
C Code to add a Cocoa Window to and Igor XOP
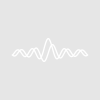
KevinRBoyce
There is no interaction whatsoever between Igor and the window, so I have high hopes that it will continue to work in Igor 7.
The hard part (for me) was finding and loading the correct nib file. For those of you familiar with Cocoa, here is the main structure of how I do it. This of course needs to be in an objective-C (.m) file.
Once you've done this, you can put stuff in the window either by making IBOutlets and connecting them to views in the window, or with bindings. I use a combination of the two. I put all the outlets in my window controller. You could put them in the window, but it's closer to the Model-View-Controller design pattern to put them in the controller.
The window seems to get all the usual events, and coexists very nicely with Igor. I have working buttons in my window, and I also handle keydown events (which I send to the Igor command line, since you're not supposed to be able to type in my window.)
<br /> <br /> <br /> // I subclassed NSWindow, mainly so I could handle keydown events.<br /> // I subclassed NSWindowController to handle actions from controls in the window.<br /> // I store the window and its controller in global variables.<br /> MyWindowClass* myCocoaWindow;<br /> MyWindowController* myWinController;<br /> <br /> // Create the window and its controller. I store the NSWindow and the NSWindowController<br /> // in global variables, but you could return them.<br /> // I also never release the window, so it just gets hidden when closed. If you want to<br /> // release it, you could do that (and remember to nil out the global variable!) in<br /> // the delegate's WindowWillClose method, or in the window's Close method (assuming you<br /> // subclass NSWindow).<br /> static long ShowMyCocoaWindow()<br /> {<br /> long err = 0;<br /> NSString* title;<br /> NSBundle* xopBundle; // CAUTION: CFBundles are NOT toll-free bridged with NSBundles.<br /> NSArray* nibObjects;<br /> <br /> // Don't forget to create your own autorelease pool, since we're not in a regular run loop.<br /> // If we don't do this, all autoreleased objects we create below will leak.<br /> NSAutoreleasePool* pool = [[NSAutoreleasePool alloc] init];<br /> <br /> if( myCocoaWindow )<br /> {<br /> // It already exists. Just bring it to the front.<br /> [myCocoaWindow makeKeyAndOrderFront:nil];<br /> }<br /> else<br /> {<br /> // First we have to find the bundle containing the XOP.<br /> // bundleWithIdentifier looks first in our own process, so it will always<br /> // find the one that was actually loaded. Nice!<br /> xopBundle = [NSBundle bundleWithIdentifier:@"gov.nasa.gsfc.myxop"];<br /> <br /> if( xopBundle )<br /> {<br /> // Now find the desired nib in this bundle.<br /> NSNib* winNib = [[NSNib alloc] initWithNibNamed:@"MyWindowName" bundle:xopBundle];<br /> <br /> if( winNib )<br /> {<br /> // Found the nib! Create a window controller with no window.<br /> myWinController = [[XGXboxWinController alloc] initWithWindow:nil];<br /> <br /> // Instantiate the nib containing the window.<br /> [winNib instantiateNibWithOwner:myWinController topLevelObjects:&nibObjects];<br /> <br /> // Find the window amongst the top objects in the nib. <br /> // There may be a more elegant way to do this, but this is guaranteed to work<br /> <br /> NSEnumerator* enumerator = [nibObjects objectEnumerator];<br /> id thisObject;<br /> while( (thisObject = [enumerator nextObject] ) )<br /> {<br /> if( [thisObject isKindOfClass:[NSWindow class]] )<br /> {<br /> myCocoaWindow = thisObject;<br /> break;<br /> }<br /> }<br /> <br /> // Now set the window controller to control this window, and <br /> // copy the window to my global variable<br /> [myWinController setWindow:myCocoaWindow];<br /> <br /> // Title the window appropriately. title is autoreleased; good thing we have an autorelease pool, eh?<br /> title = [NSString stringWithFormat:@"A Cocoa Window"];<br /> [myCocoaWindow setTitle:title];<br /> }<br /> }<br /> }<br /> <br /> [pool release];<br /> <br /> return err;<br /> } <br />
That's it! The window and controller have no other code that deals with creating it or updating it. I have a bunch of IBOutlet NSTextFields in the controller, which I have connected to the text fields in Interface Builder, and when I have new data I just say [[myWinController someTextField] setFloatValue:x] and let the Cocoa runtime do the rest.
Oh, here is the code I use to make sure key events that go to this window are redirected to Igor's command window. This goes in MyWindowClass.m
å<br /> - (void)keyDown:(NSEvent *)theEvent<br /> {<br /> NSString* chars;<br /> <br /> // Put the character(s) that we got onto the command line<br /> chars = [theEvent characters];<br /> PutCmdLine( [chars cStringUsingEncoding:NSASCIIStringEncoding], INSERTCMD );<br /> <br /> // Bring command window to the front<br /> XOPSilentCommand( "DoWindow/H" );<br /> }<br />
Left as an exercise for the reader, because I haven't done it yet, is handling command-whatever key combinations, which do not arrive as key events.
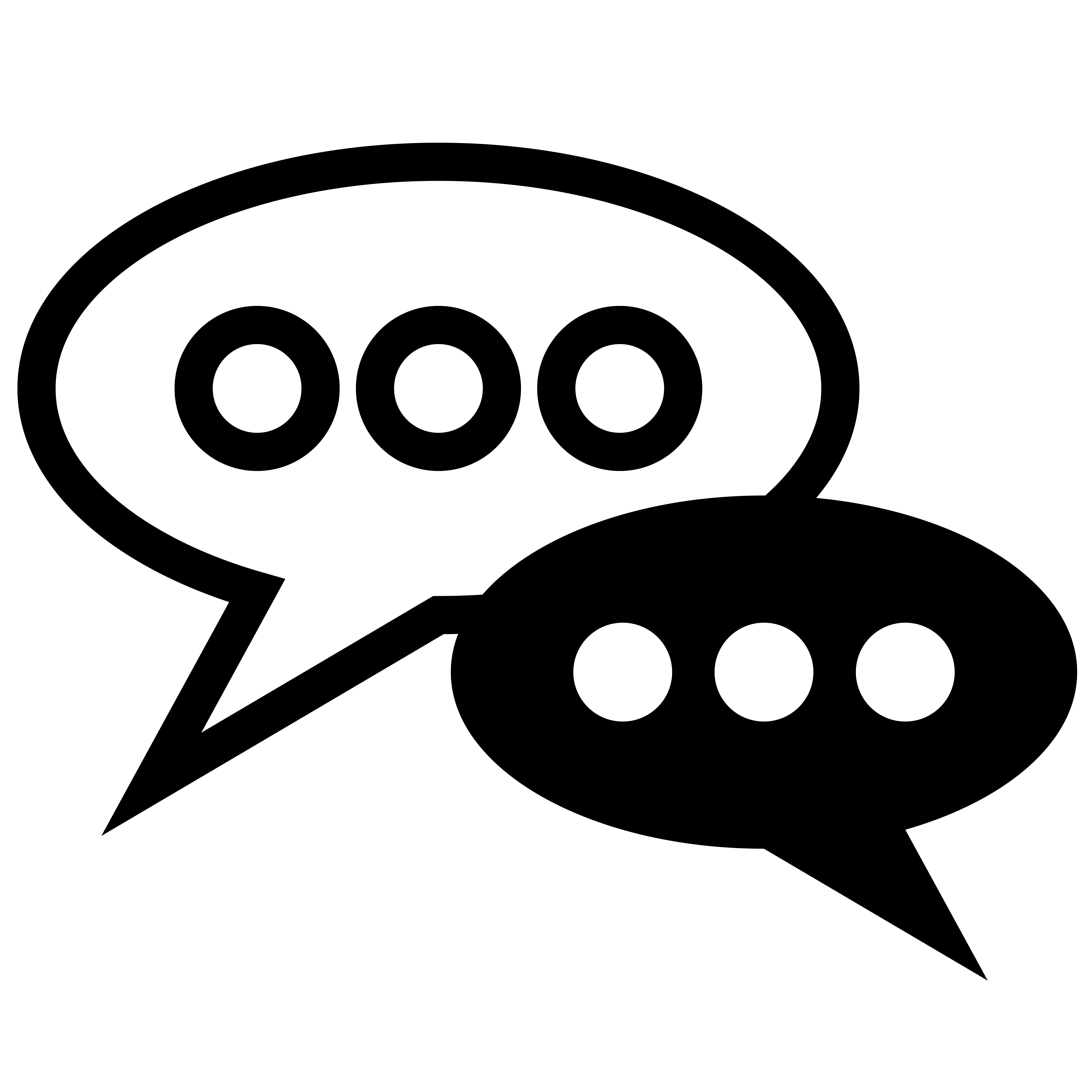
Forum
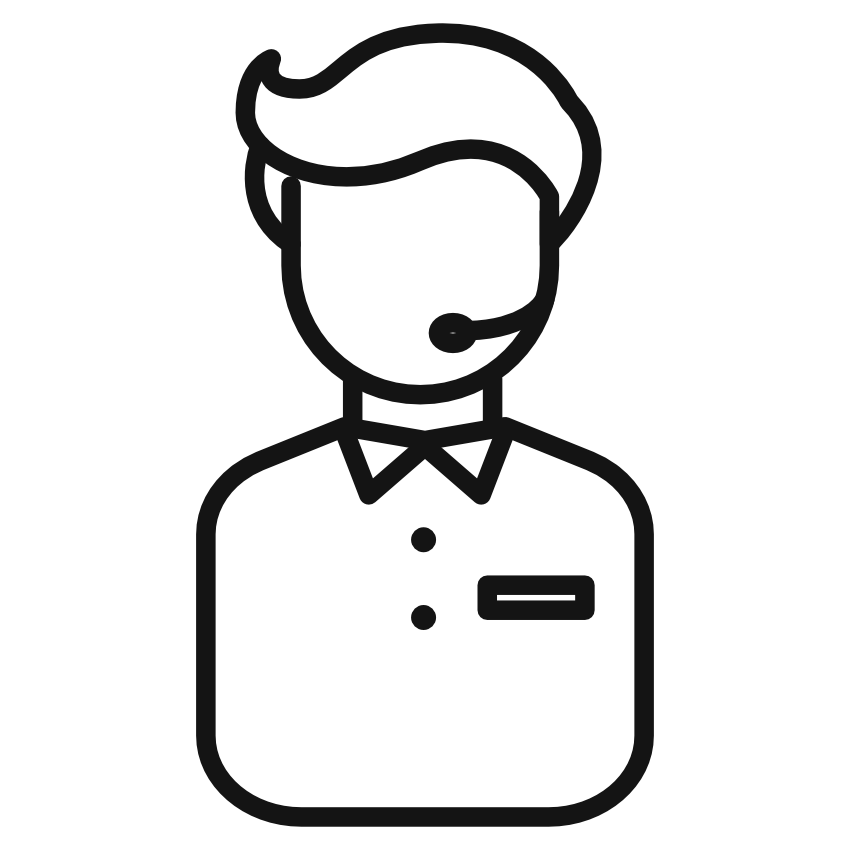
Support
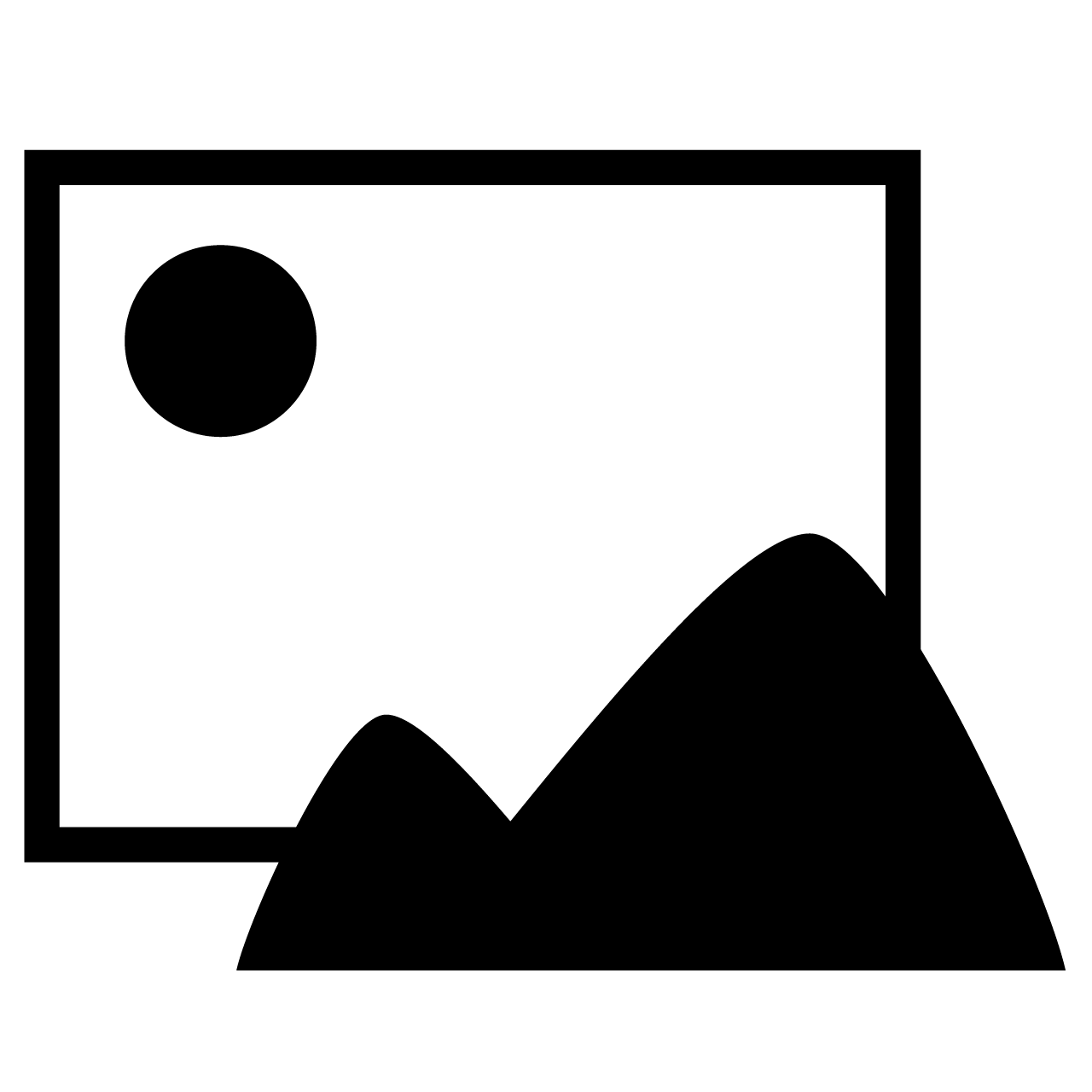
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More