
Deleting Specific Points in a Wave
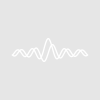
j s s
Hello All,
I am trying to delete specific unwanted points. I have two waves. I need to delete the "topwave" points when both topwave and botwave values !=2 or when it is not a NaN.
I know there is a deletepoints function but it seems to delete a range of points you select instead of checking for a condition and deleting them that way. I was going to replace the "topwave" points with 0, but I am histogramming the wave later and this results in a large bin around 0.
Thanks in advance!
Function IsolateTop(TopWave, BotWave) Wave TopWave,BotWave Variable i, N = numpnts(Topwave) Duplicate Topwave, IsolatedTop for (i=0; i<N; i+=1) if ((NumType(TopWave[i]) !=2) && (NumType(BotWave[i]) != 2)) deletepoints 0, N, IsolatedTop endif endfor end // !=2 numtyp is not a NaN aka a real number // If numtype of isolated top and bottom both have numbers, "deletes" the top where both top and bottom overlap
Hi,
Couple of issues with you approach is delete is slow and more problematic is that in each loop that deletes a point and changes the length of the wave. So when you don't actually test all the points and you since it shifts and you could get an out of range error towards the end of wave as the number of points is reduced. One way around this potential issue that I have used in the past is to work backwards from the end of the wave to point 0. That way you you won't get an out of range error.
Another alternative is to use extract.
Extract source, dest, Topwave!=2 && NumType(BotWave)!=2
Then kill the source wave.
Andy
November 7, 2018 at 07:57 am - Permalink
In reply to Hi, Couple of issues with… by hegedus
This function extracts the points and places them all together in a separate wave, I need to maintain them in the same index order. I haven't figured out how to kill the source wave. Does that delete the extracted points?
November 7, 2018 at 09:06 am - Permalink
Hi,
Give this a try.
Extract TopWave, IsolatedTop, Topwave!=2 && NumType(BotWave)!=2
This will create a new wave IsolatedTop with the points removed and in the same order as in top wave.
Andy
November 7, 2018 at 09:17 am - Permalink
In reply to Hi, Give this a try. … by hegedus
That's what I tried and the new wave doesn't match the index of the source wave.
November 7, 2018 at 09:32 am - Permalink
In reply to Hi, Give this a try. … by hegedus
I hope I'm being clear about what I need.
I essentially want to delete the topwave points when both top and bottom waves have real numbers. So referring to the image I attached, the isolatedtop wave should have blanks, and then have numbers after the 3rd index.
November 7, 2018 at 09:35 am - Permalink
Deleting a point is not the same as setting the value of the point to blank/NaN.
Which do you want?
November 7, 2018 at 10:25 am - Permalink
In reply to Deleting a point is not the… by JimProuty
Sorry for not being clear. I want to set the value to a blank/NaN.
November 7, 2018 at 10:33 am - Permalink
In reply to Deleting a point is not the… by JimProuty
When I try the following code, it assigns all of my other values as 0.
November 7, 2018 at 10:49 am - Permalink
How about:
topwave = Numtype(BotWave)==2? Topwave : NaN
If topwave is a NaN then it stays a NaN
if Botwave is a NaN then the topwave remains the same whether it is a real value or a NaN
if Botwave is a real number then it changes topWave at that point to NaN
The result is a topwave with real values and NaNs.
If you want to subsequently remove the NaNs from topwave you can do a wavetransform zapnans.
Andy
November 7, 2018 at 11:00 am - Permalink
In reply to When I try the following… by j s s
That is because IsolatedTop starts out as all 0s and is assigned nothing else inside your for loop when the conditions do not match. Why did you use Make instead of Duplicate as before?
November 8, 2018 at 06:42 pm - Permalink