
A rudimentary timer (low granularity), that doesn't lock IGOR up.
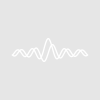
andyfaff
//a background task that you can use to wait for a given timeperiod. This is probably called from //another background task. This is useful if you don't want to lock IGOR up. Function startwait(timeout) variable timeout variable/g root:endtime NVAR endtime = root:endtime endtime = datetime+timeout CtrlNamedBackground waiter,period=60,proc=waittask CtrlNamedBackground waiter, start End Function stopwait() ctrlnamedbackground waiter,stop=1 killvariables root:endtime ENd Function waitStatus() ctrlnamedbackground waiter,status return numberbykey("RUN",S_info) End Function waittask(s) STRUCT WMBackgroundStruct &s NVAR endtime = root:endtime if(datetime > endtime) stopwait() return 1 endif return 0 End
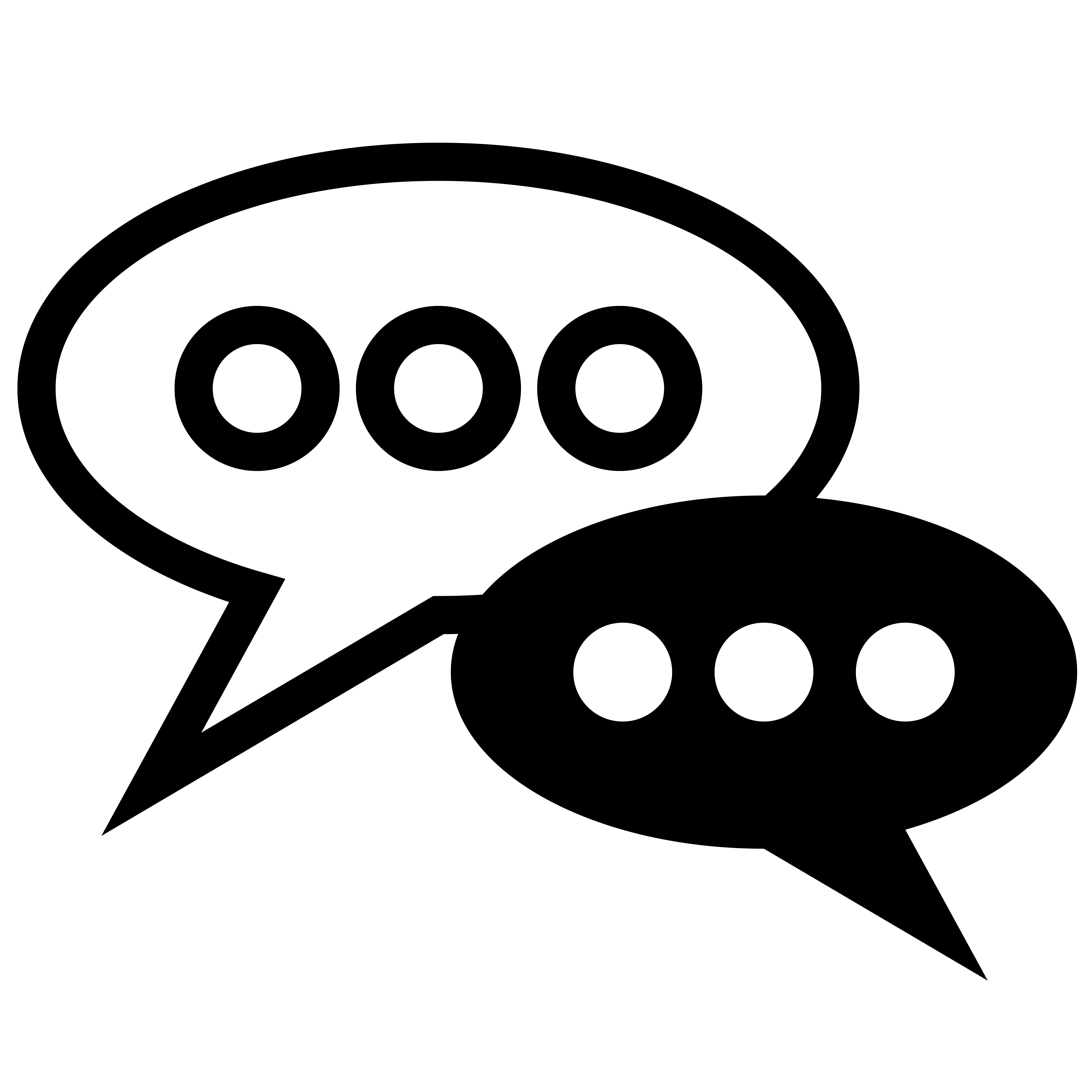
Forum
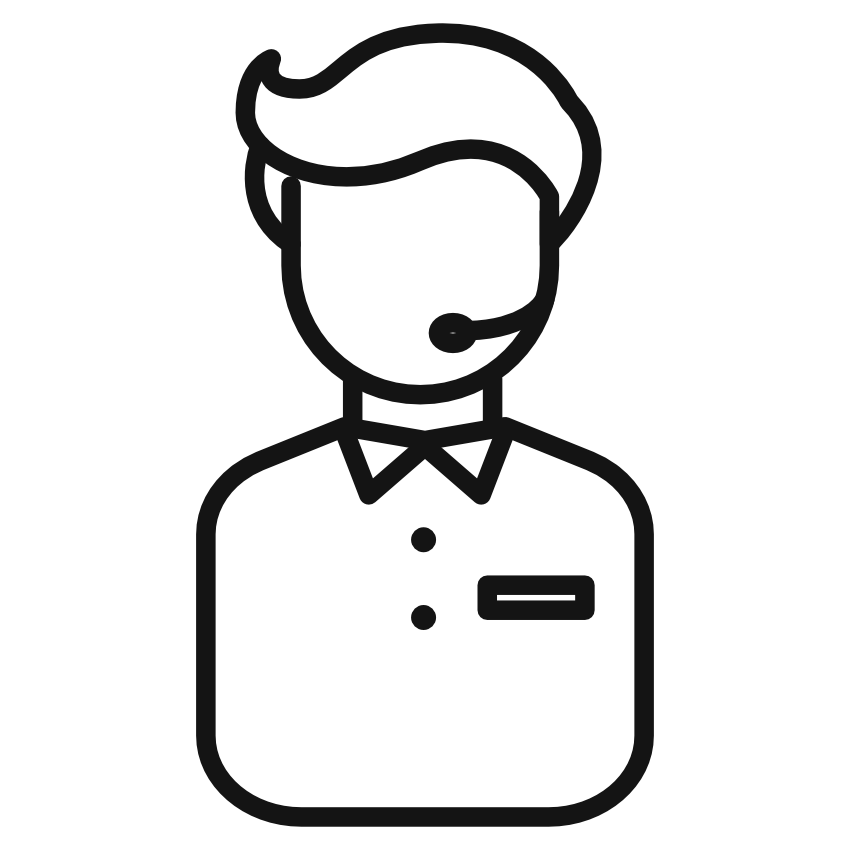
Support
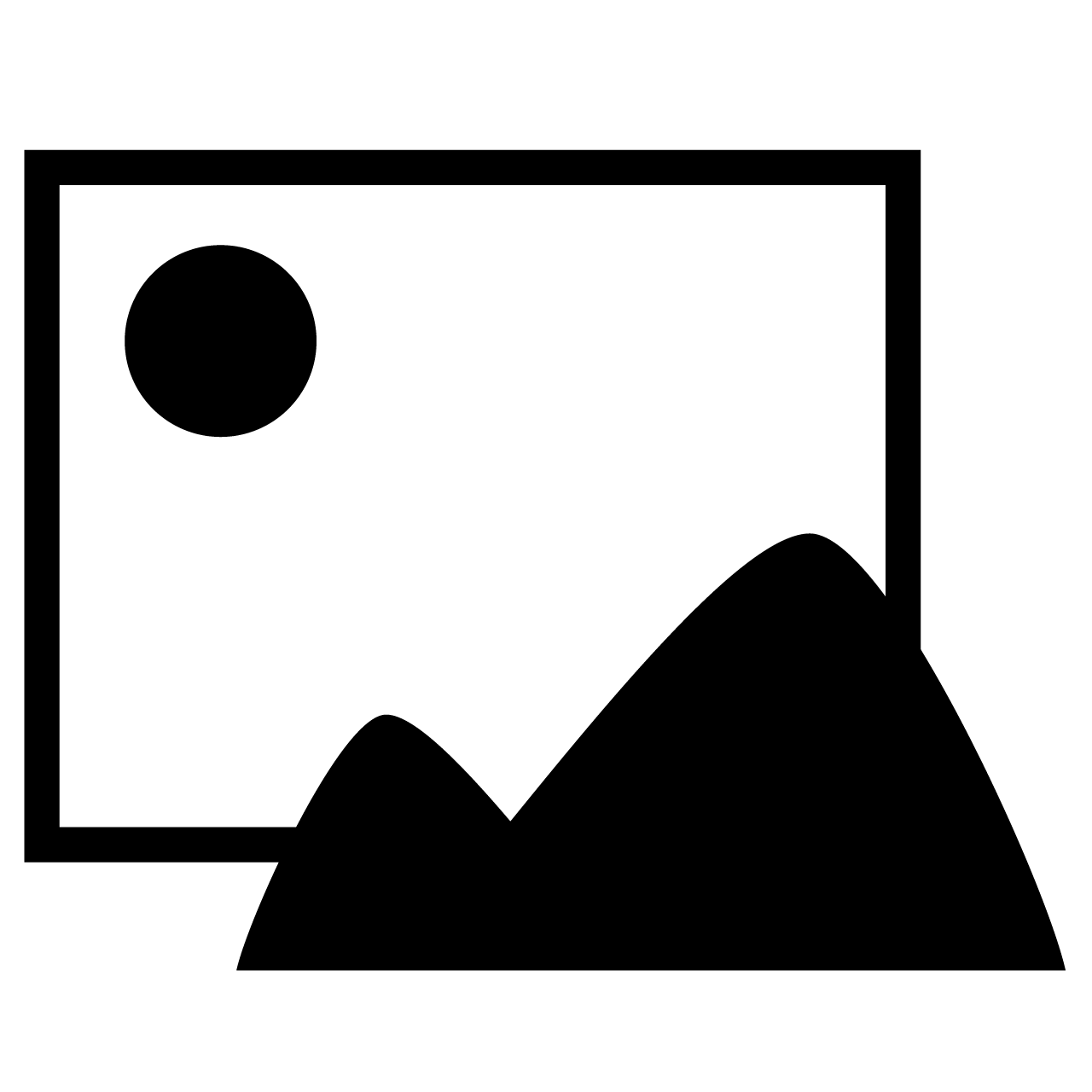
Gallery
Igor Pro 9
Learn More
Igor XOP Toolkit
Learn More
Igor NIDAQ Tools MX
Learn More
May 30, 2010 at 05:13 am - Permalink