
Programming help for new user
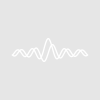
pareitu3
I'm new to Igor (and programming!) and want to apologize in advance for the basic question. I've just started going through the manual but have a somewhat urgent deadline and would be very grateful for some help pointing me in the right direction.
I have two waves, x and V. I need to find the maximum V for every group of 32 points. These maxima would ideally be interpolated, and I should note that every group of points takes the shape of a smooth, yet antisymmetric peak. I then need to make a wave of the x-values corresponding to these maximum V-values. Because I'm working with a high volume of data, manually plotting/calculating this is not feasible. Every set of 32 x-values is identical.
Where would be the best place to start in order to figure this out quickly? I got Igor today and have been going through the tutorial and programming section to get started. I still feel like I'm a long way away from being able to write a program from scratch and am not sure how to go about my main task.
Thanks!
July 22, 2010 at 10:50 pm - Permalink
Wow, thank you so much. I really appreciate it!!
July 26, 2010 at 03:47 pm - Permalink