
Printing multiple Layouts in a go
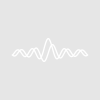
CJanssen
Before I struggle too long, I thought I would ask. I found an old thread on the igor mailing list which was on a related topic, but it was concerned with printing of graphs. The command "PrintGraphs" accepts several graphs to be printed at a time, but the corresponding "PrintLayout" command does seem to come only in the singular version.
To me it seems that the standard PrintGraphs/PrintLayout commands do print things just on one page, but do not allow to print a series of Layouts/Graphs on several pages. Am I correct there?
As a workaround, I was thinking I might need to export the layouts (as pdfs, for example), combine them to one file and then print from the shell.
Christof Janssen
To print all layouts, just copy the function above into a procedure window in your experiment and execute the following at the command line:
To print a list of specific layouts, copy the function into your experiment and execute the following at the command line:
If you wanted to get really fancy you could create a control panel with all layouts in the experiment and provide a ListBox control that the user could use to check off which layouts should be printed. You could look at my CreateLayouts project at http://www.igorexchange.com/project/ACL_CreateLayouts to get an idea of how this might work.
[Edit: I've created a code snippet for this at http://www.igorexchange.com/node/513 ]
February 28, 2008 at 06:25 am - Permalink
Thanks, this is marvellous! It works just how I wished it would (and its a much nicer code than that which I would have come up myself). I will probably go the route of adding a simple Listbox to allow for easy (just very basic) selection of the layouts. At the moment I do not have the time for a more sophisticated GUI, but maybe I will improve later using your CreateLayout project as inspiration.
I have a completely unrelated technical question. When I copied your code into my procedure window, the compiler choked on an invisible sign after one of the endifs. It took me a while to find it, however. Is there any reason why there should be such 'hidden' signs?
February 29, 2008 at 02:14 am - Permalink
I'm not sure what you are talking about. As far as I can tell, the only thing after both endifs is a line break, followed by a space and another line break.
What browser and what OS are you using? Perhaps there's something strange about how different browsers copy and paste the HTML code. I'm using FireFox 2 on WinXP, for what that's worth.
February 29, 2008 at 07:58 am - Permalink
I am using Safari 3.0.4 on Mac OS X (10.4.11). So it might be a line ending issue. I reproduced the behaviour. The error is always on the first sign in the empty lines of your code, ie the newline after the two endifs and after the declaration "string layoutlist". The igor compiler highlights this empty sign by a blueish box and says : "expected a keyword or object name". After deleting these signs, the procedure compiles just fine.
February 29, 2008 at 10:48 am - Permalink
I have submitted a bug report to WaveMetrics about this, though I'm not certain that Igor is to blame. For now, you can workaround this problem either by just deleting those three lines or replacing them with an actual space character, or you can use another browser (Firefox 2 on the Mac is fine) or use another operating system (using IE, FF, or Safari on WinXP or Vista does not result in this problem).
March 1, 2008 at 08:01 am - Permalink
The advantage of using this code is that I don't have to deal with a print dialogue each time. The disadvantage is that I can't set the print instructions, e.g. two per page or save as PDF instead of printing. I'm using a Mac.
June 2, 2008 at 04:58 am - Permalink
Check out the PrintSettings operation.
June 3, 2008 at 06:34 pm - Permalink