
global variable, prompt, dependency
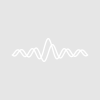
Simon87
Wed, 02/15/2012 - 08:27 am
My problem is the following. I would like to establish a dependency of a wave on a variable that I created using the doprompt operation :
Function bibi()
Variable j, m
Prompt j, "Enter j"
Prompt m, "Enter m"
Doprompt "Parameters", j, m
Make/D/O/N=10 theWave; edit theWave
theWave:=p*j+m
End
But I get this error message : "Can't use local variables in dependency formula".
So I changed the function as following :
Function bibi()
Variable/G j, m
Prompt j, "Enter j"
Prompt m, "Enter m"
Doprompt "Parameters", j, m
Make/D/O/N=10 theWave; edit theWave
theWave:=p*j+m
End
But now, the error message is : "Can't prompt for this type of variable".
So, my aim is to establish a wave dependency on a variable (so that I would only need to change the variable instead of using the entire macro/function to change the wave). Is there a trick to make a variable (created by prompt/doprompt) global ?
variable/G tg, kg
theWave := jg*p + kg
display theWave
Then, you can cause changes by running the viaPrompt function.
NVAR jg, kg
wave theWave = root:theWave
variable j, k
Prompt j, "Enter j"
Prompt m, "Enter k"
Doprompt "Parameters", j, k
jg = j
kg = k
return 0
End
Here is another way using an input panel. Create theWave at the root level and run the Panel Macro named Input. This avoids the need for global variables.
Function UpdateWave()
wave theWave = root:theWave
variable j, k
ControlInfo/W=Input setvarj
j = V_value
ControlInfo/W=Input setvark
k = V_value
theWave = j*p + k
return 0
end
Function SetVarProc(sva) : SetVariableControl
STRUCT WMSetVariableAction &sva
switch( sva.eventCode )
case 1: // mouse up
case 2: // Enter key
case 3: // Live update
Variable dval = sva.dval
String sval = sva.sval
UpdateWave()
break
case -1: // control being killed
break
endswitch
return 0
End
Window Input() : Panel
PauseUpdate; Silent 1 // building window...
NewPanel /W=(403,74,562,159) as "Input"
ShowTools/A
SetVariable setvarj,pos={18,15},size={120,19},proc=SetVarProc,title="j = "
SetVariable setvarj,fSize=12,value= _NUM:0
SetVariable setvark,pos={15,40},size={120,19},proc=SetVarProc,title="k = "
SetVariable setvark,fSize=12,value= _NUM:0
EndMacro
--
J. J. Weimer
Chemistry / Chemical & Materials Engineering, UAHuntsville
February 15, 2012 at 09:22 am - Permalink
Prompt j, "Enter j"
Prompt m, "Enter m"
Doprompt "Parameters", j, m
Variable/G globalJ = j
Variable/G globalm = m
Make/D/O/N=10 theWave; edit theWave
SetFormula theWave, "globalJ*p + globalm"
End
If you want to check for existence of the global variables, and use their current values as a default, do this:
Variable j = NumVarOrDefault("globalJ", 0)
Variable m = NumVarOrDefault("globalm", 0)
Prompt j, "Enter j"
Prompt m, "Enter m"
Doprompt "Parameters", j, m
Variable/G globalJ = j
Variable/G globalm = m
Make/D/O/N=10 theWave; edit theWave
SetFormula theWave, "globalJ*p + globalm"
End
Note that this code ignores the issue of Data Folders. The way this code is written, it will create the global variables in whatever data folder is the current data folder. It will only work correctly if the current data folder is the same as the last time. Most likely, that folder will be root: and you will get along for a long time without any problems. But as time passes you will start using data folders and then it will break mysteriously.
Using data folders correctly in a case like this is difficult. A poor solution, but one that will always work, is to simply always use root:
Variable j = NumVarOrDefault("root:globalJ", 0)
Variable m = NumVarOrDefault("root:globalm", 0)
Prompt j, "Enter j"
Prompt m, "Enter m"
Doprompt "Parameters", j, m
Variable/G root:globalJ = j
Variable/G root:globalm = m
Make/D/O/N=10 theWave; edit theWave
SetFormula theWave, "root:globalJ*p + root:globalm"
End
John Weeks
WaveMetrics, Inc.
support@wavemetrics.com
February 15, 2012 at 09:31 am - Permalink
I will use the solution given by johnweeks, it will be easier for me (I also tried to create a local variable and a global variable equal to the local one, but using ":=" and it didn't work, and I didn't know the setformula operation).
February 15, 2012 at 09:54 am - Permalink