
Exporting multiple 2D waves
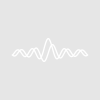
mssmtrainee
Basically, I'd like to export N-2D waves of an experiment into N-text files. Of course, I can just open the wave table, copy the 2 columns and past into a text file, but it is getting intense when I have few hundreds of waves.
I did some coding in the past, but I am totally new to Igor, so any help and advice will be much appreciated. Thanks!
But first . . .
If you are not familiar with the important concept of Igor symbolic paths, execute this and read the corresponding help:
If you are not familiar with the important concept of Igor data folders, execute this and read the corresponding help:
Also, if you have not already done it, do the first half of the Igor Guided Tour which you can find by choosing Help->Getting Started.
Here is the routine:
November 30, 2011 at 07:12 pm - Permalink
First, I'd like to name my text files 1.dat, 2.dat, 3.dat etc. Is there away to convert an Integer into a String? (7th line from the end)
And second, to make the code work, I need to load my waves. Is there a way to load, let say 50 waves in the same time?
December 2, 2011 at 03:26 pm - Permalink
Sure, it's
num2istr
.Maybe you can start by modifying this function posted by Adam Light.
A
December 3, 2011 at 03:02 am - Permalink
Execute this:
December 3, 2011 at 06:06 am - Permalink