
getXRDML() - function to load xrdml data from Panalytical diffractometers using the XOP XMLutils
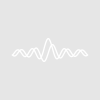
harvey
It creates a folder for each xrdml-file read. The foldername will be the xrdml-filename in lower case letters without the fileextension. The intensities are loaded into waves called 'intensities##' stored in the folder /XMLutil/XRD/'filename'.
It can also handle mutiple (repeated) scans in one xrdml-file by assigning a number '##' to each intensities wave. It also reads the scan comments and additional information about each scan in the file like scannumber, start-,endtime, start-,endposition, measurement program and so on and stores them in a note to each intensities wave.
I am grateful to andyfaff for helping me putting his XMLutils to use with the xrdml files and for reviewing this snippet.
#pragma rtGlobals=1 // Use modern global access method. Function getXRDML() variable fileID variable ii, llength, numscans, iii, numcommlines, start, finish, numrows string folder="", noteStr="", noteAddStr="", noteFinStr="" open/r/d/t="????"/M="Please select the XRDML file" fileID if(strlen(S_filename)>0) fileID = XMLopenfile(S_fileName) if(fileID<1) print "Error, can't load file" return 0 endif else return 0 endif //Make DataFolder according to XRDML-Filename llength = ItemsInList(S_fileName, ":") folder = StringFromList(llength-1, S_fileName, ":") folder = RemoveEnding(lowerstr(folder), ".xml") folder = RemoveEnding(lowerstr(folder), ".xrdml") //printf "\rfolder=%s", folder NewDataFolder/S/O root:XMLutils NewDataFolder/S/O root:XMLutils:XRD NewDataFolder/S/O $folder //find out how many scans there were. XMLlistXpath(fileID, "//xrdml:scan", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3") Wave/t M_listXPath numscans = dimsize(M_listXPath,0) //get measurement info and add as note to the wave XMLwaveFmXPath(fileID, "/xrdml:xrdMeasurements/xrdml:comment/xrdml:entry", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "\n") Wave/T M_xmlContent, W_xmlContentNodes numcommlines=dimsize(M_xmlContent,1) for(iii=0; iii<numcommlines; iii+=1) noteStr=noteStr+M_xmlContent[0][iii]+"\r" endfor //get measurement program comment XMLwaveFmXPath(fileID, "/xrdml:xrdMeasurements/xrdml:xrdMeasurement/xrdml:comment/xrdml:entry", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "\n") noteStr=noteStr+"Measurement program comment:\r"+M_xmlContent[0][0]+"\r" //get sample id XMLwaveFmXPath(fileID, "//xrdml:sample/xrdml:id", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "\n") noteStr=noteStr+"Sample Name: "+M_xmlContent[0][0]+"\r" //get generator/tube info XMLwaveFmXPath(fileID, "//xrdml:incidentBeamPath/xrdml:xRayTube/xrdml:anodeMaterial", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "\n") noteStr=noteStr+"Tube: " + XMLstrFmXPath(fileID, "//xrdml:incidentBeamPath/xrdml:xRayTube/@name", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "") + " @ " XMLwaveFmXPath(fileID, "//xrdml:incidentBeamPath/xrdml:xRayTube/xrdml:tension", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "\n") noteStr=noteStr+M_xmlContent[0][0]+"kV/" XMLwaveFmXPath(fileID, "//xrdml:incidentBeamPath/xrdml:xRayTube/xrdml:current", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "\n") noteStr=noteStr+M_xmlContent[0][0]+"mA\r" //get wavelength info XMLwaveFmXPath(fileID, "//xrdml:usedWavelength", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "\n") noteStr=noteStr+"Wavelengths:\rkAlpha1: "+M_xmlContent[0][0]+"\rkAlpha2: "+M_xmlContent[0][1]+"\rkBeta: "+M_xmlContent[0][2]+"\r" //load all the xrd intensities in. for(ii=1 ; ii<numscans+1 ; ii+=1) //load the intensities and put the scaling on the wave XMLwaveFmXpath(fileID, "//xrdml:scan["+num2str(ii)+"]/xrdml:dataPoints/xrdml:intensities", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "") Wave/T M_xmlContent, W_xmlContentNodes numrows = dimsize(M_xmlContent,0) make/o/d/n=(numrows) $"intensities"+num2istr(ii) Wave intensity = $"intensities"+num2istr(ii) intensity = str2num(M_xmlcontent) //get scan info and add to the wave note //get start/end time of scan noteAddStr=noteAddStr + "--- Scan #"+num2str(ii)+"---\r" noteAddStr=noteAddStr+"Start time: " + XMLstrFmXPath(fileID, "//xrdml:scan["+num2str(ii)+"]/xrdml:header/xrdml:startTimeStamp", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "") +"\r" noteAddStr=noteAddStr+"End time: " + XMLstrFmXPath(fileID, "//xrdml:scan["+num2str(ii)+"]/xrdml:header/xrdml:endTimeStamp", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "") +"\r" //here comes the scaling XMLwaveFmXpath(fileID, "//xrdml:scan["+num2str(ii)+"]//xrdml:positions[@axis='2Theta']/xrdml:startPosition", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "") start = str2num(M_xmlcontent[0]) noteAddStr = noteAddStr + "Start position: " + M_xmlContent[0] +"\r" XMLwaveFmXpath(fileID, "//xrdml:scan["+num2str(ii)+"]//xrdml:positions[@axis='2Theta']/xrdml:endPosition", "xrdml=http://www.xrdml.com/XRDMeasurement/1.3", "") finish = str2num(M_xmlcontent[0]) noteAddStr = noteAddStr + "End position: " + M_xmlContent[0] +"\r" noteFinStr=noteStr+noteAddStr Note/NOCR intensity, noteFinStr noteAddStr="" noteFinStr="" SetScale/I x, start, finish, intensity endfor killwaves/z M_Xmlcontent, W_xmlcontentnodes, M_listXPath if(fileID) xmlclosefile(fileID, 0) endif SetDataFolder root:XMLutils:XRD return 0 End
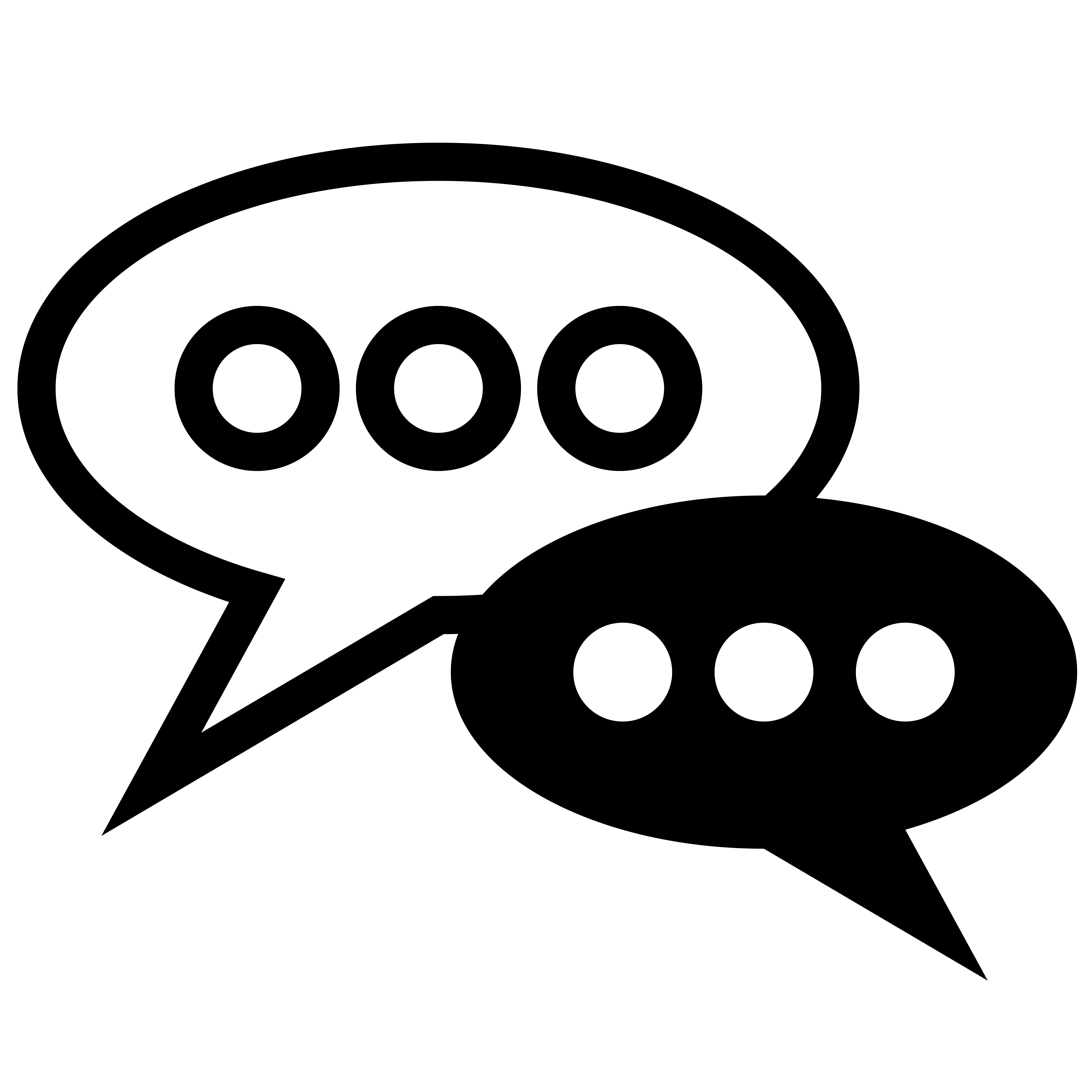
Forum
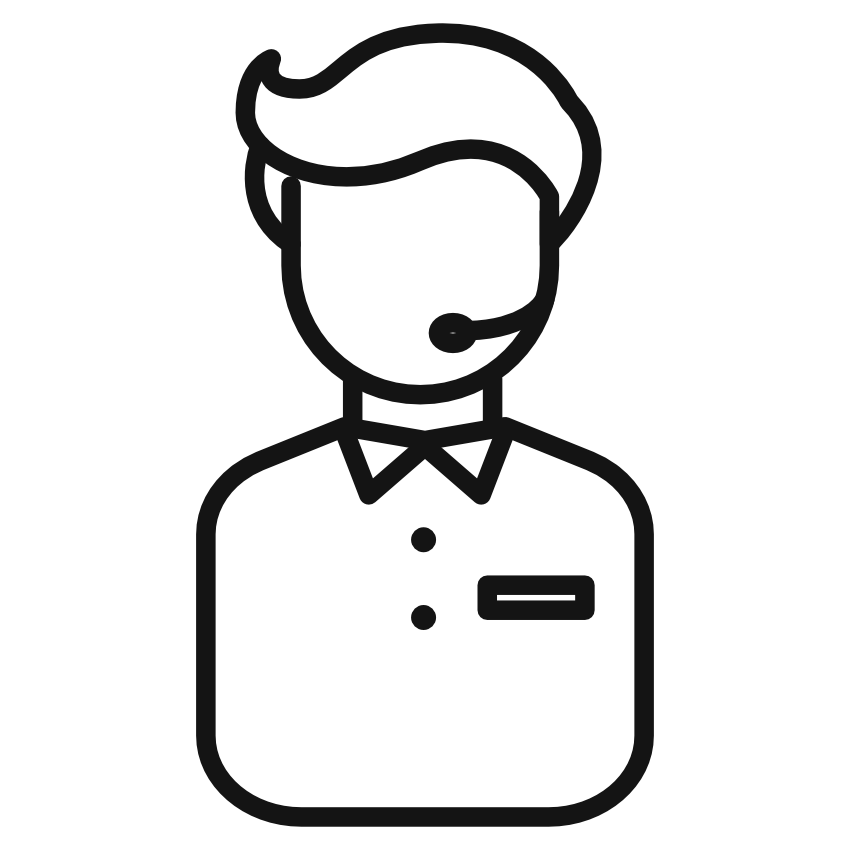
Support
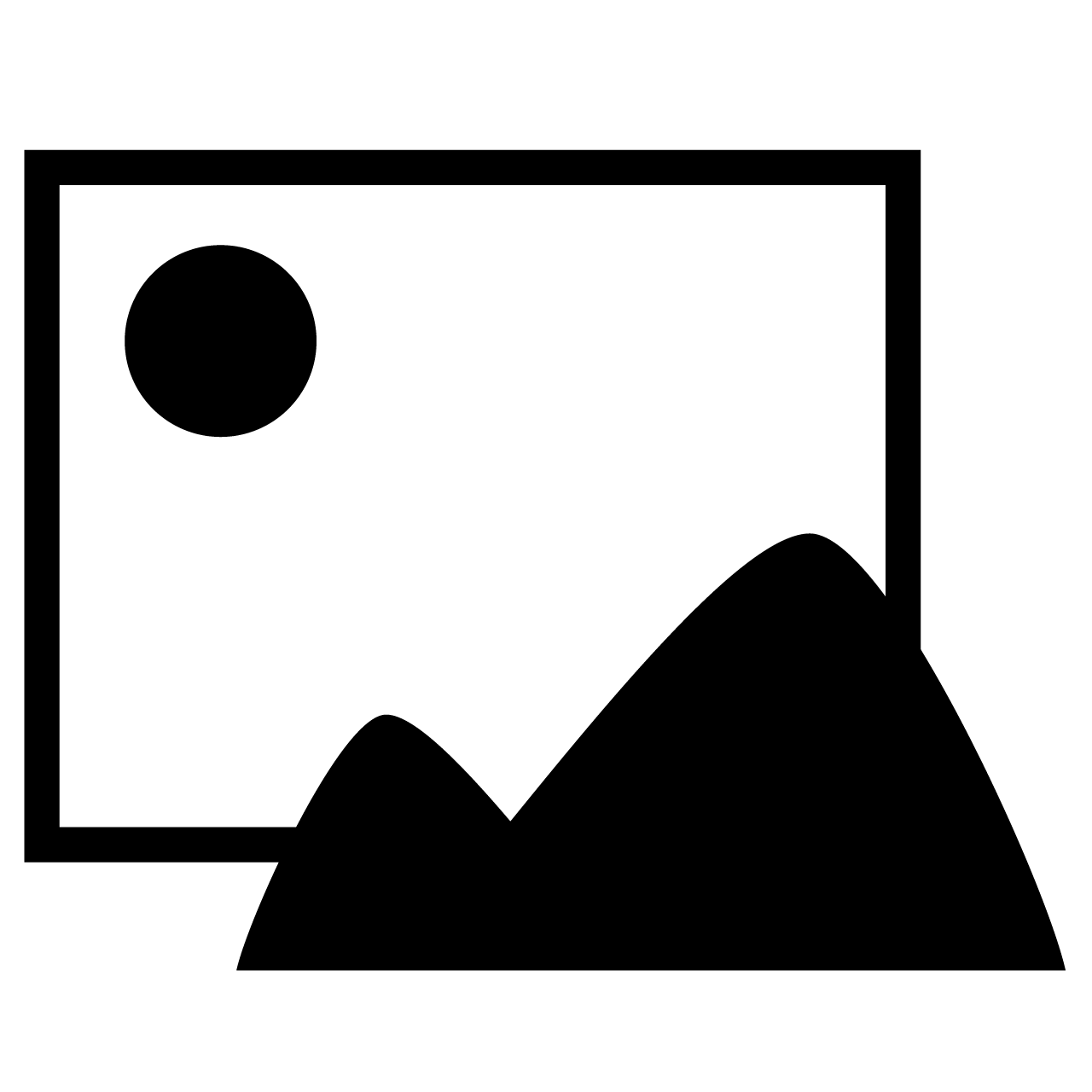
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More