
Making a number of graphs
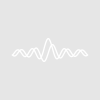
meraculin
Hi, I am new to Igor.
I want to make a number of simple time vs displacement graphs automatically, but I am still unfamiliar with the programming. I have tried reading several forum threads but I still don't get how to do it.
All the graphs has the same x-axis time, from the table -> wave0. Then, wave1, wave2, ... are the displacement on y-axis.
I want to make many graphs with Graph 1 -> wave0 vs wave 1, Graph 2-> wave0 vs wave2, and so on.
Thanks!
August 23, 2022 at 10:12 pm - Permalink
Your function call would look like
MakeManyGraphs(wave0, "wave1;wave2;wave3")
To understand what's happening above execute
DisplayHelpTopic "Converting a String into a Reference Using $"
But if you are new to Igor, you would benefit from doing the Guided Tour.
August 23, 2022 at 11:15 pm - Permalink
In reply to if you have many waves and… by ChrLie
Thank you so much! Your code works like magic.
Also, I didn't realize that I needed to write the function in the procedure tab, I was writing my code on the command window and was wondering why it didn't work even though it made sense.
I modified your code so that I don't need to write long list of waves.
Is there any other way to count the number of columns on a table? I had already made the table but I need to put the number of columns manually.
August 24, 2022 at 01:35 am - Permalink
It seems like you would benefit very much from reading / doing the Guided Tour of Igor. To open the tour run the following command in the command line:
DisplayHelpTopic "Guided Tour of Igor Pro"
Regarding your question, it depends. Your function only works because you inserted all your data in a table, and this automatically creates standard data 'waves', with default names wave0, wave1, etc. However, in general the waves have proper names. If you only ever want to work the way you do now, and simply plot columns of a table with default names, then the following code plots all columns without the need to entering a number:
August 24, 2022 at 02:20 am - Permalink
I'll make sure to do the Guided Tour. Thank you so much!
August 24, 2022 at 02:55 am - Permalink