2019 at 02:12 pm - Permalink
In reply to The code is for IP 8.x where… by Igor
Ok , I have igor 6.10...
what is the alternative for earlier versions
September 30, 2019 at 02:41 pm - Permalink
IP6 is way too old.
Obviously you can rewrite
MatrixOP/O index1=replace((greater(index,v1)*greater(v2,index))*indexRows(index),0,NaN)
In IP6 code using code similar to:
September 30, 2019 at 03:20 pm - Permalink
In reply to IP6 is way too old. … by Igor
thanks a lot
September 30, 2019 at 05:17 pm - Permalink