
Manipulating and combining existing graphs programatically
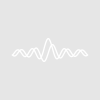
Hi,
I often have a few data folders (DFs) where I'd like to view the contents -- as graphs. So, I'm currently trying to produce a Panel where I can click through different views in order to visualize the DFs.
I'm using a code package which already works fine to produce various graphs. So, I'm just looking to make a "wrapper" around pre-existing graph-producing functions.
My strategy has been to:
- Execute() the functions and macros which make graphs
- Use WinRecreation() to capture the recreation macros for the resulting graphs, and reproduce them inside a panel
- Kill the original graphs
This leads to some issues and confusion with wave paths in the WinRecreation result (which are sometimes relative and sometimes absolute) and also with ControlBar (I think I will need to remove all ControlBar commands for this to really work... or somehow programatically shift down all controls that would have been inside the ControlBar).
I'm starting to think I should take an alternative approach:
- Use NewPanel /EXT to produce an attached panel, so on any given graph I can jump to another graph.
- Just produce a lot of graphs and use the WindowBrowser to only show graphs for a given DF. Unfortunately, the WindowBrowser doesn't allow filtering by DF, only by wave. But I could use a keyword prefix.
- Just produce one reliable "mega graph" and then use ReplaceWave allincdf
With this post, I'm hoping for any insights from those who have more experience programming UIs in Igor. Thanks in advance, j
I struggled with something akin to this in Image Tools. I need to store images for contact prints. I scroll through the images in a single window, so they do not remain "resident" for some later recreation.
I ended up storing the WinReaction strings for the image graph in a global folder (root:Packages:Image Tools: ...). Each WinReaction string has a name that is characteristic of the image that was stored in the graph at the time. It helps to check back and show the user when that image has already been set as a "contact print image".
I know that I wonder about a "more elegant" way to handle my needs as well. But, necessity was a push, and my approach seems to work now.
Also, as far as I recall, I had some way that I used to remove the control bar portion of the WinReaction. I can dig back for the specifics as needed. In the meantime, you are welcomed to look at the code in the Image Tools Print.ipf procedure file for further insights. Ask back here for specifics as needed.
April 29, 2020 at 02:27 pm - Permalink
Thanks for pointing me to that. I did not really notice any use of ControlBar except for a few buttons.
I think the only way to guarantee elegance in both cases is for the package to only manage graphs it produces.
The fundamental problem for me is that Igor contains certain "hacks" like the ControlBar, which let users blur the line between Graphs and Panels. The ways I see to handle ControlBars are:
April 30, 2020 at 08:44 am - Permalink
Odd. Somehow between the previous version and the current version I've misplaced what happens to the control bar.
You are right about this. A Control Bar will get in the way to create a clean graph copy. I also cannot see a clean way to program around the bells and whistles that come with a control bar, e.g. via regex eliminations from the WinReaction.
I will also say that, when I run an Execute/Q/Z using the WinReaction string that contains a ControlBar commands, I do not get the ControlBar. I am not sure why (I have to dig deeper on what my as-yet-kludged-together code is really doing at that point).
April 30, 2020 at 09:36 am - Permalink
I'm not sure exactly what you want....
But what I often do is browse through XPS spectra, for instance. I do that by creating a graph of an empty wave that I would call MyDisplayedWave, for instance. I also create two popup menus: one listing all datafolders and the other listing all the waves of the desired type and dimensionality in the in folder selected in the first pop up menu. Selecting a wave in the 2nd popup menu now duplicates the selected wave into MyDisplayedWave, which will display the data. I found that much easier and less prone to bugs than adding and removing waves from the graph.
Is it something like that you want?
If you want to remove buttons and controlbars from a windows recreation string have a look at Grep. Below is an example I use to create clean copies of graphs
April 30, 2020 at 10:33 am - Permalink
@olelytken Thanks, I think you are right that this is the most painless strategy.
May 11, 2020 at 11:00 pm - Permalink
I tried to reduce the functions I use to a minimal working example. Run CreateWindow to see how it works
May 12, 2020 at 04:47 am - Permalink