
Repeat a row n times
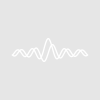
erickbermont
Hello, I want to repeat a row of data with "m" columns several times "n", in order to have a matrix "nxm". Of course I can use Concatenate but this function do not allow to concatenate n times at once. How can I do that?
Let say my data is in the first row as [1,2,3,4,5] and I want to copy that row 500 times to get the matrix [1,2,3,4,5;1,2,3,4,5,...500 times]
Thank you and sorry for such a simple question.
should do the trick.
June 2, 2019 at 05:04 am - Permalink
My reading of the OP's question is that they want a matrix with rows reading 1,2,3,4,5. Thomas's answer will give a matrix with columns reading 1,2,3,4,5. Adding a MatrixTranspose matrix line to the end will therefore give the correct result.
An alternative method is with MatrixOp
June 2, 2019 at 12:53 pm - Permalink
In reply to My reading of the OP's… by sjr51
Thanks a lot. MatrixOP worked well. Also a solution I tried before was concatenating n times the row I wanted to repeat.
If it's possible, can you help me with another problem? I have a wave W which is result from the reading of an instrument that is measuring two inputs during 5 min each:
W= 5 min of data input 1, 5 min of data input 2, 5 min of data input 1, 5 min of data input 2...)
I want to generate two new waves, so then I can separate the signals in W from input 1 and input 2. Something like these two new waves:
Input1=only input 1 data from W
Input2=only input 2 data from W
I hope you can help me. Thank you.
July 3, 2019 at 12:55 am - Permalink
If the runs of data are equal length for each input and let's say it is 5 values for each. You can do:
July 3, 2019 at 06:34 am - Permalink
The first solution repeats the first five points for each input wave. You have to do something more complex for the wave assignment, such as:
This is ugly, but gets the job done. There must be a better way.
However, the solution really depends on how you differentiate between the two runs of values. The above solution only works if the runs are the same length each time. If the lengths of the runs differ then you need to have another way to distinguish the two data sets.
So this begs the question of how you know when one run of data ends and the other begins.
July 3, 2019 at 07:20 am - Permalink
@jtigor: you're correct, my suggestion doesn't work. I didn't test it properly.
July 3, 2019 at 02:07 pm - Permalink
OK here is a corrected version which does not involve writing a loop.
July 3, 2019 at 02:52 pm - Permalink
In reply to OK here is a corrected… by sjr51
Thanks a lot again, your solution works perfectly.
July 4, 2019 at 11:11 pm - Permalink
In reply to The first solution repeats… by jtigor
Thank you for your help. Now I have the problem you mention, the two data sets differ in length each time, but I have other wave that can help me to distinguish between input 1 and input 2. How can I use this "index wave" to separate input 1 and input 2 regarding its length?
For example:
Data=input1, input2, input1, input2...
Where input 1 and input2 have different lengths, but I have an "indexwave" which have values of 0 for input1 and 1 for input2. Then I wan to use the "indexwave" to generate two new waves:
input1=only data from input1
input2=only data from input2
Thanks in advance.
August 6, 2019 at 06:03 am - Permalink
Perhaps the Extract operation would work for you. Something like this:
August 6, 2019 at 12:56 pm - Permalink
In reply to jtigor wrote: However,… by erickbermont
Perhaps you are after something akin to this:
Then remove the NaN values.
August 6, 2019 at 01:05 pm - Permalink
Thank you verz much for your response, I already tried your solutions and they work, however, my index file now got more complicated, now it contains date data, so then I have:
Data=X sec of input 1, Y sec of input 2, X sec of input 1, Y sec of input 2
IndexWave=Date&Time (format MM/DD/YYYY HH:MM:SS)
Still I want to generate two waves:
Input1=Only data from input 1
Input 2=Only data from input 2
Now the problem is that my index wave is in date-time format and I know the times when the data is switching from input1 to input2 and so on. The question still more less the same: How can I use my date-time index wave to separate input1 data from input 2 data?. I supousse that there is no way to program this since the times when the data is switching are very specific and not something really periodic. I already tried with the function "extract", but it does not accept time as parameter.
Thank you in advance.
August 8, 2019 at 08:04 am - Permalink
In reply to Thank you verz much for your… by erickbermont
if you can write a function whichInput(strDateTime) that decides which input you have at a given time you can do it like this:
whichInput(strDateTime) should return 0 or 1 based on Date&Time.
August 8, 2019 at 08:28 am - Permalink
In reply to if you can write a… by tony
Thank you, but this only work if the dimension of all waves is the same. In my case the date-time index wave has 1 sec resolution while Data has 30 sec resolution.
August 8, 2019 at 08:57 am - Permalink
In reply to Thank you, but this only… by erickbermont
I think Tony's answer is correct even with your situation of different sizes. He is saying though that you need a function to determine which row should be sorted to which wave. If you can do this, his solution followed by zapnans on both waves will give you what you want.
August 9, 2019 at 08:27 am - Permalink