
Creating new 2D wave from multiple existing wave data
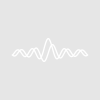
franc.23
Hello,
I am quite new with Igor and somehow between beginner and intermediate level in coding/programming and need some help and suggestions.
I currently deal with mass spectrometry data of five different chemical species/components: NH4, NO3, SO4, Chl, and Org. It's a 2D wave, with +8000 rows representing time and 97 columns representing ion mass. I want to sum the data of each species in the corresponding time and mass in a new 2D wave. Here is what I code for the function:
Function SumAllSpecies() int rownum,colnum //Row number and column number int i,j //Index Wave NH4_data, Chl_data, NO3_data, SO4_data, Org_data //Data waves rownum=DimSize(NH4_data, 0) colnum=DimSize(NH4_data, 1) Make /O/N=(rownum,colnum) AllSpecies_data for(i=0;i<rownum;i=i+1) for(j=0;j<colnum;j=j+1) AllSpecies_data[i][j] = NH4_data[i][j]+Chl_data[i][j]+NO3_data[i][j]+SO4_data[i][j]+Org_data[i][j] endfor endfor end
However, it doesn't do its job properly. Has anyone ever done something similar to this (not necessarily mass spectrometry data). Do you have any suggestions? Thank you very much!
Welcome to the forum. It is unclear what does not work properly for you. What should the result look like? Currently your code just sums up each respective point of all five samples. This disregards the (possibly present) time and ion mass scaling and also assumes that all data sets have exactly the same number of points. Do you want to sum the data for each (scaled) time and mass value? Then you would need to consider the x and y scaling, which might be different for each data set. But it is difficult to suggest something without knowing how your data looks exactly. Would it be possible to post a small example experiment file with a description of what you want to achieve? We could easily suggest small code then.
January 26, 2022 at 01:44 am - Permalink
In reply to Welcome to the forum. It is… by chozo
Hello, thank you very much for the reply!
Each species wave contain the time data as row (which is the same across all species because they are collected from the same instrument at the same time) - no need to scale - and m/z values from 0 to 97 as column. The values are the signal obtained from each m/z and time of data acquisition on the instrument.
I am actually going to use "AllSpecies_data" to perform PMF analysis in Igor instead of doing each species separately, to see whether the combination of all species detected in each m/z would result in different profile or make sense.
January 26, 2022 at 02:00 am - Permalink
OK, then summing the data like this should give you the sum of all data sets (actually including the time row). Is this then not what you want? If you want the average values then you should divide by 5 at the end. I am not well-versed in mass spectroscopy, so I do not know what a PMF analysis is. We can help you with the task if you can formulate how you want the data to turn out (again, having some example data would be much easier for everybody to test how things go).
By the way, just a small comment on your code: You do not need to have the for-loops since Igor is doing this for you automatically during wave assignment. Also, since all data sets seem to be the same size you can just write (even in the command line without the need for a function):
January 26, 2022 at 02:12 am - Permalink
In reply to OK, then summing the data… by chozo
Thank you for the suggestion! I am looking for the sum not the average.
The final graph looks like this but now since you mentioned about the time/date, I am convinced that the procedure messes up with the date value/format.
January 26, 2022 at 04:44 am - Permalink
If you want to combine a bunch of 1D waves to create a 2D wave, you can use Concatenate:
Concatenate {NH4_data, Chl_data, NO3_data, SO4_data, Org_data}, AllSpecies_data
Execute
DisplayHelpTopic "concatenate"
for details
January 26, 2022 at 04:48 am - Permalink
Yes, I guess if you use the time row from the summed data for the plot this will not work. Either use the time row from one of the original data waves or have the time as a separate (x) wave in the first place. If you divide the time row by 5 after summing it might work, but I would not count on that. By the way, I have no idea how the plot is supposed to look, so I am a bit lost on what is wrong here.
January 26, 2022 at 04:49 am - Permalink
using duplicate instead of make will preserve any scaling (for time, mass) in your 2D input waves
January 26, 2022 at 04:56 am - Permalink
In reply to Duplicate/O NH4_data,… by tony
Thank you very much everyone for your help and suggestions! I will look everything up again :)
January 26, 2022 at 05:03 am - Permalink
In reply to If you want to combine a… by tony
I believe the input waves are actually 2D (see DimSize definition of AllSpecies_Data above). In this case you could use
Concatenate/O/NP=2 {NH4_data, Chl_data, NO3_data, SO4_data, Org_data}, DataCube
to make a 3D wave, then apply MatrixOP to obtain the same result:
MatrixOP/O AllSpecies_Data = sumBeams(datacube)
Plenty of options ;-)
January 26, 2022 at 06:20 am - Permalink