
Arbitrary Scaling of Gizmo Plot
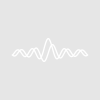
sgreenfield
// ************************************************************************************** // This section of code is PART of the Gizmo window creation routine. // It sets up clip planes to prevent data that extends beyond the xyz range of the Gizmo cube from being displayed. // The first line sets things up for non-unity scaling, if desired later. ModifyGizmo setDisplayList=1, opName=scale0, operation=scale, data={1,1,1} // enables non-even scaling (default is cubic) ModifyGizmo setDisplayList=2, opName=enable0, operation=enable, data=12288 // enables clip plane 0 ModifyGizmo setDisplayList=3, opName=enable1, operation=enable, data=12289 // enables clip plane 1 ModifyGizmo setDisplayList=4, opName=enable2, operation=enable, data=12290 // enables clip plane 2 ModifyGizmo setDisplayList=5, opName=enable3, operation=enable, data=12291 // enables clip plane 3 ModifyGizmo setDisplayList=6, opName=enable4, operation=enable, data=12292 // enables clip plane 4 ModifyGizmo setDisplayList=7, opName=enable5, operation=enable, data=12293 // enables clip plane 5 ModifyGizmo setDisplayList=8, opName=ClipPlane0, operation=ClipPlane, data={0,0,0,1,1} // clips at bottom ModifyGizmo setDisplayList=9, opName=ClipPlane1, operation=ClipPlane, data={1,0,0,-1,1} // clips at top ModifyGizmo setDisplayList=10, opName=ClipPlane2, operation=ClipPlane, data={2,1,0,0,1} // clips at xmin ModifyGizmo setDisplayList=11, opName=ClipPlane3, operation=ClipPlane, data={3,-1,0,0,1} // clips at xmax ModifyGizmo setDisplayList=12, opName=ClipPlane4, operation=ClipPlane, data={4,0,1,0,1} // clips at ymin ModifyGizmo setDisplayList=13, opName=ClipPlane5, operation=ClipPlane, data={5,0,-1,0,1} // clips at ymax ModifyGizmo setDisplayList=14, object=surface0 // actual surface ModifyGizmo setDisplayList=15, opName=disable0, operation=disable, data=12288 // disables clip plane 0 ModifyGizmo setDisplayList=16, opName=disable1, operation=disable, data=12289 // disables clip plane 1 ModifyGizmo setDisplayList=17, opName=disable2, operation=disable, data=12290 // disables clip plane 2 ModifyGizmo setDisplayList=18, opName=disable3, operation=disable, data=12291 // disables clip plane 3 ModifyGizmo setDisplayList=19, opName=disable4, operation=disable, data=12292 // disables clip plane 4 ModifyGizmo setDisplayList=20, opName=disable5, operation=disable, data=12293 // disables clip plane 5 // ************************************************************************************** // ************************************************************************************** // Procedure manually sets the XYZ range of the Gizmo plot // NOTE: one can return to autorange (i.e., plotting full range of data) with: // ModifyGizmo ScalingMode=2 Proc SetGizmoScales(xMin, xMax, yMin, yMax, zMin, zMax) Variable xMin, xMax, yMin, yMax, zMin, zMax ModifyGizmo setOuterBox={xMin,xMax,yMin,yMax, zMin, zMax} ModifyGizmo update=2 // NOTE: doesn't work with update=1 EndMacro // ************************************************************************************** // ************************************************************************************** // Function allows Gizmo display cube to be set to something other than cube. // XY aspect ratio can be set to match displayed xy data range. // Z aspect ratio can be set arbitrarily anywhere from 0-1 Function GizmoRescale() Variable zAxisScaling = 1 Variable xyScalingMode Prompt zAxisScaling, "Scaling Factor for z-axis (default=1)" Prompt xyScalingMode, "XY axes scaling", popup, "None (default);Scaled to displayed xy data range" DoPrompt "Gizmo Scaling Parameters", zAxisScaling, xyScalingMode if(V_Flag) return -1 // user cancelled endif String Cmd Variable xAxisScaling = 1 Variable yAxisScaling = 1 Variable diag // want to scale xy plane to data's aspect ratio if(xyScalingMode==2) NVAR /Z aspectRatio = root:gGizmoXYaspectRatio // displayed aspect ratio already stored as global variable if( !NVAR_Exists(aspectRatio) ) Variable /G root:gGizmoXYaspectRatio = 1 print "Warning: unknown xy aspect ratio - setting to unity" endif if(aspectRatio>1) yAxisScaling = aspectRatio else xAxisScaling = 1/aspectRatio endif diag = sqrt(xAxisScaling^2 + yAxisScaling^2) // this scaling always shrinks - compensate xAxisScaling *= sqrt(2)/diag yAxisScaling *= sqrt(2)/diag endif // create actual display list (order is important) sprintf Cmd, "ModifyGizmo setDisplayList=1, opName=scale0, operation=scale, data={%f,%f,%f}", xAxisScaling, yAxisScaling, zAxisScaling Execute Cmd End // **************************************************************************************
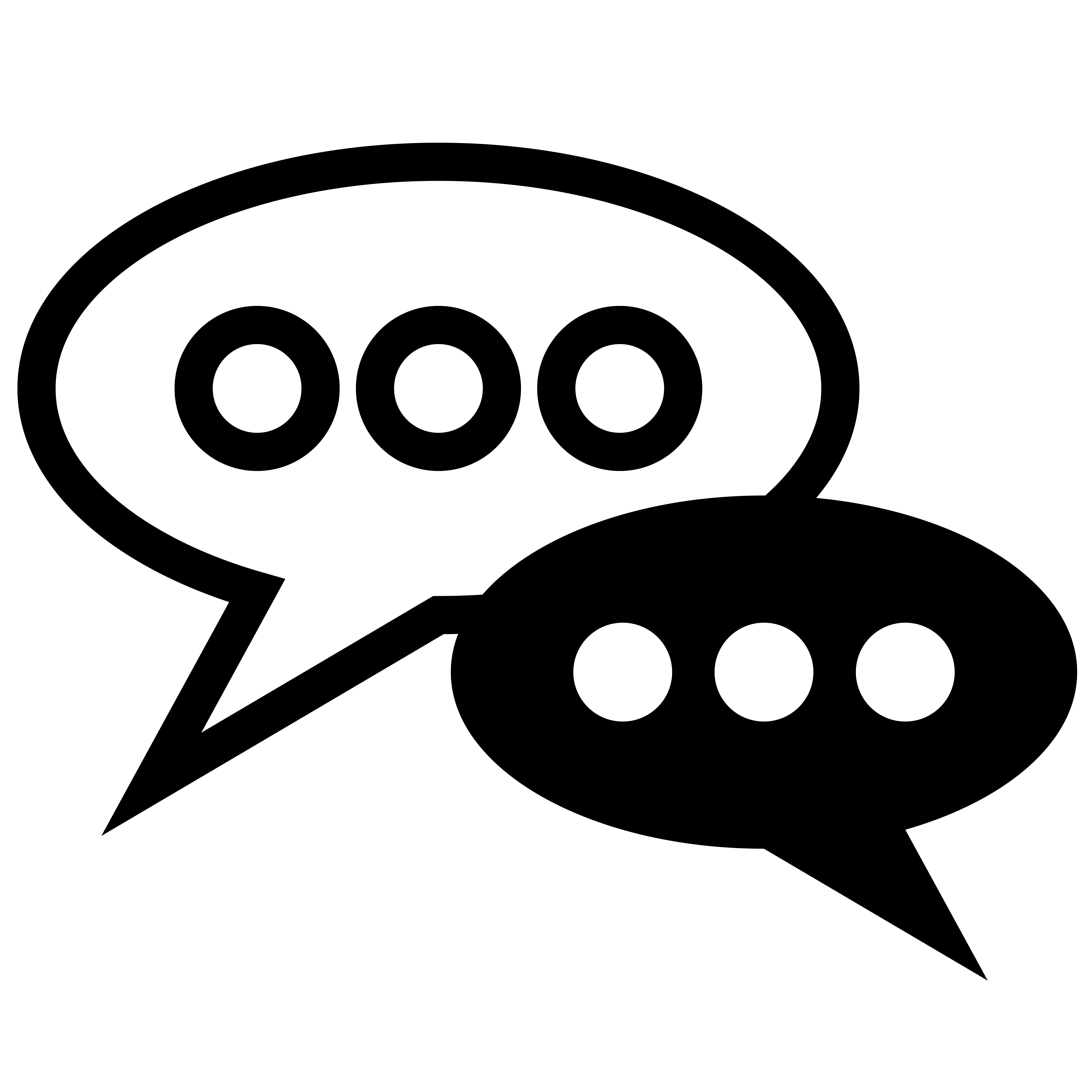
Forum
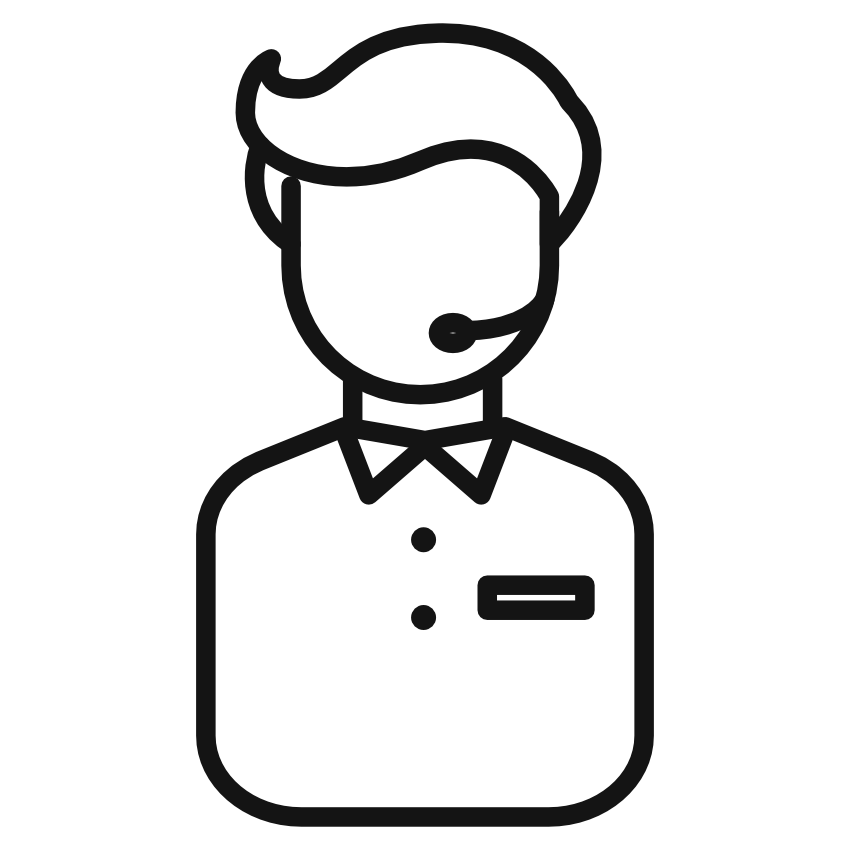
Support
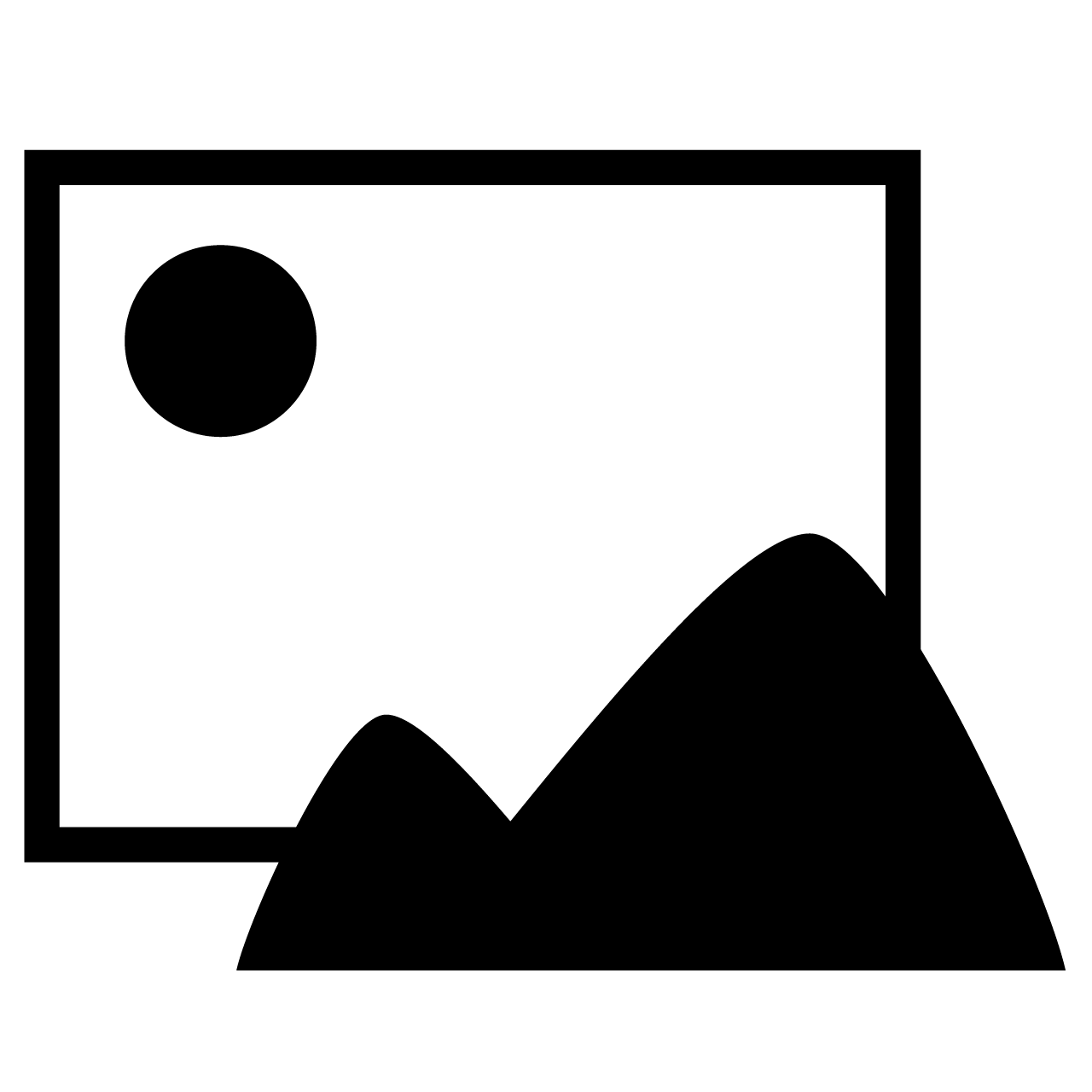
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More