
User defined curve fitting
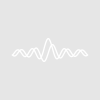
graduate_student_1
Function GGaussian(xx, mu, sigma) Variable xx, mu, sigma return exp(-(xx-mu/sigma)^2/2)/sigma/sqrt(2*pi) End Function Heaviside(x2, x3) Variable x2, x3 return x2 < x3 ? 0 : 1 End Function CustomFitFunc(w, x) :FitFunc wave w variable x Variable Ao=w[0] Variable Eg=w[1] Variable Eb=w[2] Variable sigma = w[3] Variable i, exciton, result, continu, sp for(i=1; i<4; i+=1) exciton += Ao*Eb*4/i^3*pi*GGaussian(x, Eg-(Eb/(i^2)), sigma) endfor sp=sqrt(Eb/(x-Eg)) continu=Ao*pi*exp(pi*sp)/sinh(pi*sp)*Heaviside(x, Eg) result=continu+exciton return result End
Hello. I want to fit my data on absorption coefficient. The organized customfit Function for curve fitting above can be compiled.
However, the error message ('singular matrix or other numeric error') is appeared when I try curve fitting.
How can I get through this?
Please let me know the solution.
Are there any messages in the history about "These parameters may be linearly dependent:" or "There may be no dependence on these parameters:"?
The first means that two (or more) coefficients vary together in a way that prevents the curve fit from distinguishing between them. The second means that one or more coefficients when perturbed by a small amount for the purposes of computing numerical derivatives didn't cause any change in chi-square. The first is a mathematical problem with your fitting function; it can't be fixed except by combining or holding some coefficients. The second can be fixed using an epsilon wave: DisplayHelpTopic "The Epsilon Wave"
It can also happen if your initial guess isn't good enough, or there is something pathological about your fitting function that makes the solution run *away* from convergence.
June 28, 2023 at 09:08 am - Permalink
What happens if you plot your fit function using initial guess values? Have you found values for initial guesses that give an output in the vicinity of your data?
June 28, 2023 at 09:51 am - Permalink
To start with, I think there is error here:
I think you are missing brackets:
I did not check more, but critical is to test that the code actually works when not used for fitting.
June 28, 2023 at 10:33 am - Permalink