
Integer division, convertation from double to integer, loading list of waves, point corresponding to maximum, fixing parameters when fitting
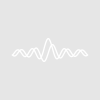
Hi all,
I'm learning Igor Pro programming. I have some questions. Could you please help me with solving them?
1) How to implement integer division, i.e. 1/3 = 0?
2) How to convert double variable to integer and vice versa?
3) How to load list of waves with preserving original names of files? For example, one folder contains txt files 1.txt, 2.txt, 3.txt, 4.txt, 5.txt. I need to load them into Igor as waves and give them the same names, i.e. 1.txt, 2.txt, 3.txt, 4.txt, 5.txt, instead of wave0, wave1, wave2, wave3, wave4.
4) How to change wave scaling for each wave, i.e. apply the same wave scaling to each wave from list? And how to set "Start and Right" scaling mode?
5) How to plot wave (y-value) vs calculated (x-value) implying that calculated is new calculated, i.e. after changing wave scaling?
6) How to save graph to specific folder as .pdf or .png file?
7) How to fix some parameters when fitting?
8) How to find point and corresponding x-value which correspond to maximum y-value?
I will be very appreciate for you help.
That’s a lot of questions that could have been split into multiple posts to help other readers find answers to the same question.
Let me take a first cut:
1) How to implement integer division, i.e. 1/3 = 0?
Waves can have 8, 16 and 32 bit integer format, so wave arithmetic will do what you want. Variables are double precision. Maybe the round, mod, floor and related functions will help here?
2) How to convert double variable to integer and vice versa?
As above. Note also that structures can have integer fields.
3) How to load list of waves with preserving original names of files? For example, one folder contains txt files 1.txt, 2.txt, 3.txt, 4.txt, 5.txt. I need to load them into Igor as waves and give them the same names, i.e. 1.txt, 2.txt, 3.txt, 4.txt, 5.txt, instead of wave0, wave1, wave2, wave3, wave4.
Here you’ll likely need to write a little code. Use open /D/R/F=fileFilters/MULT=1 refnum to get a list of files, construct a list or textwave to hold the desired wavenames, then loop using an appropriate combination of flags for LoadWave together with the destination name to do the file loading. Code that does something like this is available on request, and there’s probably some example code in the manual or on this site somewhere.
4) How to change wave scaling for each wave, i.e. apply the same wave scaling to each wave from list? And how to set "Start and Right" scaling mode?
displayHelpTopic "setscale"
A common task is to load a two column text wave and take the scaling values for the second column from the first and last points of the first column. Ask if you want to see some template code.
5) How to plot wave (y-value) vs calculated (x-value) implying that calculated is new calculated, i.e. after changing wave scaling?
If you plot wave vs calculated, changing scaling will update the plot. I have a utility function that converts waves plotted as y vs x to scaled waves and replaces them on the plot with waveform traces, if that’s of interest.
6) How to save graph to specific folder as .pdf or .png file?
If you’re exporting graphics, say, by using File – Save Graphics, notice that commandline records the appropriate Igor operation.
displayHelpTopic "SavePict"
You should also read about paths (NewPath, ParseFilePath, and more).
7) How to fix some parameters when fitting?
Check the help for this. Basically you create a coefficient wave and add a flag to tell CurveFit or FuncFit which parameters to hold. Try it with the GUI first and take a look at the command history.
8) How to find point and corresponding x-value which correspond to maximum y-value?
displayHelpTopic "wavestats"
-- edited to format code
April 16, 2019 at 05:30 am - Permalink
In reply to That’s a lot of questions… by tony
Thanks a lot for your answer! It would be great to see how to solve the problem #3 (loading list of waves with preserving original names of files). I tried to compose some code, but it doesn't do what I would like.
April 16, 2019 at 07:39 am - Permalink
1) How to implement integer division, i.e. 1/3 = 0?
The above requires Igor 7 or later. With earlier versions you can use the methods already suggested by others.
Regarding #3, LoadWave allows you to specify a name, though it can sometimes be complicated. See the documentation for the /A, /B, /N, and /W flags, all which can control how loaded waves are named. You could also let LoadWave use whatever name it wants and then use the S_waveNames output variable to get the name of the loaded wave and call Rename to give it a new name.
But be careful--you said you wanted the names to be something like 1.txt, which requires use of liberal names. Programming with liberal names can be tricky. For more information, execute the following command on Igor's command line:
DisplayHelpTopic "Programming with Liberal Names"
You also might find this example useful:
DisplayHelpTopic "Loading All of the Files in a Folder"
With that example, there's one thing to keep in mind--the order of files returned by IndexedFile is undefined (Igor takes whatever the OS gives, and that varies by OS and file system). If the order is important, you'll want to use IndexedFile($pathName, -1, ".dat") to get a list of all files in a directory, and then call SortList on that list to sort it how you want it sorted.
Also, if you haven't already done so, I recommend that you go through the guided tour (Help->Getting Started). It might take you an hour or two, but you'll learn a lot about some of Igor's basic functionality that's easy to miss if you just dive in.
April 16, 2019 at 08:21 am - Permalink
In reply to 1) How to implement integer… by aclight
Thanks a lot! I will try it.
April 16, 2019 at 08:47 am - Permalink
In reply to tony wrote: That’s a lot… by Physicist92
Here's an example of some code for loading multiple files. The code expects the text files to have two columns, and sets scaling of the second wave based on the X values in the first column. Wavenames are based on filename after conversion to non-liberal format.
April 16, 2019 at 09:05 am - Permalink
In reply to Here's an example of some… by tony
Thank you very much!
April 16, 2019 at 10:57 am - Permalink
This might get you started:
https://www.wavemetrics.com/code-snippet/load-all-files-folder-assumes-…
April 17, 2019 at 06:55 pm - Permalink