
Time & Date Import as a single string
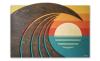
Hi, I have looked through the various other posts but haven't found a solution for this particular issue. Probably due to lack of Igor coding knowledge at the moment.
I'm trying to import a csv file through the load waves menu option. I understand about tweaking the data such that the date is formatted correctly but IP doesn't allow for 'time date' only 'date time'.
I can use 'space' as a deliminator to separate the time and date into two waves, doing so requires you to turn 'read wave names' off as there is no 'date' column name.
The data is in the format 'hh:mm:ss dd-mmm-yyyy' the mmm is abbreviated alphabetic i.e. 'Sep'. Ideally I want to read this as a single wave, instead of reading it as two for every dataset then combining it back into one.
I have attached a sample of the dataset (which is too long to open in excel to format the data first).
I tried to follow this post: https://www.wavemetrics.com/code-snippet/create-datetime-wave-text-waves and load both as text waves then create a date&time wave but it just comes up with command errors 'expected wave name....' and I don't know enough to make it work yet. My date format is different also, but I don't get that far.
Any help appreciated
There is nothing built-in in Igor that can interpret "JAN", "FEB", etc, as months so you need to write code to do that.
Here is a solution. If you are running Igor Pro 6, you need to replace "int" and "double" with "Variable".
November 8, 2020 at 10:31 am - Permalink
Here is an edit of that function to convert your date format to an Igor format.
To use this, you need to create a double precision wave with the same size as your date/time textwave, then execute
DateTimeWave=ConvertTextToDateTime(textWave)
EDIT: oops, too slow!
November 8, 2020 at 10:38 am - Permalink
Thanks Howard for your input. I can read in the data now and it looks great however the month is not reading correctly.
The text wave 'DateAsText' reads the date correctly, beginning 08/05/2016 and incrementing but the 'DateAndTime' wave begins 08/01/2016 and the month doesn't increment at all.
The day and year along with time import perfectly. The data becomes multiple overlapping traces within the single month of January.
I've attached a longer segment of the archive for your reference.
Another question is regarding quote 'There is nothing built-in in Igor that can interpret "JAN", "FEB", etc, as months so you need to write code to do that'. This confused me as when I was using the load waves/load waves/Tweaks function on IP8 it allows me to do 'Other' date format and customize the month as 'Abbreviated alphabetic'. This reads the date wave and gives me the correct igor format e.g. 08/05/2016 output as a numeric wave.
November 8, 2020 at 10:18 pm - Permalink
Yes. The problem is that I forgot that WhichListItem is case-sensitive by default. I have changed it in the procedure above.
It is actually I who was confused. I forgot that LoadWave supported alphabetic dates even though I wrote the code (about 25 years ago :)
Here is a revised procedure that uses this feature of LoadWave:
November 9, 2020 at 04:44 am - Permalink
works perfectly. Thank you very much!
November 9, 2020 at 04:58 am - Permalink