
DrawArcWedge
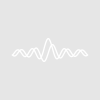
harneit
The parameters are: Origin = (x0, y0), Arc1 = (r1, phi1-->phi2), Arc2 = (r2, phi2-->phi1), the arcs are connected by lines. See the grey object in the image. If one of the radii is 0, you get a pizza wedge. If r1==r2, it's just an arc. If r1*r2 < 0, it's a kind of fan (see the orange colored object in the image).
The coordinate system is similar to DrawArc: 0° is at 3 o'clock, the angle is counterclockwise. No choice is made for the drawing coordinate system, you have to set it up with SetDrawEnv.
To try out DrawArcWedge, after having compiled your procedure window, use:
Display; ModifyGraph width={Aspect,1} // make a square graph so that a circle is a circle SetDrawEnv fillfgc= (47872,47872,47872); DrawArcWedge(0.5, 0.5, 0.25, 0.45, 150, 210) // a grey "c" SetDrawEnv fillfgc= (65280,43520,0); DrawArcWedge(0.5, 0.5, -0.25, 0.45, 60, 120) // an orange fan
Here's the whole DrawArcWedge snippet:
// DrawArcWedge draws an arced wedge, i.e. a polygon delimited by two concentric arcs. // Origin = (x0, y0), Arc1 = (r1, phi1-->phi2), Arc2 = (r2, phi2-->phi1), the arcs are connected by lines. // If one of the radii is 0, you get a pizza wedge. If r1==r2, it's just an arc. If r1*r2 < 0, it's a fan. // The coordinate system is similar to DrawArc: 0° is at 3 o'clock, the angle is counterclockwise. // No choice is made for the drawing coordinate system, you have to set it up with SetDrawEnv. // To try out DrawArcWedge without the overhead of the other functions in this proc file, use: // Display; ModifyGraph width={Aspect,1} // make a square graph so that a circle is a circle // SetDrawEnv fillfgc= (47872,47872,47872); DrawArcWedge(0.5, 0.5, 0.25, 0.45, 150, 210) // a grey "c" // SetDrawEnv fillfgc= (65280,43520,0); DrawArcWedge(0.5, 0.5, -0.25, 0.45, 60, 120) // an orange fan // (the easy way: select the three lines above, choose "Decommentize" (Edit menu), then hit "Ctrl+Enter") function DrawArcWedge(x0, y0, r1, r2, phi1, phi2) variable x0, y0, r1, r2, phi1, phi2 // 1. set up basic variables ("hard-wired" section) variable rad = 180 / pi // sin() and cos() need radians, not degrees variable dphi = phi2 - phi1 // difference angle, might be zero variable intphi = 5 // use approx 5° intervals between polygon points in arcs variable nphi = max( floor(dphi / intphi), 1 ) // number of arc points - 1 // "max(.., 1)" prevents division by zero (short arcs also need two end points) dphi /= nphi // (interval for the arc points) = (difference angle) / (number of arc points - 1) // 2. draw the ArcWedge // 2.1 draw the first point on radius r1, at angle phi1 variable angle = phi1 / rad variable x1 = r1*cos(angle), y1 = -r1*sin(angle) // note: this implements the coordinate system of DrawArc DrawPoly x0+x1, y0+y1, 1, 1, {x1, y1} // use an unnamed polygon instead of a wave pair to make each ArcWedge unique // 2.2 draw the arc on radius r2, from angle phi1 to phi2 (implicitely creates line from r1 to r2, at phi1) variable k, kmax kmax = nphi * ( r2 != 0 ) // 1st arc at r2 (only one point iff r2 == 0) for( k = 0; k <= kmax; k += 1 ) // integers are better for the loop to prevent rounding errors angle = (phi1 + k*dphi) / rad DrawPoly/A {r2*cos(angle), -r2*sin(angle)} endfor // 2.3 draw the arc on radius r1, from angle phi2 to phi1 (implicitely creates line from r2 to r1, at phi2) kmax = nphi * ( r1 != 0 ) // 2nd arc at r1 (only one point iff r1 == 0) for( k = 0; k <= kmax; k += 1 ) angle = (phi2 - k*dphi) / rad // note: loop direction inverted DrawPoly/A {r1*cos(angle), -r1*sin(angle)} endfor end
Have fun!
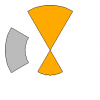
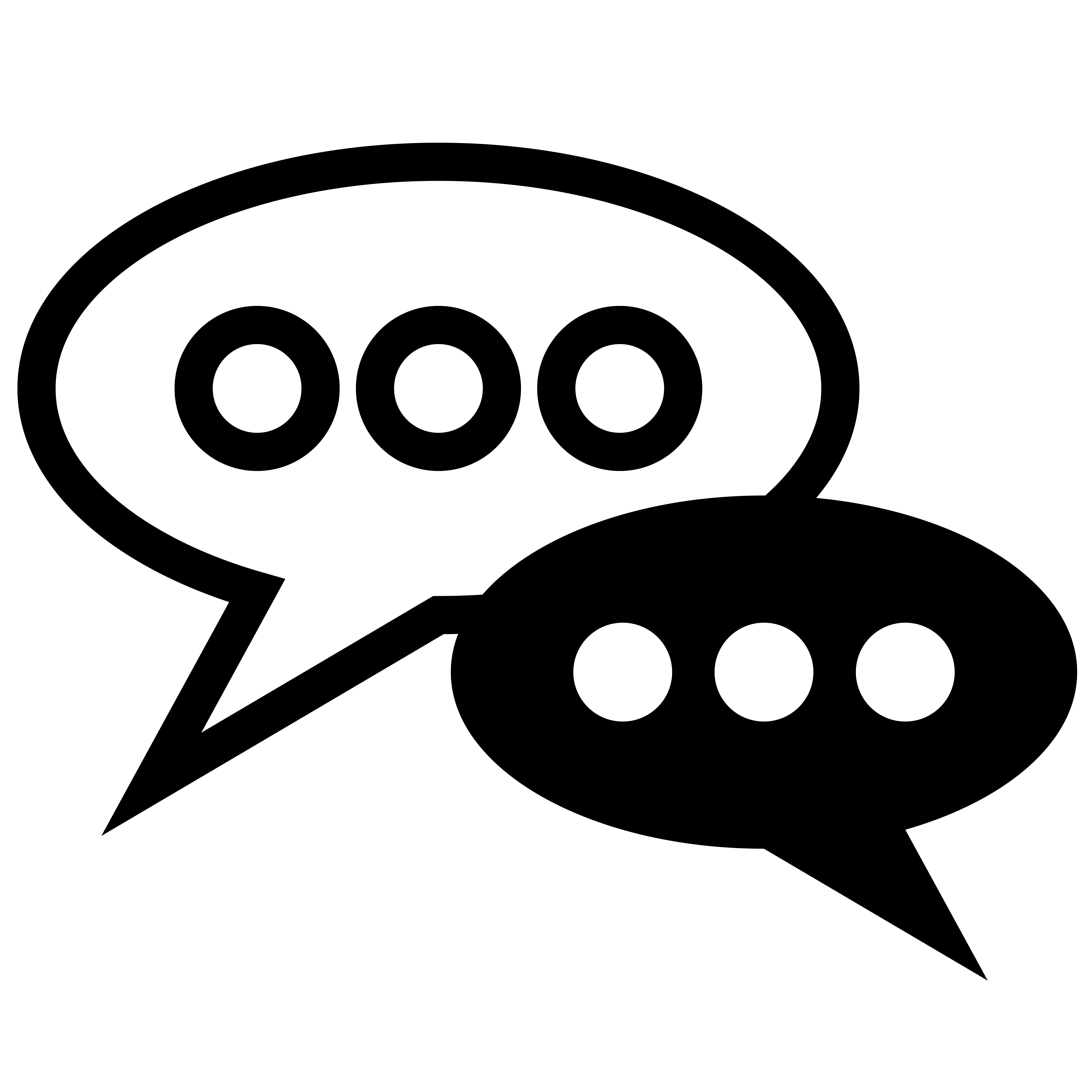
Forum
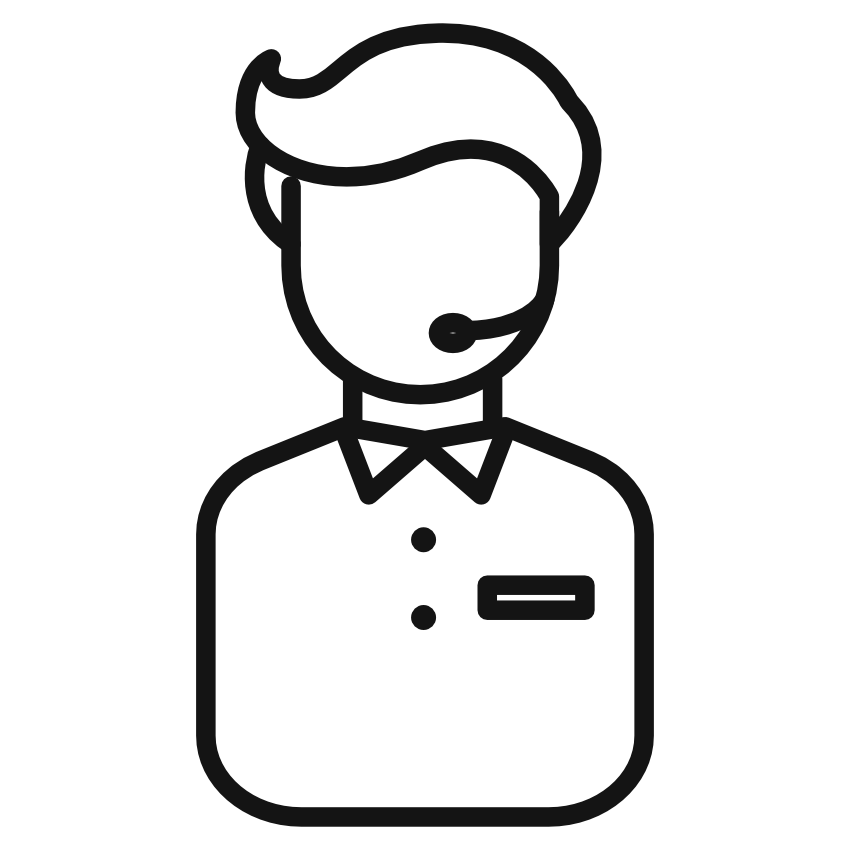
Support
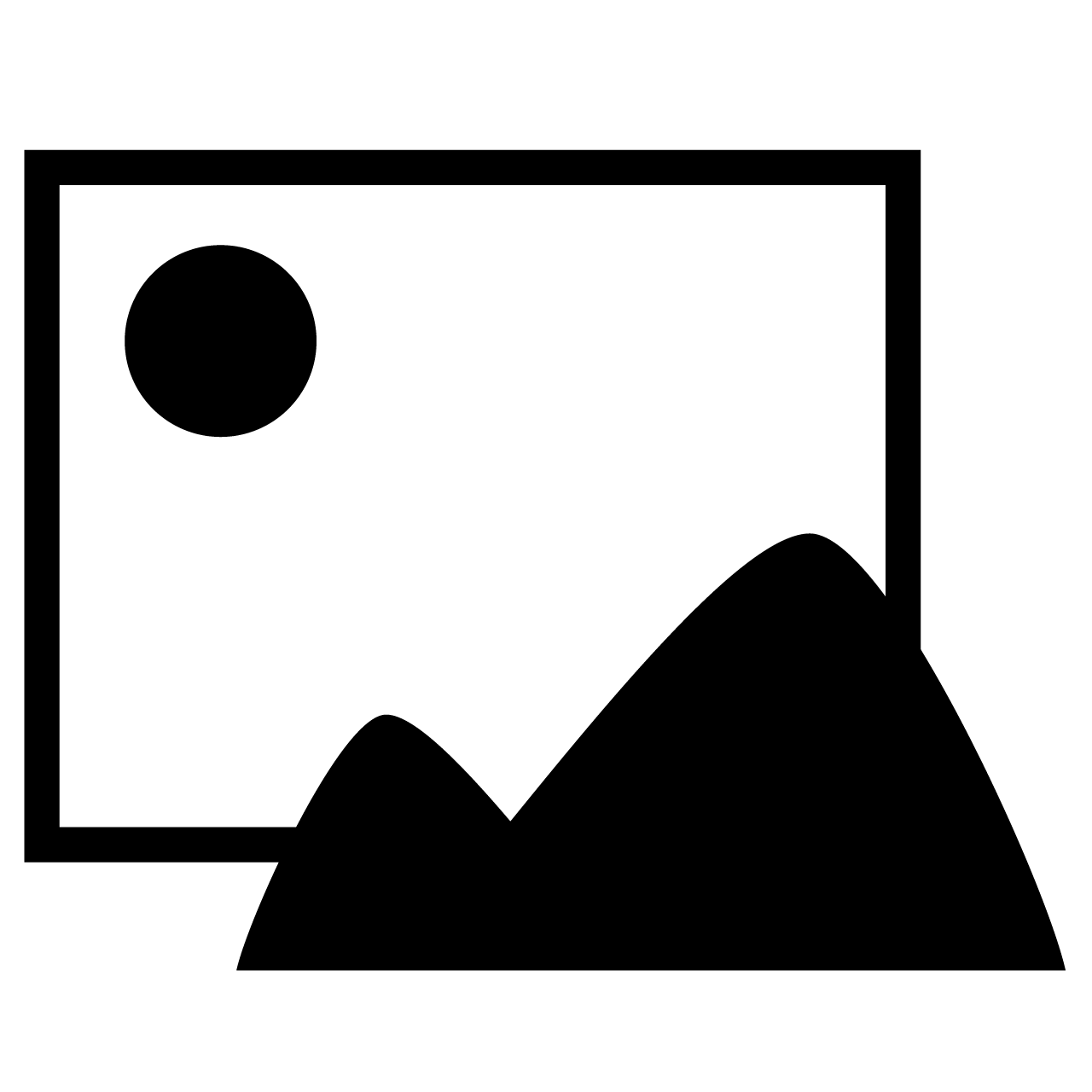
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More